Reading Excel Sheets in C: A Step-by-Step Guide
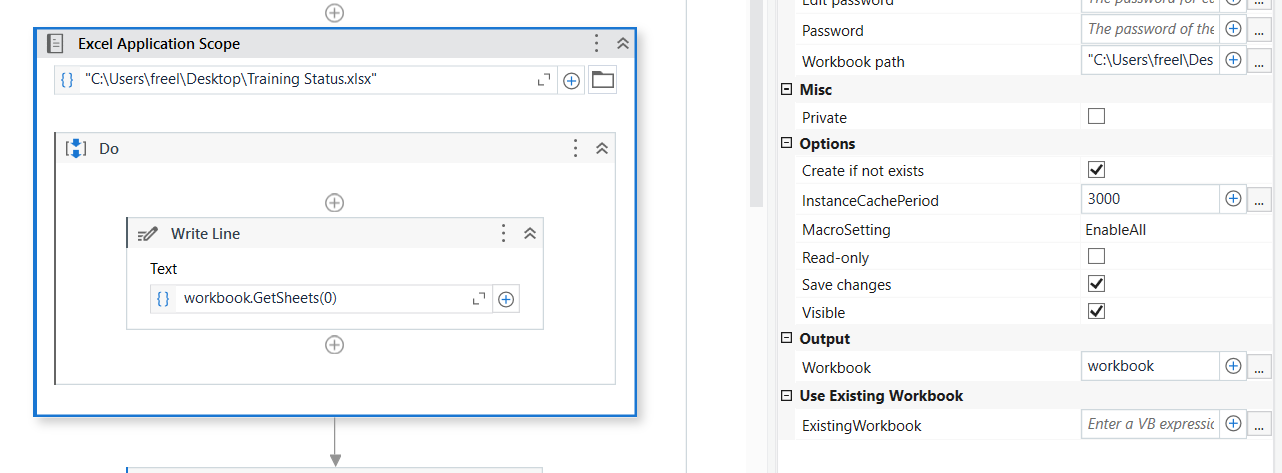
Introduction

Reading Excel files in C is not as straightforward as with languages like Python or R, but it is certainly achievable with the right libraries and techniques. In this comprehensive guide, we’ll explore how you can effectively read and process Excel spreadsheets using C, providing a clear pathway from setting up your environment to executing the code.
Setting Up Your Environment
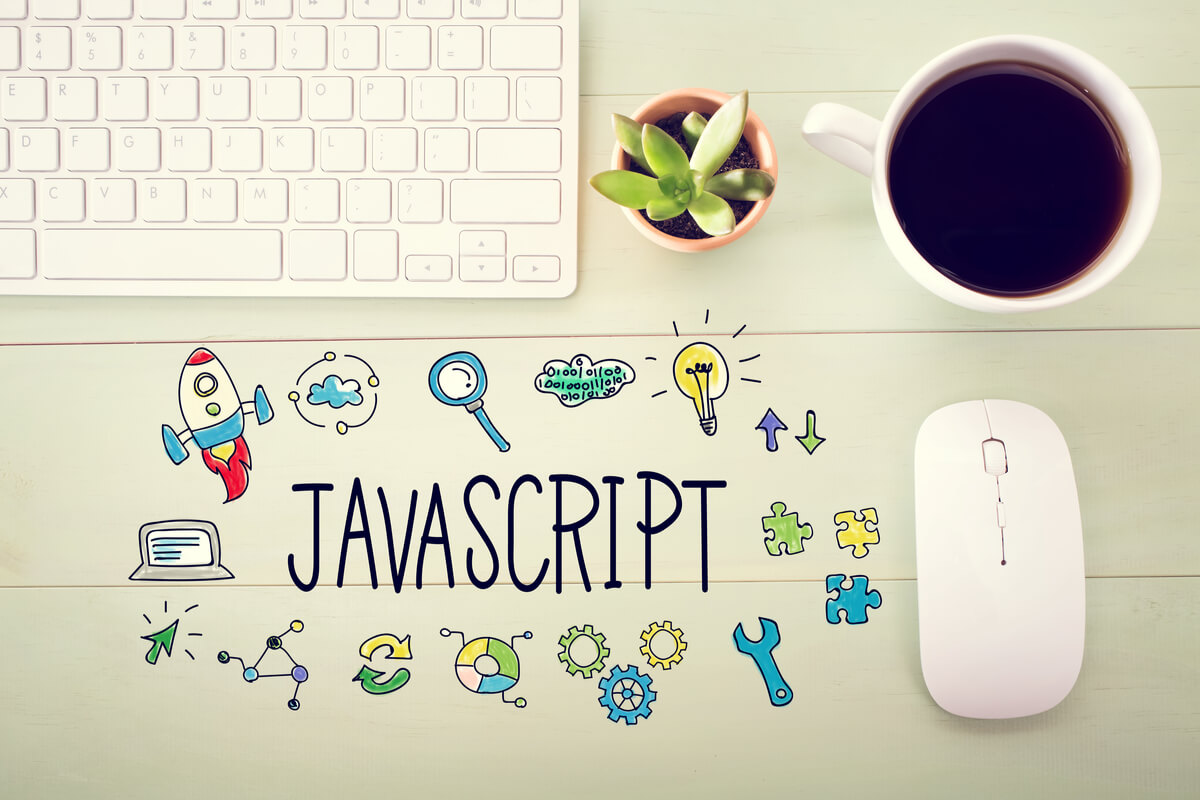
To begin reading Excel files in C, you’ll need to prepare your development environment:
- Install Visual Studio or another C compiler: For this tutorial, we assume you’re using Visual Studio on Windows.
- Library for Excel Reading: Use libraries like LibXL or ExcelDataReader which are designed for Excel file manipulation:
- Download and integrate LibXL or similar libraries into your project.
- Add necessary headers, libraries, and link them during compilation.
💡 Note: Ensure your version of the library supports your Excel file format (xls, xlsx).
Code to Read Excel Files

Here’s how you can write C code to read an Excel file using LibXL:
#include
#include
#include "libxl.h"
int main() {
// Initialize the Excel file
BookHandle book = xlCreateBook();
if (book) {
if(xlBookLoad(book, "data.xlsx")) {
SheetHandle sheet = xlBookGetSheet(book, 0);
if(sheet) {
int row;
for(row = 0; row < xlSheetRowCount(sheet); row++) {
char* cellValue = xlSheetReadStr(sheet, row, 0, 0);
printf("Row %d: %s\n", row, cellValue);
free(cellValue); // Don't forget to free memory
}
}
xlBookRelease(book);
} else {
printf("Unable to load Excel file\n");
}
} else {
printf("Unable to initialize Excel library\n");
}
return 0;
}
Key Points in the Code

- The library function xlCreateBook initializes an Excel workbook.
- xlBookLoad loads your Excel file into memory.
- By using xlSheetGetSheet, we retrieve the first sheet.
- We iterate through rows, reading string data from the first cell of each row with xlSheetReadStr.
- Remember to free any dynamically allocated memory to prevent leaks.
Handling Excel Formats

When dealing with different Excel formats:
- .xls: Older Excel formats can be opened with libraries supporting legacy files.
- .xlsx: Use libraries that support Office Open XML format.
🔍 Note: If dealing with multiple Excel versions, consider using libraries that support both.
Advanced Techniques
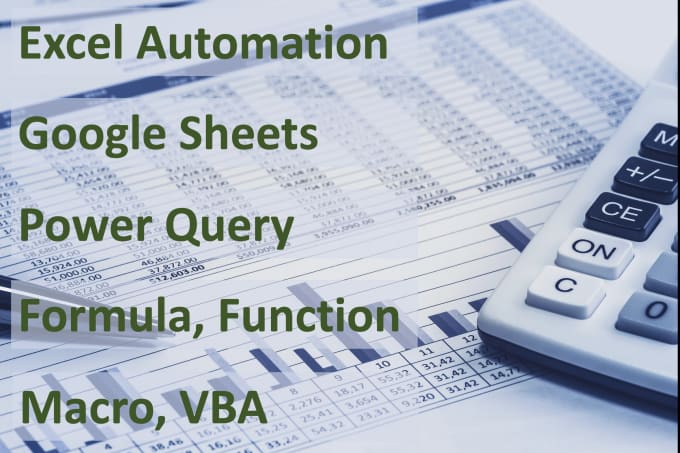
To enhance your Excel file reading capabilities:
- Reading Specific Ranges: Specify cell ranges if you only need data from a particular part of the spreadsheet.
- Handling Data Types: Different libraries might have different ways to read numeric values, dates, or formulas.
- Error Handling: Implement robust error checking to manage issues like file corruption or unsupported formats.
Method | Description |
---|---|
xlSheetReadNum | Read numerical data from a cell. |
xlSheetReadFormula | Read formula from a cell. |
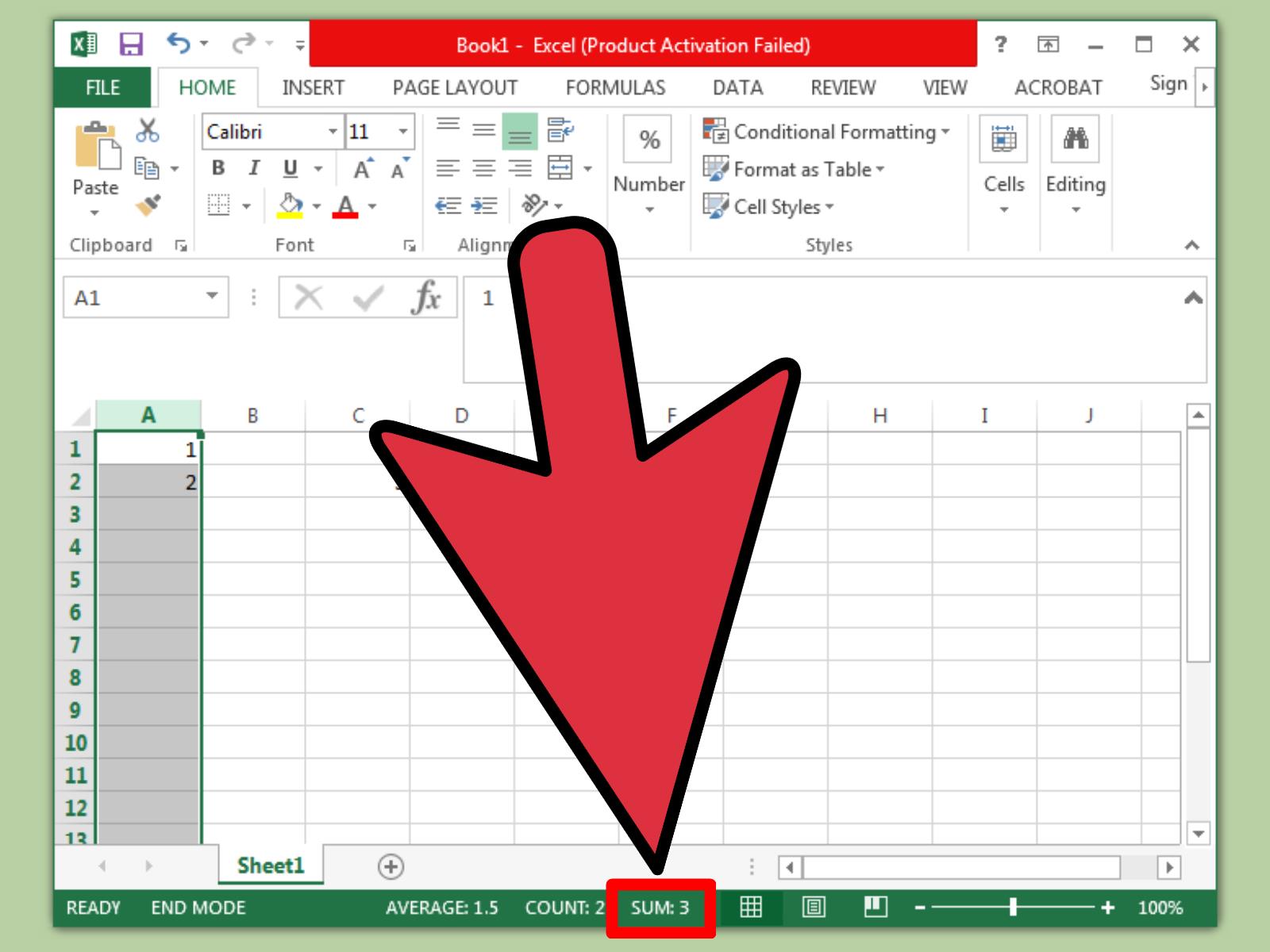
Conclusion
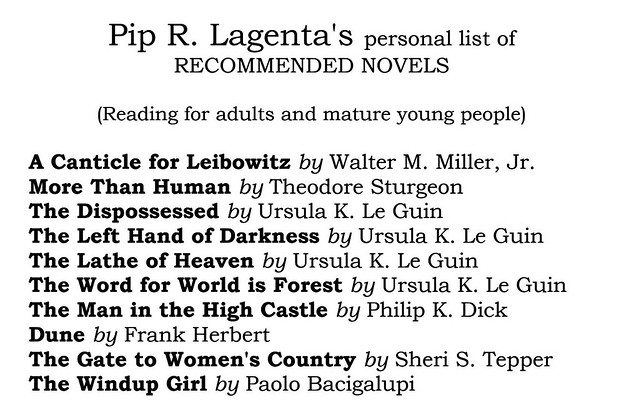
While reading Excel files in C might seem daunting at first, with the right setup and understanding of libraries like LibXL, you can seamlessly incorporate Excel data processing into your C applications. This guide has provided you with the tools and knowledge to start working with Excel files in your C projects, from setting up the environment to implementing basic and advanced reading techniques. By now, you should have a solid foundation to explore further possibilities and customize your Excel interactions in C programming.
Can I read both .xls and .xlsx files using the same library in C?

+
Yes, libraries like LibXL support both old (.xls) and newer (.xlsx) Excel formats. Make sure to check the documentation for version-specific features or limitations.
What if my Excel file is password protected?

+
Some libraries might not directly support reading password-protected Excel files. You might need to decrypt the file first or find a library that explicitly offers this functionality.
How do I deal with complex Excel formatting?

+
Libraries often provide ways to read formatting information, but for complex formatting, you might need to process the data post-reading or use a library with advanced format handling capabilities.