Reading Excel Files in ASP.NET C# - Simplified

In the dynamic world of web development, handling data is a common task, and often, that data resides in Excel files. Whether it's for importing customer data, financial records, or inventory lists, Excel remains a preferred format due to its universality and ease of use. ASP.NET developers, dealing with C# as their core programming language, need efficient ways to integrate this data into their applications. This blog post guides you through the process of reading Excel files in an ASP.NET C# application, simplifying what can be a complex task.
Understanding Excel File Formats
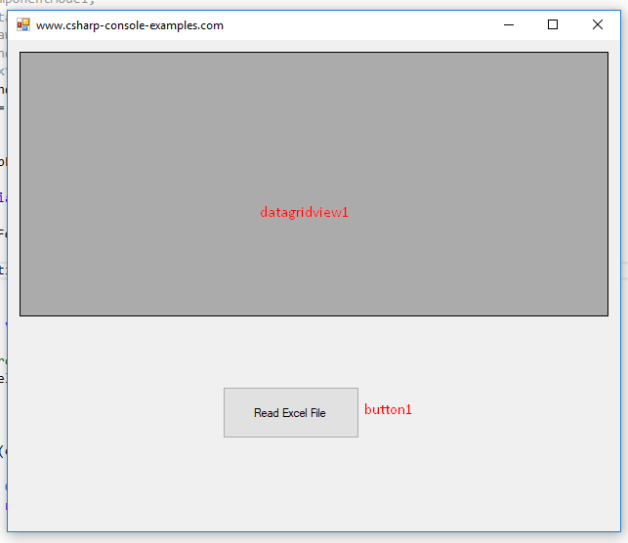
Before diving into the programming side of things, it’s crucial to understand the types of Excel files we might encounter:
- .xls: Microsoft’s older binary file format for Excel 97-2003.
- .xlsx: A newer XML-based format for Excel 2007 onwards, part of Office Open XML.
Each has different considerations when it comes to reading data into your application.
Tools for Excel File Handling

To read Excel files in ASP.NET C#, you’ll need libraries that can parse Excel formats:
- Microsoft.Office.Interop.Excel: Comes with Office installation, but it requires Excel to be installed on the server, which can be problematic.
- EPPlus: An open-source library for managing Excel files without needing Excel installed.
- OpenXML SDK: Allows direct manipulation of the .xlsx file format using XML structures.
For this tutorial, we’ll use EPPlus because of its simplicity and widespread compatibility.
Setting Up Your Environment

Begin by installing the EPPlus NuGet package:
- Open your ASP.NET project in Visual Studio.
- Right-click on your project in the Solution Explorer, choose “Manage NuGet Packages.”
- Search for EPPlus, and install it.
Reading Excel Files with EPPlus

Here’s how to read an Excel file:
var file = new FileInfo(“path/to/your/excel.xlsx”); using (var package = new ExcelPackage(file)) { var workbook = package.Workbook; var worksheet = workbook.Worksheets[1]; // Assuming you’re reading from the first worksheet
int rowCount = worksheet.Dimension.Rows; int colCount = worksheet.Dimension.Columns; for (int row = 1; row <= rowCount; row++) { for (int col = 1; col <= colCount; col++) { var cellValue = worksheet.Cells[row, col].Value; // Process your data here } }
}
Remember to handle exceptions, particularly for file operations or invalid data.
Processing Data from Excel

Once the data is read from the Excel file, you can manipulate it further:
- Data Validation: Ensure the data conforms to expected formats.
- Insertion into Databases: Use ADO.NET or Entity Framework to store the data.
- Displaying Data: Bind the data to ASP.NET controls for visualization or further processing.
⚠️ Note: EPPlus works with .xlsx files. If dealing with .xls, conversion tools might be necessary.
The above example showcases how to read every cell in the Excel file. Depending on your use case, you might want to:
- Read specific ranges.
- Look for headers to understand the structure of your data.
- Handle merged cells or hidden columns.
The approach chosen should reflect the specific needs of your application, ensuring it's efficient and meets performance requirements.
After reading Excel data, integrating it with ASP.NET is the next step:
- Ensure your application can handle large files efficiently by considering multi-threading or batch processing.
- Implement appropriate error handling to manage file corruption or unexpected data formats.
- User Interface: Provide feedback mechanisms to the user about the status of the file upload and processing.
Best Practices

- File Security: Validate uploaded files to prevent server vulnerabilities.
- Performance: Avoid processing large files in memory; consider streaming data if applicable.
- Threading: Use background tasks for file processing to keep your UI responsive.
💡 Note: When handling sensitive data, ensure compliance with data protection regulations like GDPR or HIPAA.
This guide wraps up by summarizing key points for reading Excel files in ASP.NET C#. From understanding file formats to choosing the right tools, setting up your development environment, and executing the file read operation, you now have a comprehensive framework to work with Excel data in your web applications. Remember, the process involves not only reading data but also integrating it smoothly into your ASP.NET applications for further processing or visualization.
Can I use EPPlus to write data to an Excel file as well?

+
Yes, EPPlus is capable of both reading from and writing to Excel files. It provides methods to add, update, or delete cells, rows, and columns.
What if I only need to read specific data from the Excel file?

+
You can read specific data by using range selections, named ranges, or by targeting headers to locate data of interest.
Is there a limit to the file size that EPPlus can handle?

+
EPPlus does not specify a hard limit for file size, but performance will degrade with extremely large files. Server configurations also play a role in handling large files.
Do I need Excel installed on my server to use EPPlus?

+
No, EPPlus is a standalone library and does not require Microsoft Excel to be installed on your server to read or write Excel files.