3 Ways to Read Excel Sheets with Python

Excel spreadsheets are ubiquitous in various industries due to their ease of use for data storage, analysis, and visualization. When dealing with data analysis, automating tasks like reading Excel files can significantly boost productivity. Python, with its robust ecosystem of libraries, makes this task straightforward. In this post, we will explore three effective methods to read Excel files into Python, enhancing your data processing capabilities.
Method 1: Using Openpyxl

Openpyxl is a Python library for reading and writing Excel 2010 xlsx/xlsm/xltx/xltm files. It’s particularly useful for those who need to interact with spreadsheets in an automated fashion.
Installation

First, you’ll need to install Openpyxl. Run the following command:
pip install openpyxl
Reading an Excel File

Here’s how you can open and read an Excel file:
from openpyxl import load_workbook
# Load workbook
workbook = load_workbook(filename="example.xlsx")
# Get sheet by name
sheet = workbook["Sheet1"]
# Iterate over rows
for row in sheet.iter_rows(values_only=True):
print(row)
- This code loads an Excel file named "example.xlsx."
- It then accesses a specific worksheet named "Sheet1."
- Finally, it iterates over all rows, printing their values.
💡 Note: The values_only=True
parameter in iter_rows()
returns only cell values without their formatting or formula information.
Method 2: Pandas
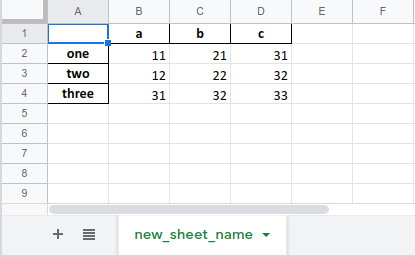
Pandas is one of Python’s go-to libraries for data manipulation and analysis. Its Excel reading capabilities are powerful and widely used.
Installation

Ensure Pandas is installed:
pip install pandas
Reading Excel Files with Pandas

Here’s a simple example to read an Excel file:
import pandas as pd
# Read the Excel file into a DataFrame
df = pd.read_excel("example.xlsx", sheet_name="Sheet1")
# Display the DataFrame
print(df)
- The `read_excel()` function reads the file into a DataFrame object.
- `sheet_name` parameter allows selecting specific sheets.
- Now you can use Pandas' powerful features to analyze or manipulate the data.
Method 3: XLRD

XLRD is an older library that was popular for reading data from older Excel files (like .xls files) but can also work with newer .xlsx files.
Installation

To install XLRD:
pip install xlrd
Using XLRD to Read Excel Files

Here’s how to read an Excel file with XLRD:
import xlrd
# Open the workbook
workbook = xlrd.open_workbook("example.xls")
# Select the first worksheet
sheet = workbook.sheet_by_index(0)
# Iterate over rows and columns
for row in range(sheet.nrows):
for col in range(sheet.ncols):
cell_value = sheet.cell_value(row, col)
print(cell_value)
- This method opens the workbook using `xlrd.open_workbook()`.
- Then it selects the first worksheet using `sheet_by_index(0)`.
- It iterates over all cells, printing each value.
Comparison of Methods

Library | Advantages | Disadvantages |
---|---|---|
Openpyxl | - Supports modern Excel formats - Good for automation and working with formats |
- Slower for large datasets - More complex setup for reading multiple sheets |
Pandas | - Easy to handle multiple sheets at once - Robust data manipulation features |
- Larger memory footprint due to DataFrame structure |
XLRD | - Ideal for legacy Excel files - Lightweight for basic operations |
- Limited support for .xlsx files - Less intuitive for complex operations |

In conclusion, each method offers unique advantages tailored to different needs:
- Openpyxl is excellent for detailed spreadsheet manipulation, particularly when you’re concerned with formats and formulas.
- Pandas stands out for its versatility in data manipulation, making it suitable for large datasets and quick analyses.
- XLRD might be your choice if dealing with older Excel files or when simplicity is key.
Choosing the right method depends on your project’s requirements, the complexity of the Excel files, and your familiarity with these libraries.
Which library is best for reading Excel files with Python?

+
It depends on your needs. Pandas is generally recommended for its data manipulation capabilities, but for specific tasks like formatting, Openpyxl might be better.
Can I read Excel files without libraries in Python?

+
Directly reading Excel files without external libraries is impractical. Libraries like Openpyxl, Pandas, and XLRD provide interfaces to parse Excel files efficiently.
How can I read specific sheets from an Excel file?

+
With Openpyxl, use load_workbook().active
or workbook["SheetName"]
. In Pandas, specify sheet_name
in pd.read_excel()
, and for XLRD, use sheet_by_name("SheetName")
.
Can these methods handle large Excel files?
+Pandas can manage larger files, but performance might degrade with very large datasets. Openpyxl can be slow, and XLRD struggles with newer file formats and large datasets.