Print Excel Sheets with Python: Easy Guide

Mastering the art of automation can significantly boost your productivity. If you're dealing with data in Excel spreadsheets, you've probably realized the potential of automating print-related tasks. This is where Python shines, offering a blend of simplicity, power, and adaptability.
Understanding the Basics of Python and Excel Interaction

Before diving into the printing specifics, let's understand how Python interacts with Excel:
- openpyxl: A powerful library to read, write, and edit Excel 2010+ .xlsx files without Excel.
- xlrd, xlwt, and xlutils: Libraries used for older Excel file formats (.xls).
- COM Object: For Windows users, Python can interact with Excel through COM, allowing direct manipulation.
📝 Note: For most modern applications, openpyxl is recommended due to its support for Excel 2010 onwards.
Setting Up Your Environment

First, ensure you have Python installed, and then:
- Install openpyxl:
pip install openpyxl
- For Windows users interested in COM:
pip install pywin32
Printing Excel Sheets with Python

Here’s how you can automate printing Excel sheets with Python:
Using openpyxl

from openpyxl import load_workbook
# Load workbook
wb = load_workbook(filename="your-excel-file.xlsx")
ws = wb["Sheet1"] # Select sheet
# Print sheet
ws.print_area = 'A1:D10'
wb.save("your-excel-file-printed.xlsx")
📝 Note: This script sets a print area. Adjust it based on your sheet's needs.
Using COM for Windows

import win32com.client
from win32com.client import Dispatch
# Open Excel file
Excel = win32com.client.gencache.EnsureDispatch('Excel.Application')
wb = Excel.Workbooks.Open("your-excel-file.xlsx")
ws = wb.Sheets('Sheet1')
# Print sheet
ws.PrintOut(From=1, To=1) # Print the first page
wb.Close(False)
Excel.Quit()
📝 Note: Ensure Excel is installed on the machine, and be aware of potential version dependencies.
Customization for Printing

Python allows for extensive customization:
- Adjust Print Area: Use
ws.print_area
to define which cells to print. - Page Setup: Set margins, orientation, scaling with openpyxl.
- Fit to Page: Ensure content fits on one or multiple pages.
- Print Titles: Specify rows or columns to repeat at the top of each printed page.
Automating Print Jobs

You might want to automate more than just the printing of a single sheet:
Printing Multiple Sheets
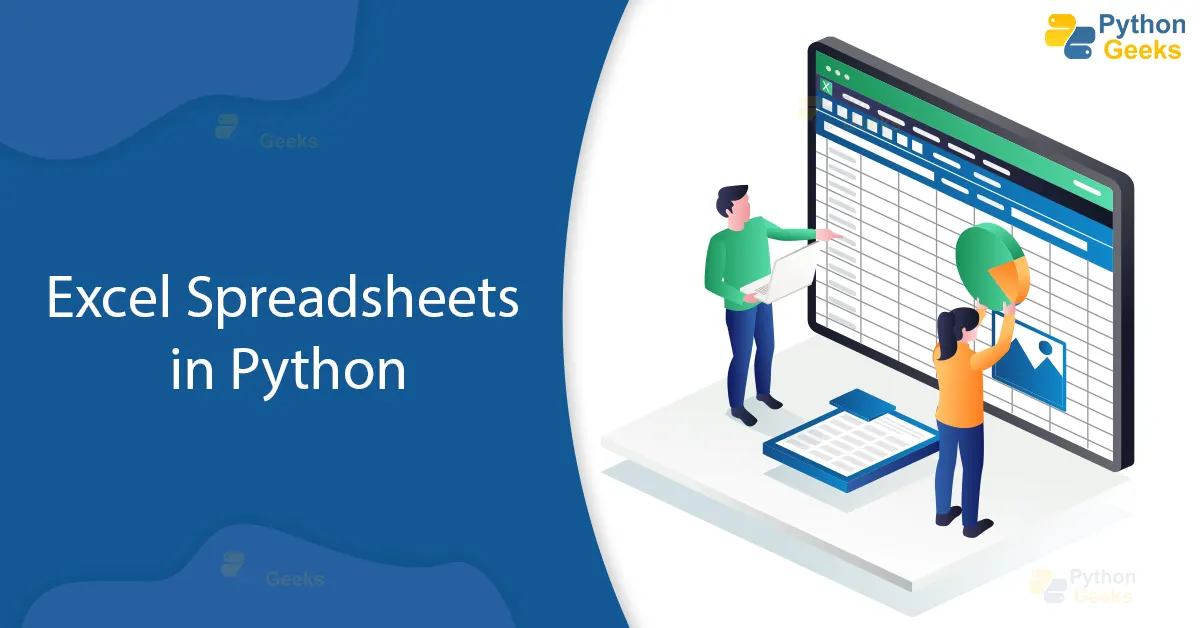
from openpyxl import load_workbook
wb = load_workbook(filename="your-excel-file.xlsx")
for ws in wb.sheetnames:
sheet = wb[ws]
sheet.print_area = 'A1:D10'
wb.save(f"your-excel-file-{ws}.xlsx")
📝 Note: This script will save each sheet as a separate file, ready for printing.
Other Considerations

Beyond the basics, consider:
- Error Handling: Implement robust error handling for file issues or printing errors.
- Print Settings: Explore Excel's print settings, like draft mode or print quality, to fine-tune your output.
In this guide, we’ve covered how to automate the printing of Excel sheets using Python. This knowledge allows you to streamline your data management and presentation processes, offering a tangible boost to productivity. By leveraging Python’s versatility, you can customize, scale, and efficiently handle Excel data for any printing needs. The insights provided give you the tools to automate what can often be a tedious task, freeing up your time for more creative and analytical endeavors. Whether you’re dealing with financial reports, project updates, or any data-intensive work, Python has you covered.
What Python libraries can I use for Excel automation?

+
You can use openpyxl for modern .xlsx files, xlrd, xlwt, and xlutils for older .xls files, or utilize Python’s COM object interface on Windows for direct interaction with Excel.
Can I set the print area and customize page setup with openpyxl?

+
Yes, openpyxl allows you to define a print area with ws.print_area
and offers features to adjust page setup, margins, orientation, scaling, and repeat rows/columns as titles.
Do I need Excel installed for Python to print Excel sheets?
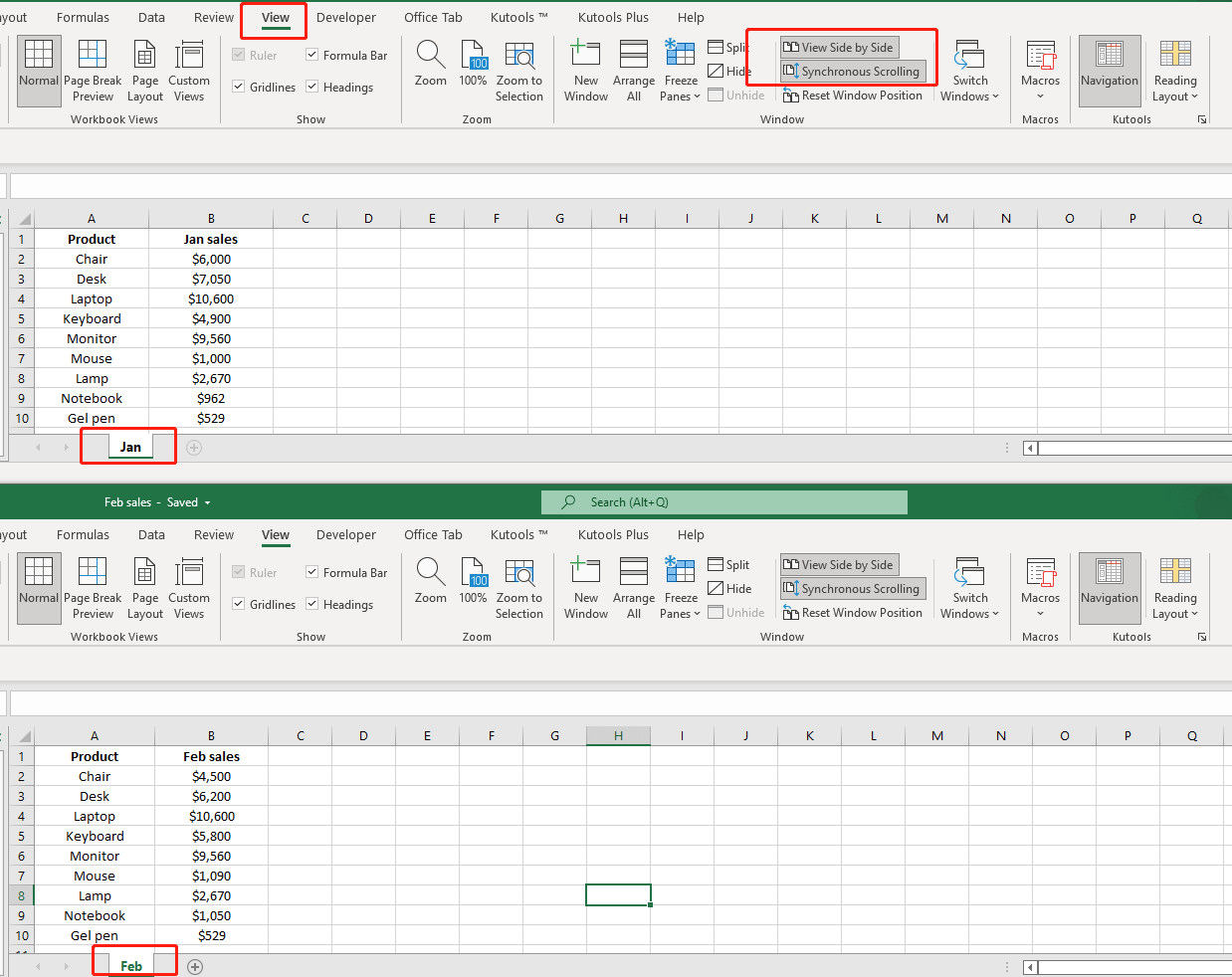
+
For methods like openpyxl, you don’t need Excel installed. However, for methods using COM on Windows, Excel must be present on the system.