5 Easy Ways to Import Excel Sheets into R
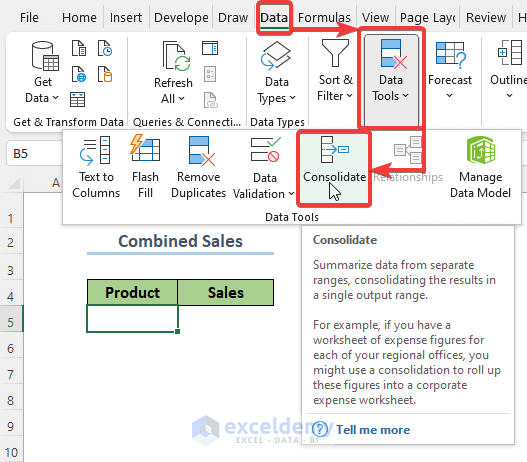
Let's explore five straightforward methods to import Excel sheets into R, a popular programming language for statistical computing and graphics. Importing Excel data into R can streamline your data analysis workflow, allowing you to leverage R's extensive libraries and tools for data manipulation and visualization.
Method 1: Using the readxl Package

The readxl package in R provides functions to read Excel files directly without needing additional software like Java or Python. Here are the steps:
- Install and load the
readxl
package:
install.packages("readxl")
library(readxl)
data <- read_excel("path/to/your/file.xlsx")
data_sheet2 <- read_excel("path/to/your/file.xlsx", sheet = 2)
🔔 Note: The readxl
package supports .xlsx files by default, but can also handle older .xls files with some limitations.
Method 2: Using the xlsx Package

The xlsx package allows you to read and write Excel 2007 (.xlsx) files. Here’s how to use it:
- Install and load the
xlsx
package:
install.packages("xlsx")
library(xlsx)
data <- read.xlsx("path/to/your/file.xlsx", sheetIndex = 1)
Sys.setenv(JAVA_HOME = "path/to/java")
Method 3: Using the openxlsx Package

The openxlsx package provides functionalities for reading and writing Excel files without requiring Java or external dependencies:
- Install and load the
openxlsx
package:
install.packages("openxlsx")
library(openxlsx)
data <- read.xlsx("path/to/your/file.xlsx")
Here's a small comparison table for the packages discussed:
Package | Dependency | File Types |
---|---|---|
readxl | None | .xlsx, .xls |
xlsx | Java | .xlsx |
openxlsx | None | .xlsx |

Method 4: Using Base R Functions

If you prefer to stick with base R, you can convert Excel files to CSV or use read.table()
with specific settings to read delimited files:
- First, save your Excel sheet as a CSV or tab-delimited file.
- Then, use:
data <- read.csv("path/to/your/file.csv")
data <- read.delim("path/to/your/file.tsv")
Method 5: Using the RODBC Package for Larger Files

The RODBC package lets you connect to various database systems including Excel, which is useful for larger datasets:
- Install and load the
RODBC
package:
install.packages("RODBC")
library(RODBC)
conn <- odbcConnectExcel("path/to/your/file.xlsx")
data <- sqlFetch(conn, "Sheet1")
odbcClose(conn)
Each method has its advantages depending on your workflow needs: - readxl: Simplest to use, no external dependencies. - xlsx: Requires Java but has more comprehensive read/write capabilities. - openxlsx: No external dependencies, easy to work with. - Base R: Widely applicable beyond Excel. - RODBC: Best for larger datasets, flexible querying. The choice between these methods can be influenced by file size, complexity, software dependencies, and personal workflow preferences. You might find one method more suited to your specific situation, or you might alternate between them based on the context of your data analysis tasks.
What is the fastest way to import Excel data into R?
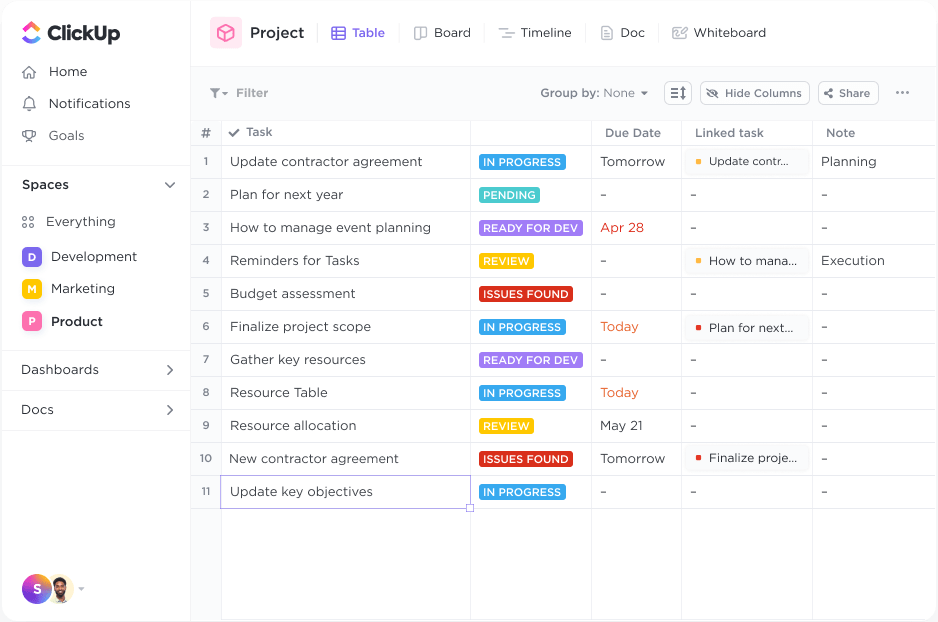
+
For small to medium-sized files, the readxl
package offers a balance of speed and simplicity, making it one of the fastest methods to import Excel data into R.
Can R import other spreadsheet formats besides Excel?

+
Yes, R can handle various formats like CSV, Google Sheets (using packages like googlesheets
), LibreOffice, and more, often by exporting to an intermediary format like CSV or using specialized packages for those formats.
How do I deal with large Excel files in R?

+
For larger files, consider using the RODBC
package which allows for efficient querying of data or batch processing to handle data in chunks, reducing memory usage.