5 Easy Ways to Print Excel Sheets with VB.NET
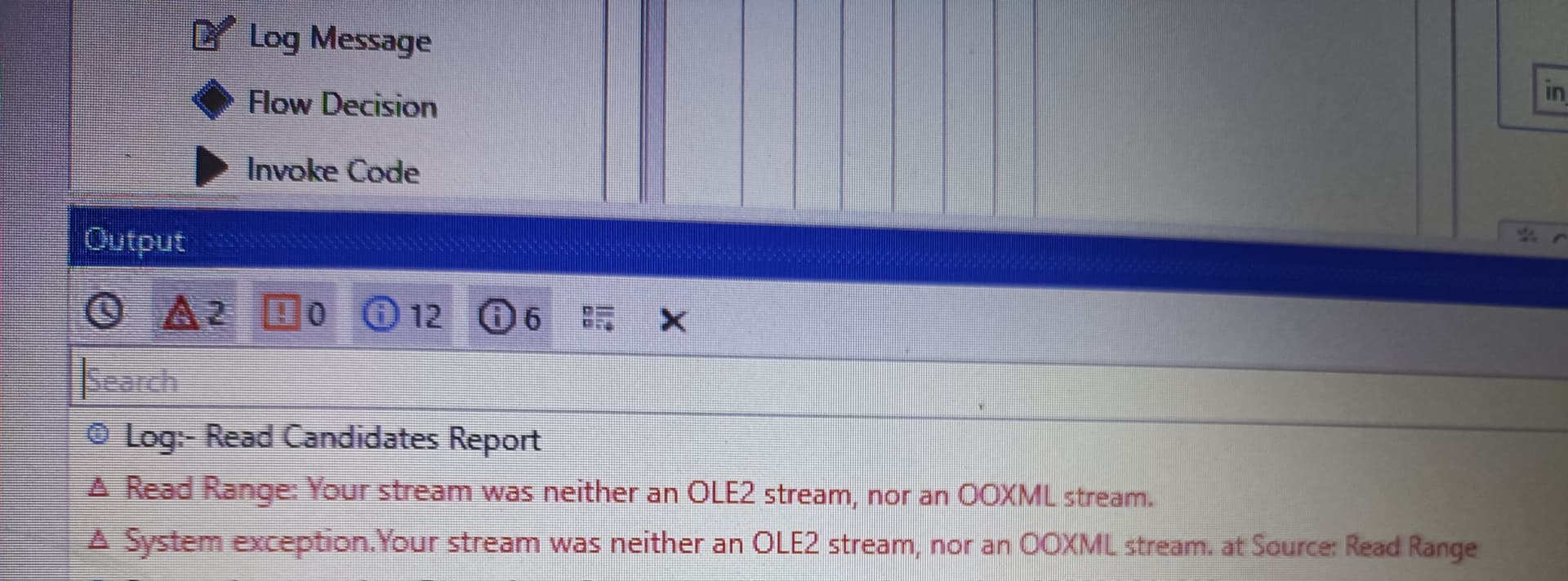
Understanding Excel Printing with VB.NET

Excel, one of the most powerful tools in Microsoft Office Suite, has always been a go-to for data analysis, financial reporting, and managing records. However, when it comes to automation, integrating Excel with a programming language like VB.NET opens up numerous possibilities. One such possibility is printing Excel sheets. This task might seem straightforward, but the integration ensures seamless automation and greater control over the printing process. Let’s explore five easy ways to print Excel sheets using VB.NET.
1. The Basics of Print Control

Before diving into the methods, understanding how to manage the print settings in Excel via VB.NET is essential. Here’s what you need to know:
- How to open an Excel workbook and select a worksheet.
- Controlling the Page Setup to define print margins, header/footer, and page orientation.
- Adjusting the print area to print only selected cells or ranges.
Here’s a simple example of opening an Excel file and setting up the print options:
Imports Excel = Microsoft.Office.Interop.Excel
Dim app As New Excel.Application
Dim wb As Excel.Workbook = app.Workbooks.Open(“C:\path\to\your\excel\file.xlsx”)
Dim ws As Excel.Worksheet = CType(wb.Worksheets(“Sheet1”), Excel.Worksheet)
‘ Setting up page setup
ws.PageSetup.PrintArea = “A1:F20” ’ Print area
ws.PageSetup.Orientation = Excel.XlPageOrientation.xlLandscape
ws.PageSetup.PrintTitleRows = “1:1”
2. Printing Excel Sheets with VB.NET

Let’s delve into the methods for printing Excel sheets:
a. Direct Printing
This method uses the Excel application to print the entire active sheet directly:
ws.PrintOut()
b. Custom Print Range
Sometimes, you might only want to print specific ranges or different sheets within the same workbook. Here’s how:
‘ Print a specific range
ws.Range(“A1:F20”).PrintOut()
c. Printing Multiple Sheets
Printing multiple sheets at once can save time:
wb.PrintOut(From:=1, To:=3) ‘Prints the first three sheets
d. Silent Printing
To avoid the print dialog, use silent printing:
ws.PrintOut(Preview:=False, Copies:=1, Collate:=True)
e. Print to PDF
Sometimes, it’s useful to save your Excel sheet as a PDF instead of printing physically:
ws.ExportAsFixedFormat(Excel.XlFixedFormatType.xlTypePDF, “C:\path\to\your\pdf\file.pdf”)
💡 Note: Always ensure the Excel application is properly closed after printing to avoid leaving it running in the background.
3. Error Handling

When automating Excel, handling errors gracefully is crucial to maintain the robustness of your application:
- Try-Catch Blocks: Use these to manage exceptions that might occur during the printing process.
- Check if Excel is Installed: Before attempting to automate, ensure Excel is installed on the machine.
- File Access: Make sure the Excel file is not locked by another process.
Here’s an example of error handling in VB.NET:
Try
ws.PrintOut()
Catch ex As Exception
Console.WriteLine(“Error during printing: ” & ex.Message)
End Try
4. Interacting with the Printer

While Excel does a lot of the heavy lifting, sometimes you need to interact with the printer settings directly:
- Printer Selection: Programmatically select which printer to use.
- Number of Copies: Define how many copies should be printed.
- Print Collation: Ensure pages are collated or not.
To interact with the printer, you can use:
Dim printers As System.Drawing.Printing.PrinterSettings.InstalledPrinters
For Each printer As String In printers
If printer = “DesiredPrinterName” Then
app.Dialogs(Excel.XlBuiltInDialog.xlDialogPrinterSetup).Show(printer)
End If
Next
5. Enhancing Printing for Large Spreadsheets

Large Excel spreadsheets can be tricky to print, so here are some tips:
- Page Breaks: Manually set or automatically calculate page breaks for better control.
- Fit to Page: Use Excel’s built-in features to shrink the printout to fit on one page if possible.
- Print Preview: Although you might automate the process, offering a print preview option can be beneficial for users.
Can I print an Excel sheet without opening it through VB.NET?

+
Yes, you can automate the process to print an Excel sheet without explicitly opening it in VB.NET, using COM interop to control Excel behind the scenes.
How can I specify a different printer other than the default?

+
You can use the code to access and set the printer through Excel's dialog or by directly interacting with the printer settings in your VB.NET code.
Is it possible to save an Excel sheet as a PDF instead of printing?

+
Absolutely! Excel provides an option to export the sheet to PDF. You can do this programmatically using VB.NET.
Integrating Excel printing into your VB.NET application or automating this process in a business environment can significantly enhance efficiency, reduce human error, and standardize reports. Through understanding the basics of Excel automation, exploring various printing methods, handling errors, and optimizing for large spreadsheets, you can ensure your printing tasks are completed seamlessly. Remember to always close the Excel application properly, handle exceptions, and consider the user’s experience with features like print preview. This not only ensures smooth execution but also demonstrates the power of integrating office applications with VB.NET for automation and control.