Mastering Multiple Excel Sheet Import in Python

Managing large datasets often involves working with multiple Excel files, each containing different sheets. Importing these sheets efficiently into Python can streamline your data analysis process, making tasks like data cleaning, transformation, and analysis much easier. This guide will walk you through the steps to import multiple Excel sheets into Python using pandas, a powerful data manipulation library.
Why Use Python for Excel Data?

Python is not just versatile for web development, AI, and data science; it’s also an excellent tool for handling spreadsheets. Here are a few reasons why Python is preferred for Excel data import:
- Speed and Efficiency: Python’s libraries allow for quick data processing.
- Flexibility: You can easily manipulate data structures and automate repetitive tasks.
- Integration: Python can be integrated with other tools and databases for further analysis.
Prerequisites

Before diving in, ensure you have the following:
- Python (version 3.6 or above).
pandas
library installed (pip install pandas
).openpyxl
library for reading Excel files (pip install openpyxl
).
Importing Single Excel Sheet

Before handling multiple sheets, let’s look at how to import a single Excel sheet:
import pandas as pd
df = pd.read_excel(‘filename.xlsx’, sheet_name=‘Sheet1’)
print(df.head()) # View the first 5 rows
🔎 Note: Ensure your Excel file is in the same directory as your script or provide the full path.
Importing Multiple Sheets

Pandas provides several ways to import multiple sheets from a single or multiple Excel files:
Using sheet_name
parameter

Specify multiple sheets by name or index:
import pandas as pd
dfs = pd.read_excel(‘multiplesheets.xlsx’, sheet_name=[‘Sheet1’, ‘Sheet2’])
dfs = pd.read_excel(‘multiplesheets.xlsx’, sheet_name=[0, 1])
Importing All Sheets from a File

To load all sheets:
all_sheets = pd.read_excel(‘multiplesheets.xlsx’, sheet_name=None)
for sheet_name, df in all_sheets.items(): print(f’Sheet: {sheet_name}\n’) print(df.head()) print(‘\n’)
✨ Note: When using sheet_name=None
, pandas returns a dictionary where keys are sheet names and values are DataFrames.
Importing Multiple Files
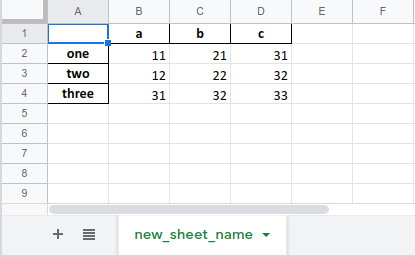
Here’s how you can import sheets from multiple Excel files in a directory:
import os import pandas as pd
directory = ‘path_to_directory’
all_dfs = {}
for filename in os.listdir(directory): if filename.endswith(‘.xlsx’): filepath = os.path.join(directory, filename) sheets = pd.read_excel(filepath, sheet_name=None)
for sheet_name, df in sheets.items(): full_key = f"{filename.split('.')[0]}_{sheet_name}" all_dfs[full_key] = df
Merging Data

Once you’ve imported the data, merging can provide a consolidated view:
- Concatenate Rows: If data from different sheets needs to be stacked vertically:
combined_df = pd.concat(all_dfs.values(), ignore_index=True)
df1 = all_dfs[‘file1_sheet1’] df2 = all_dfs[‘file1_sheet2’] merged = pd.merge(df1, df2, on=‘key’)
Advanced Techniques

Data Cleaning

Often, imported data requires cleaning:
- Handling Missing Values: Use
fillna
ordropna
methods. - Removing Duplicates:
drop_duplicates()
can eliminate repeated data. - Data Type Conversion: Ensure columns have the correct data types.
Automating Import Process

If you’re dealing with recurring files:
from datetime import datetime
def dailyimport(): date = datetime.now().strftime(‘%Y-%m-%d’) filename = f’report{date}.xlsx’ sheets = pd.read_excel(os.path.join(directory, filename), sheet_name=None) process_data(sheets) # Call a function to handle your data
In this overview, we’ve explored how to efficiently import multiple Excel sheets into Python, offering flexibility in handling diverse data scenarios. Whether you’re dealing with sheets within a single file or from multiple files, the techniques covered here facilitate a seamless transition from Excel to Python for data analysis, cleaning, and further processing. Python’s integration with tools like pandas not only makes the process efficient but also enhances your ability to automate and manage large datasets effectively.
What is the difference between sheet_name
and sheet_name=None
when importing Excel files?

+
Using sheet_name
with a value like ‘Sheet1’ imports that specific sheet. With sheet_name=None
, all sheets are imported into a dictionary where each sheet name is the key, and its DataFrame is the value.
How can I automate the process of importing Excel data daily?

+
Create a script that imports data based on the current date, then automate it using task schedulers like cron jobs or Windows Task Scheduler to run the script at specified intervals.
Can I import Excel files if they are password-protected?

+
Unfortunately, pandas does not support importing password-protected Excel files out-of-the-box. You would need third-party libraries or to manually unlock the files before importing.