5 Ways to Read Excel Sheets in R Efficiently

The R programming language has become indispensable for data analysis and manipulation, offering a rich ecosystem of libraries that simplify complex tasks, including dealing with Excel spreadsheets. Excel files are widely used for storing data, but reading them efficiently in R requires familiarity with the right tools and techniques. Here are five efficient ways to read Excel sheets in R, ensuring smooth data handling and analysis.
Using readxl Package

The readxl package is one of the simplest and most efficient ways to import Excel data directly into R. Here’s how you can get started:
- Installation: Use the command
install.packages(“readxl”)
to install the package. - Loading the library: After installation, load the library with
library(readxl)
. - Reading a sheet: Use the
read_excel()
function to read the Excel file, specifying the path to the file and the sheet you want to import.
library(readxl)
data <- read_excel(“path/to/your/file.xlsx”, sheet = “Sheet1”)
💡 Note: The readxl
package can handle both .xlsx and .xls file formats, making it versatile for different versions of Excel.
Importing with openxlsx

The openxlsx package is another great alternative for dealing with Excel files, especially when you need to work with styles or conditional formatting. Here’s how to use it:
- Installation: Install the package with
install.packages(“openxlsx”)
. - Loading: Load it into R with
library(openxlsx)
. - Reading: Use the
read.xlsx()
function to read the data:
library(openxlsx)
data <- read.xlsx(“path/to/your/file.xlsx”, sheet = “Sheet1”)
R Programming with xlsx Package

If you are looking for basic reading and writing functionalities, the xlsx package might be your choice. Here’s how you can leverage it:
- Installation: Install the package using
install.packages(“xlsx”)
. - Loading: Load with
library(xlsx)
. - Reading: Use the
read.xlsx()
function:
library(xlsx)
data <- read.xlsx(“path/to/your/file.xlsx”, sheetName = “Sheet1”)
💡 Note: The xlsx
package requires Java to be installed on your system, which can be a consideration for setup.
Using gdata Package

While not as commonly used for this purpose, the gdata package offers functionality for reading Excel files:
- Installation: Use
install.packages(“gdata”)
to install the package. - Loading: Load with
library(gdata)
. - Reading: The
read.xls()
function can be used to read the data:
library(gdata)
data <- read.xls(“path/to/your/file.xlsx”, sheet = “Sheet1”)
Leveraging RODBC for Excel

The RODBC package provides database connectivity, including Excel files, which can be particularly useful when dealing with large datasets:
- Installation: Install with
install.packages(“RODBC”)
. - Loading: Load the package with
library(RODBC)
. - Setting up Connection: Establish a connection to the Excel file using ODBC:
library(RODBC)
conn <- odbcConnectExcel(“path/to/your/file.xlsx”)
data <- sqlFetch(conn, “Sheet1”)
odbcClose(conn)
💡 Note: RODBC requires Excel to be installed on your machine, and there might be some setup complexities due to driver installation.
To wrap up, having an understanding of multiple packages like readxl, openxlsx, xlsx, gdata, and RODBC allows R users to choose the most suitable method for reading Excel files based on specific needs such as speed, file handling capabilities, or integration with other functionalities. Each package has its strengths, making it important to consider the nature of your data and your analysis requirements when selecting one. Here are some key takeaways:
- Flexibility: Different packages offer various levels of support for Excel file formats and features.
- Performance: Speed can vary, with
readxl
being one of the fastest for basic Excel file reading. - Compatibility: Some packages might need additional setup like Java or Excel itself installed on the system.
By using these methods, analysts and data scientists can efficiently incorporate Excel data into their R environment, thereby leveraging R’s powerful data analysis capabilities on Excel’s ubiquitous data storage format.
Which package should I use to read Excel files in R?

+
The choice depends on your needs: - readxl: Ideal for simplicity and speed with basic Excel files. - openxlsx: For more complex Excel file operations, including style preservation. - xlsx: Basic operations with a Java dependency. - gdata: Legacy option for smaller datasets. - RODBC: When dealing with large datasets or requiring database-like interactions.
Can I read both .xls and .xlsx formats?
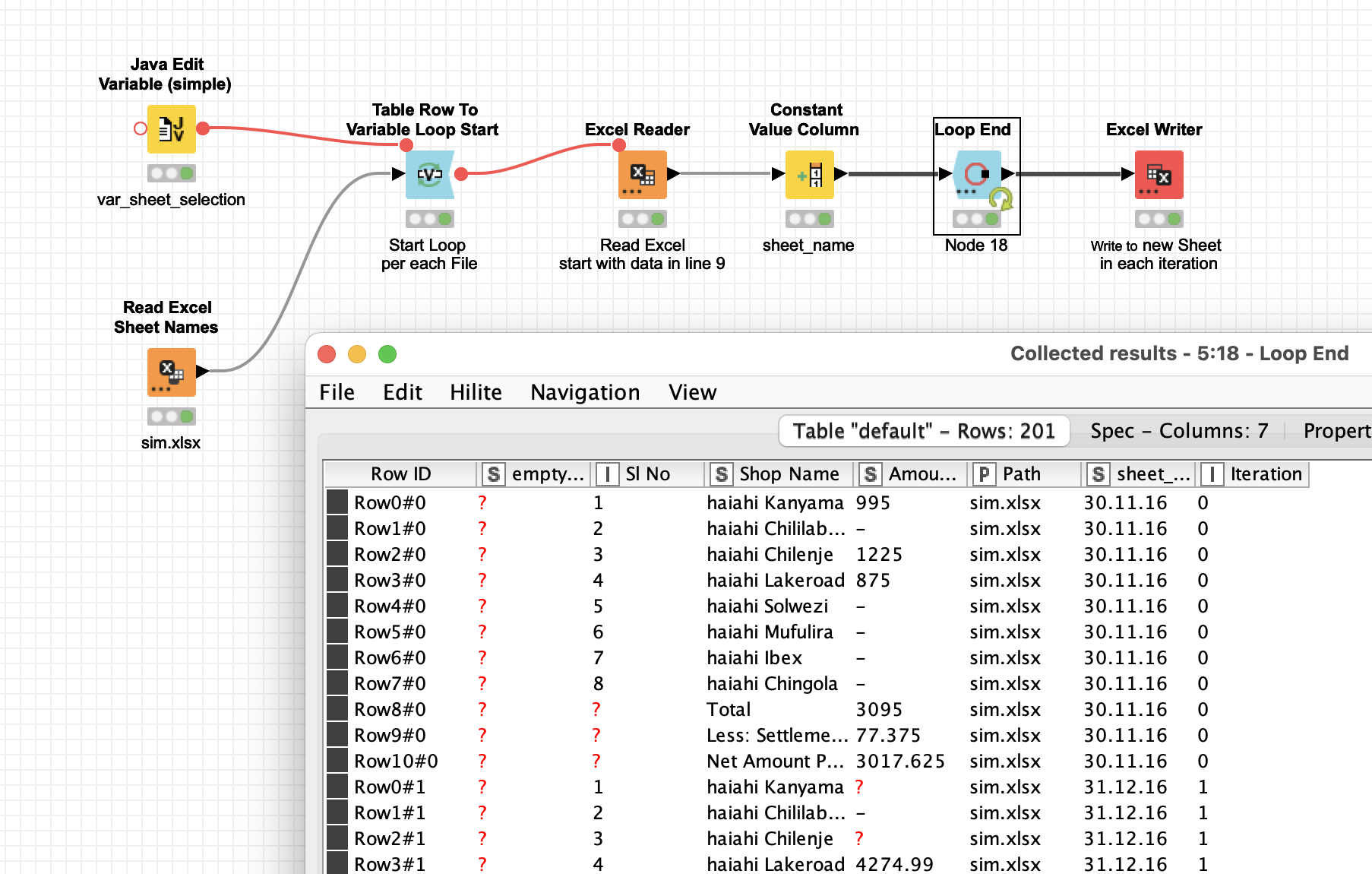
+
Yes, most packages like readxl and openxlsx support both .xls and .xlsx file formats.
What if my Excel file has complex formatting?

+
The openxlsx package is the best option as it preserves formatting and handles styles when reading or writing Excel files.