5 Ways to Read Excel Sheets in JavaScript Easily
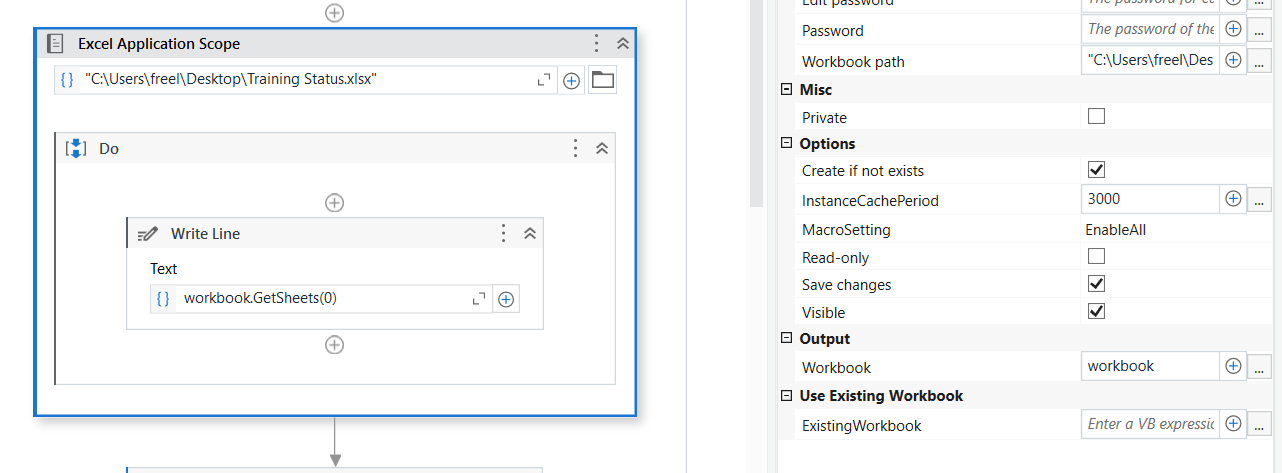
Working with Excel files has long been a staple in many business environments. From financial analysts to human resource departments, the ability to manipulate and read data from Excel spreadsheets is invaluable. Yet, integrating Excel functionality into web applications traditionally required server-side scripting. With the advent of JavaScript, developers can now perform these tasks on the client side, enhancing performance and user interaction. This blog post explores five effective methods to read Excel sheets using JavaScript.
1. Using JavaScript Excel Libraries

One of the easiest ways to handle Excel files in JavaScript is by leveraging established libraries designed specifically for this purpose. Here are some popular ones:
- SheetJS (formerly XLSX.js): Renowned for its speed and compatibility, SheetJS can read and write Excel files, including older formats like XLS and newer ones like XLSX, XLSM, and even ODS.
- ExcelJS: Focused on server-side applications but also works client-side, ExcelJS provides comprehensive control over spreadsheet creation and manipulation.
To use these libraries, you would first include them in your project:
Then, with a few lines of code, you can:
- Read files from an input file element
- Process the Excel file into a workable JavaScript object
- Extract and manipulate data as needed
⚠️ Note: Make sure to check the licensing terms of the libraries before using them in commercial applications.
2. HTML5 File API with FileReader

The HTML5 File API allows direct file manipulation in the browser, providing a client-side solution without the need for external libraries:
- Create an
element to select an Excel file.
- Use the
FileReader
object to read the file.
Here's a basic example:
var fileInput = document.getElementById('excel-file');
fileInput.addEventListener('change', function(event) {
var file = event.target.files[0];
var reader = new FileReader();
reader.onload = function(e) {
var data = e.target.result;
// Parse data here
};
reader.readAsBinaryString(file);
});
3. Utilizing JSON and CSV Intermediaries

If your Excel file can be exported as CSV or JSON:
- You can leverage JavaScript’s built-in parsing capabilities for these formats.
- CSV files can be split by commas and lines to convert into JavaScript arrays or objects.
- JSON, being natively supported by JavaScript, can be parsed with
JSON.parse()
.
This approach simplifies the process but loses some Excel-specific features like cell formatting.
4. Browser Extensions or Add-ons

For users who might not want to deal with code, browser extensions like “Excel Viewer” or “Google Sheets Offline” allow reading Excel files within the browser itself:
- Users can directly open an Excel file in their browser.
- No need to write any code; the functionality is provided by the extension.
💡 Note: While extensions provide an easy way for users, they are less flexible for development purposes and might require installation by the end-user.
5. Utilizing Web Workers for Performance

For handling large Excel files, leveraging web workers can:
- Prevent UI from freezing while parsing complex data.
- Offload heavy computations to background threads.
An example setup:
// main.js
var worker = new Worker("excel-worker.js");
worker.addEventListener("message", handleMessage);
function handleMessage(event) {
console.log(event.data);
}
document.getElementById("excel-file").addEventListener('change', function(event) {
var file = event.target.files[0];
worker.postMessage({file: file});
});
// excel-worker.js
onmessage = function(e) {
// Implement file reading logic here
// Once done, post back results
postMessage("Excel data processed");
};
By the end of this blog post, you'll have a comprehensive understanding of how to integrate Excel functionality into your web applications, enhancing both the developer and user experience.
What are the advantages of using JavaScript for Excel file handling?
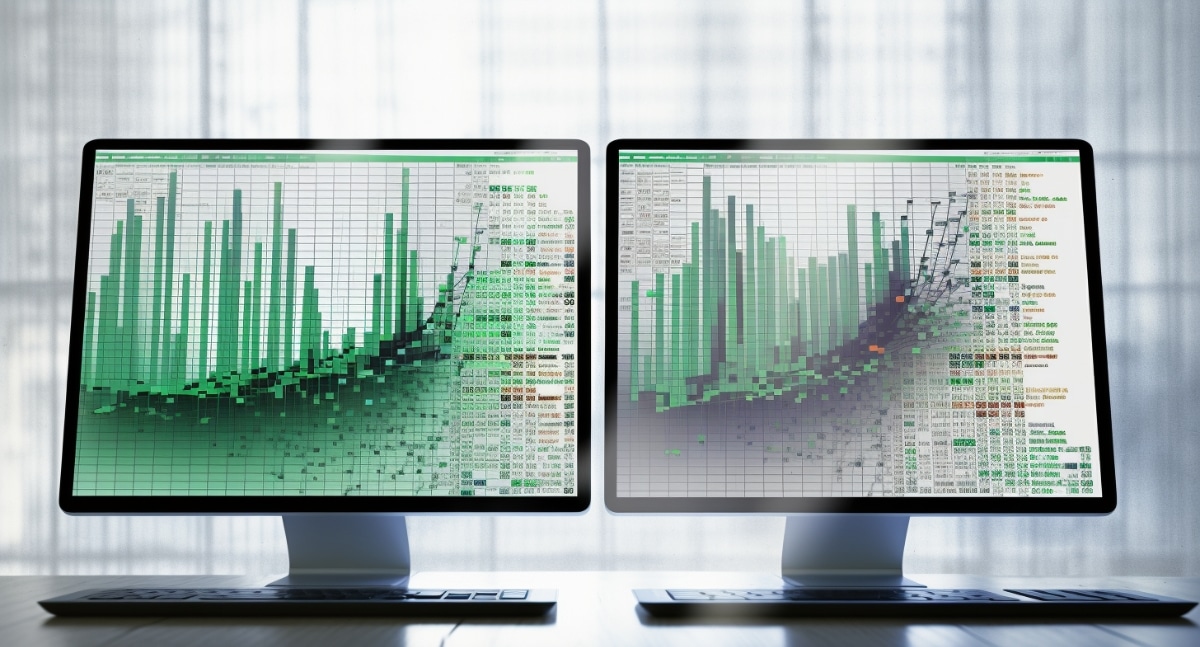
+
JavaScript offers client-side execution, which reduces server load, improves responsiveness, and provides a seamless user experience. It also allows for dynamic data manipulation without reloading pages.
Are there any limitations when reading Excel files with JavaScript?

+
Yes, browser-based JavaScript has limitations, especially with large files, complex formatting, and security restrictions. Also, some advanced Excel features might not be fully supported or interpreted correctly by libraries.
How secure is it to process Excel files on the client side?

+
While JavaScript can read files, security restrictions mean that it can’t access or save data outside the context of the current page or session. Sensitive operations should still be performed server-side for security reasons.