5 Easy Tips for Referencing Sheets in Excel VBA

Understanding the Basics of Excel VBA

Before diving into the intricacies of referencing sheets in Excel VBA, it’s crucial to have a basic understanding of what VBA (Visual Basic for Applications) entails. VBA is an event-driven programming language developed by Microsoft, primarily for the customization and automation of Office applications like Excel. Here’s how you can get started:
- Open the VBA Editor: Press Alt + F11 to open the VBA Editor. This is where you'll write your macros and functions.
- Insert a Module: From the VBA Editor, click Insert > Module to add a module to your VBA project.
- Basic VBA Syntax: Begin your VBA code with the keyword
Sub
followed by the name of your macro.
📝 Note: Make sure your security settings allow macros to run. You can change this from the Excel Options menu.
1. Using the Sheet’s CodeName
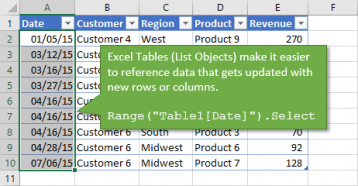
One of the easiest and most reliable ways to reference a sheet in VBA is by using its CodeName, which remains constant even if the sheet’s name changes.
- Find the Sheet's CodeName: In the Project Explorer of the VBA Editor, each sheet has two names - the one you see on the tab and the code name (e.g., Sheet1).
- Refer to the Sheet: Use the CodeName directly in your VBA code. Here's an example:
Sheet1.Range("A1").Value = "Hello"
2. Using the Sheet’s Name

Another common method for referencing sheets is using the sheet name. However, this approach can be less stable if the sheet name changes.
- Reference by Sheet Name:
Worksheets("Sheet1").Range("A1").Value = "World"
- Dealing with Spaces: If your sheet name includes spaces, you need to use single quotes:
Worksheets("'Sales Data'").Range("A1").Value = "Sales"
3. Referencing Multiple Sheets

When working with multiple sheets, using a loop or array can simplify referencing:
- Looping Through Sheets:
Dim ws As Worksheet For Each ws In ThisWorkbook.Worksheets ws.Range("A1").Value = "Test" Next ws
- Using an Array:
Dim sheetNames(1 To 3) As String sheetNames(1) = "Sheet1" sheetNames(2) = "Sheet2" sheetNames(3) = "Sheet3" Dim i As Integer For i = LBound(sheetNames) To UBound(sheetNames) Worksheets(sheetNames(i)).Range("A1").Value = "Value" Next i
⚠️ Note: When looping through sheets, consider whether you want to include chartsheets, which might require different handling.
4. Dynamic Sheet Referencing

To make your VBA code more adaptable, use variables to reference sheets dynamically:
- Using Variables:
Dim sheetName As String sheetName = "Sheet1" Worksheets(sheetName).Range("A1").Value = "Hello Dynamic!"
- Checking for Sheet Existence:
If WorksheetExists(sheetName) Then Worksheets(sheetName).Activate Else MsgBox "Sheet not found!" End If Function WorksheetExists(shtName As String) As Boolean Dim ws As Worksheet WorksheetExists = False For Each ws In ThisWorkbook.Worksheets If ws.Name = shtName Then WorksheetExists = True Exit Function End If Next ws End Function
5. Using Index Numbers for Sheet References

Sheets can also be referenced by their index number, which is less reliable due to potential changes in sheet order:
- Index Number:
Worksheets(1).Range("A1").Value = "First Sheet"
Now that we've gone through various methods to reference sheets in Excel VBA, it's clear that there's no one-size-fits-all approach. Each method has its advantages and use cases:
- CodeName is best for sheets whose name might change but whose code names remain constant.
- Sheet Name is useful when you need to reference specific sheets, especially if you're working with well-named tabs.
- Multiple Sheets - Looping or using arrays can be very efficient when dealing with multiple sheets or when the number of sheets might change.
- Dynamic References offer flexibility in dynamically changing environments or when the sheet's name might be input by the user or fetched from a cell.
- Index Numbers are useful for quick referencing or when the order of sheets is guaranteed, although this approach should be used sparingly due to its potential instability.
In conclusion, selecting the right method for referencing sheets in VBA involves understanding your project's requirements, the stability of your workbook's structure, and the potential for future changes. By using these techniques, you can write robust, flexible, and maintainable VBA code, ensuring that your macros perform reliably no matter how your Excel files evolve over time.
What’s the difference between a sheet’s name and CodeName in VBA?

+
The name is what you see and can edit in the Excel interface, while the CodeName is a constant identifier used in VBA that doesn’t change even if the tab name does.
Can I reference a sheet by its tab color?

+
Yes, but this method is not commonly used as it requires additional functions to retrieve sheets by color, which can be less reliable due to potential Excel UI changes or user modifications to colors.
Why use a loop to reference multiple sheets?

+
A loop allows your code to be dynamic and handle changes in the number of sheets or their order, reducing the need to manually update your code.
How do I reference sheets from another workbook?

+
You can use the Workbooks collection to reference external sheets. For example, Workbooks(“OtherWorkbook.xlsx”).Sheets(“Sheet1”).Range(“A1”).Value = “Value”