5 Ways to Read Excel Text with Python

Excel files are ubiquitous in data management and analysis, serving as a standard for storing and sharing tabular data across various industries and personal uses. Python, with its vast ecosystem of libraries, offers multiple avenues to read and manipulate Excel files. In this post, we will explore five key methods to read Excel text with Python, covering different libraries and techniques to handle Excel files effectively.
1. Using openpyxl

openpyxl is an incredibly versatile library designed specifically for working with Excel 2010 xlsx/xlsm/xltx/xltm files.
- Installation:
- Reading an Excel file:
!pip install openpyxl
from openpyxl import load_workbook
wb = load_workbook(‘example.xlsx’) sheet = wb[‘Sheet1’] data = [] for row in sheet.iter_rows(values_only=True): data.append(row) print(data)
💡 Note: openpyxl does not support old .xls files; for those, you might need to use a different library like xlrd.
2. Using pandas

pandas is a powerhouse for data manipulation and analysis in Python, providing a simple and efficient way to read Excel files.
- Installation:
- Reading an Excel file:
!pip install pandas openpyxl
(openpyxl is a dependency for pandas to read xlsx files)
import pandas as pd
df = pd.read_excel(‘example.xlsx’, engine=‘openpyxl’) print(df)
🧮 Note: pandas offers additional functionalities like data cleaning, transformation, and analysis post-reading.
3. Using xlrd

For those working with older Excel formats (.xls), xlrd comes to the rescue.
- Installation:
- Reading an Excel file:
!pip install xlrd
import xlrd
book = xlrd.open_workbook(‘example.xls’) sheet = book.sheet_by_index(0) for row_idx in range(sheet.nrows): print(sheet.row_values(row_idx))
🔍 Note: xlrd does not support xlsx files in versions from 2.0.0, so ensure you're using an earlier version for xlsx compatibility or use other methods.
4. Using pyexcel and pyexcel-xls/xlsx
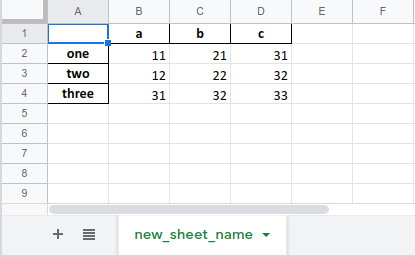
pyexcel provides a unified interface for reading, writing, and manipulating different spreadsheet file types.
- Installation:
- Reading an Excel file:
!pip install pyexcel pyexcel-xls pyexcel-xlsx
import pyexcel
data = pyexcel.get_book(file_name=‘example.xls’) sheet = data.sheet_by_name(‘Sheet1’) for row in sheet: print(row)
5. Using Apache POI with Python

Although not commonly used for Python, Apache POI can be integrated via JPype or jython for those needing to read Excel files in a Java-like manner within Python.
- Setup: You’ll need to install JPype and include POI jars in your Python path. This method is more complex and might require additional configuration.
- Reading an Excel file (pseudo-code):
import jpype jpype.startJVM(classpath=[‘path/to/poi-3.17.jar’, ‘path/to/poi-ooxml-3.17.jar’, ‘path/to/xmlbeans-2.6.0.jar’])
from org.apache.poi.xssf.usermodel import XSSFWorkbook from java.io import FileInputStream
file = FileInputStream(“example.xlsx”) wb = XSSFWorkbook(file) sheet = wb.getSheetAt(0) for row in sheet: for cell in row: print(cell.toString())
jpype.shutdownJVM()
In this comprehensive exploration of Excel file reading techniques with Python, we've covered various methods each with its unique advantages:
- openpyxl for straightforward, direct interaction with Excel workbooks.
- pandas for data analysts and scientists requiring advanced data manipulation.
- xlrd for dealing with legacy .xls files.
- pyexcel for a unified API across different spreadsheet formats.
- Apache POI with JPype or jython for those needing to integrate Java-based solutions.
The choice between these methods often depends on the specific requirements of your project, such as:
- The file format you are working with (xls vs. xlsx).
- Whether you need to perform complex data operations or just read the data.
- The level of control over Excel's features you need (e.g., formulas, charts).
- The integration with existing Python tools and libraries.
Remember, each library has its nuances, so selecting the right tool for your task can significantly affect efficiency and ease of development. Moreover, staying updated with the latest versions of these libraries can ensure compatibility and access to the most recent features and improvements.
Lastly, always consider the context of your project: for simple data extraction, openpyxl or pandas might suffice. If you're dealing with complex spreadsheets or need Java interoperation, Apache POI might be your best bet. Each method has been tried and tested by developers around the world, making Python an excellent language for Excel data manipulation tasks.
Which library should I choose for reading Excel files in Python?

+
The choice depends on your needs. Use openpyxl or pandas for straightforward Excel data extraction; opt for xlrd if you’re dealing with .xls files, or pyexcel for a uniform API across file formats. If you need to integrate with Java for Excel operations, consider Apache POI with JPype or Jython.
Can pandas read both .xls and .xlsx files?

+
Yes, with the appropriate engines: ‘xlrd’ for .xls files (older versions, less than 2.0.0) and ‘openpyxl’ for .xlsx files.
What if my Excel file contains charts or formulas?

+
Excel charts and formulas are often not directly accessible through standard Python libraries. Libraries like openpyxl can read formulas, but charts might not be preserved during import. Apache POI with Python might be needed for such operations.
Is there a performance difference between these methods?

+
Performance can vary; pandas is optimized for data operations and might perform better for large datasets, whereas openpyxl or pyexcel might be more straightforward for smaller datasets or when maintaining Excel-specific features is important.