Read Excel Column Index Effortlessly in Python

Reading Excel column indices can be a daunting task, especially when dealing with large datasets or when you need to quickly reference specific columns. However, with Python's robust libraries like openpyxl and pandas, this task becomes much more manageable. This blog post will explore various methods to read Excel column indices effortlessly using Python, providing both beginners and seasoned programmers with practical examples and insights.
Why Read Excel Column Indices?

Before diving into the methods, understanding why you might need to read Excel column indices is crucial:
- Data Analysis: Quickly identifying columns for data extraction or manipulation.
- Automation: Streamlining workflows by automating data processing tasks.
- Reporting: Generating reports that require specific columns from large datasets.
Getting Started with openpyxl

The openpyxl library is specifically designed for reading and writing Excel 2010 xlsx/xlsm/xltx/xltm files. Here’s how you can use it to read column indices:
from openpyxl import load_workbook
# Load the workbook
wb = load_workbook('example.xlsx')
sheet = wb.active
# Iterate through columns
for col in sheet.iter_cols(min_row=1, max_row=1, values_only=True):
for cell in col:
print(cell)
This code snippet will print the value of each cell in the first row, which typically contains the header names corresponding to the columns.
Working with Pandas
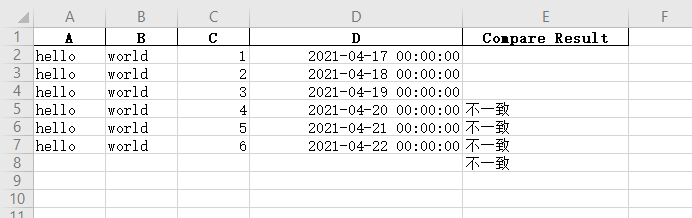
Pandas, known for its data manipulation capabilities, also provides tools for handling Excel files:
import pandas as pd
# Read Excel file
df = pd.read_excel('example.xlsx')
# Display column names
print(df.columns)
Pandas reads the Excel file into a DataFrame, which can be further manipulated. The columns
attribute of the DataFrame gives us a list of column names, effectively allowing us to reference columns by index or name.
Mapping Column Indices to Names

When working with complex spreadsheets where you need to frequently reference columns by their numeric index but prefer to deal with their names, mapping is useful:
import pandas as pd
df = pd.read_excel('example.xlsx')
# Create a dictionary mapping indices to column names
column_map = {index: name for index, name in enumerate(df.columns)}
print(column_map)
Automating Column Selection

Automating column selection can save time, especially when you’re dealing with multiple files or updating data dynamically:
import pandas as pd
# Load the Excel file
df = pd.read_excel('example.xlsx')
# Assume you want to select columns named 'ID', 'Name', and 'Salary'
columns_to_select = ['ID', 'Name', 'Salary']
# Select the columns
selected_df = df[columns_to_select]
# Print the DataFrame to verify selection
print(selected_df)
Notes on Performance

💡 Note: When dealing with large files, consider reading only the necessary columns to improve performance. Use the usecols
parameter in pd.read_excel()
.
Handling Multiple Sheets

In an Excel workbook with multiple sheets, you might need to dynamically identify which sheet contains the required data:
from openpyxl import load_workbook
wb = load_workbook('example.xlsx')
# Iterate through sheet names
for sheet_name in wb.sheetnames:
sheet = wb[sheet_name]
print(f"Columns in sheet '{sheet_name}':")
for col in sheet.iter_cols(min_row=1, max_row=1, values_only=True):
print(f" - {col[0]}")
Conclusion

Reading and manipulating Excel column indices in Python has become a streamlined process thanks to libraries like openpyxl and pandas. By automating these tasks, you can significantly increase productivity, ensuring that data analysis, reporting, and automation tasks are performed with greater efficiency and accuracy. Whether you’re a data scientist, a financial analyst, or an IT professional, mastering these techniques allows for more dynamic and less error-prone data handling. As datasets grow and become more complex, the ability to effortlessly navigate Excel files using Python remains a valuable skill.
Can openpyxl handle older Excel file formats?

+
openpyxl primarily focuses on the newer Excel file formats (.xlsx, .xlsm). For older formats like .xls, you might need to use libraries like xlrd.
How can I speed up reading large Excel files?

+
Use pandas with parameters like usecols
to read only the necessary columns, or consider converting Excel files to CSV before reading with pandas for a performance boost.
Is there a way to handle formulas in Excel when reading?

+
Yes, openpyxl can read the formulas as they are written in the cells, although the values of these formulas are not calculated within Python; you’ll get the formula string itself.