Reading Excel Sheets in VB.NET: Simplified Guide
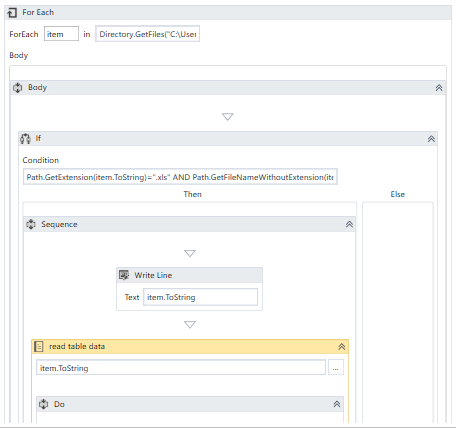
Working with Excel files in VB.NET can initially seem daunting, especially for developers who are new to handling spreadsheets programmatically. However, with the right tools and a bit of guidance, manipulating Excel files can become a streamlined and efficient part of your software development arsenal. This blog post will delve into a detailed guide on how to read Excel sheets using VB.NET, focusing on essential techniques, common use cases, and some advanced tips for enhancing your applications with Excel integration.
The Basics of VB.NET and Excel Interoperability

When you decide to integrate Excel functionality into a VB.NET application, you typically interact with the Excel Object Model through COM (Component Object Model) interoperability. Here's a basic overview of how you can start:
- Microsoft.Office.Interop.Excel - This assembly provides access to Excel objects.
- Import the Excel namespace: Add this to your VB.NET project:
Imports Microsoft.Office.Interop.Excel
- Create an Excel application instance: This can be done with the following code snippet:
Dim xlApp As New Application Dim xlWorkBook As Workbook = xlApp.Workbooks.Open("path_to_your_excel_file")
💡 Note: Make sure your target system has Excel installed since this method relies on COM interoperability which requires the actual Excel application.
Reading Data from Excel Sheets

Once you have opened an Excel workbook, the next step is to read data from the sheets. Here's how you can do it:
- Select a Worksheet: Use the Sheets collection to access specific sheets by name or index.
Dim xlWorksheet As Worksheet = xlWorkBook.Sheets("Sheet1")
- Reading Cell Values: You can read cell values directly or in a loop for multiple cells:
' Read a single cell Dim cellValue As String = xlWorksheet.Cells(1, 1).Value.ToString() ' Loop through rows and columns to read multiple cells For row As Integer = 1 To 10 For col As Integer = 1 To 5 Dim value As String = xlWorksheet.Cells(row, col).Value.ToString() Console.WriteLine("Row " & row & ", Col " & col & ": " & value) Next Next
- Handling Different Data Types: Excel stores data in a Variant type, so you'll need to check and cast the values accordingly.
Dim variantValue = xlWorksheet.Cells(1, 1).Value If IsNumeric(variantValue) Then Dim numericValue As Double = CDbl(variantValue) ElseIf IsDate(variantValue) Then Dim dateValue As Date = CDate(variantValue) Else Dim stringValue As String = variantValue.ToString() End If
Advanced Techniques for Efficient Excel Sheet Reading

To make your VB.NET application more efficient when dealing with Excel, consider these advanced techniques:
- Use Excel Ranges: Instead of accessing cells one by one, you can work with ranges which are more efficient for bulk operations:
Dim xlRange As Range = xlWorksheet.Range("A1:E10") Dim values As Object(,) = CType(xlRange.Value, Object(,)) For i As Integer = 1 To values.GetLength(0) For j As Integer = 1 To values.GetLength(1) Console.WriteLine("Row " & i & ", Col " & j & ": " & values(i, j).ToString()) Next Next
- Lazy Loading: Load only the necessary parts of the Excel file to improve performance:
' Open the workbook with read-only mode to conserve resources Dim xlWorkBook As Workbook = xlApp.Workbooks.Open("path_to_your_excel_file", ReadOnly:=True)
- Use ADO.NET to Connect to Excel: For more complex data manipulations, you might consider using ADO.NET to query Excel like a database:
Dim conn As New OleDbConnection() conn.ConnectionString = "Provider=Microsoft.ACE.OLEDB.12.0;Data Source=" & excelFilePath & ";Extended Properties='Excel 12.0 Xml;HDR=YES;'" conn.Open() Dim cmd As New OleDbCommand("SELECT * FROM [Sheet1$]", conn) Dim reader As OleDbDataReader = cmd.ExecuteReader() While reader.Read() Console.WriteLine(reader("Column1").ToString()) End While reader.Close() conn.Close()
To wrap up, reading Excel sheets in VB.NET can be simplified through the use of COM interoperability or even more so with advanced methods like using ADO.NET. Each technique has its own merits, depending on the specifics of your application's needs. Remember to choose the method that aligns with your efficiency requirements and ease of maintenance. Moving forward with Excel integration in your VB.NET applications, you'll be well-prepared to handle complex data manipulation tasks with confidence. Whether it's importing data into a database, processing it for further use, or just presenting it in a custom format, these skills will empower your applications with dynamic Excel capabilities.
What are the prerequisites for reading Excel files in VB.NET?

+
You need to have Microsoft Excel installed on the target system because VB.NET interacts with Excel through COM interop. Additionally, ensure you reference the ‘Microsoft.Office.Interop.Excel’ assembly in your VB.NET project.
Can I use VB.NET to read Excel files without Excel being installed?

+
Yes, you can use libraries like EPPlus or ExcelDataReader, which allow you to read Excel files (.xlsx, .xls) without requiring Excel to be installed.
How do I deal with large Excel files?

+
For large files, using Excel Ranges for bulk operations or lazy loading techniques can improve performance. Alternatively, consider using ADO.NET to connect to Excel as if it were a database, which provides better handling of large datasets.