Mastering Python Operations with Excel: A Step-by-Step Guide
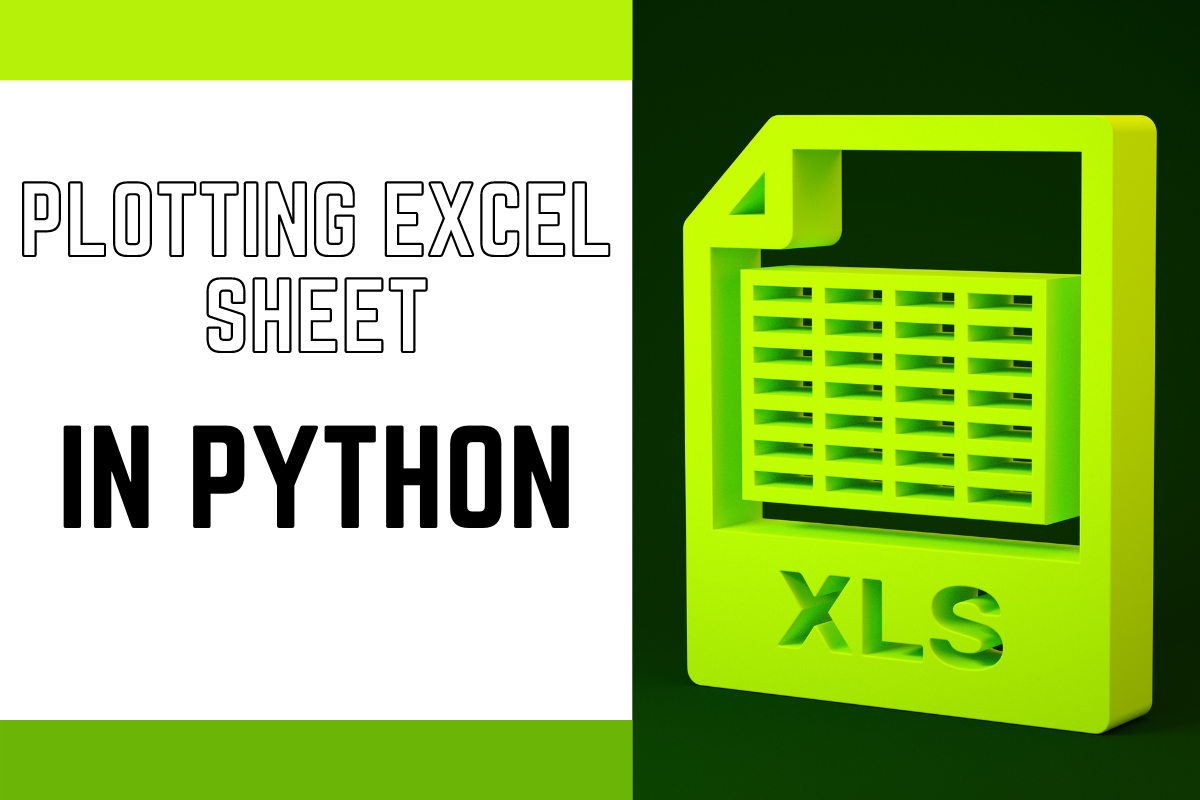
Introduction to Python and Excel Integration

Excel, the go-to spreadsheet software, and Python, a versatile programming language, can together unlock a wide array of data processing and analysis capabilities. Whether you are a data analyst, a business intelligence professional, or someone interested in automating Excel tasks, integrating Python with Excel will significantly enhance your efficiency and expand your toolkit.
Setting Up Your Python Environment for Excel

Before we dive into manipulating Excel from Python, ensure your environment is ready:
- Install Python: Download the latest version from the official Python website and install it.
- PIP and Virtual Environment: Use pip, Python's package manager, to install virtual environment tools. Create and activate a virtual environment to manage dependencies cleanly.
- Required Libraries: Install openpyxl, pandas, xlrd, and xlwt using pip to handle Excel files effectively.
⚙️ Note: Ensure your Python environment is isolated to avoid version conflicts or package incompatibilities.
Interacting with Excel Files Using Python
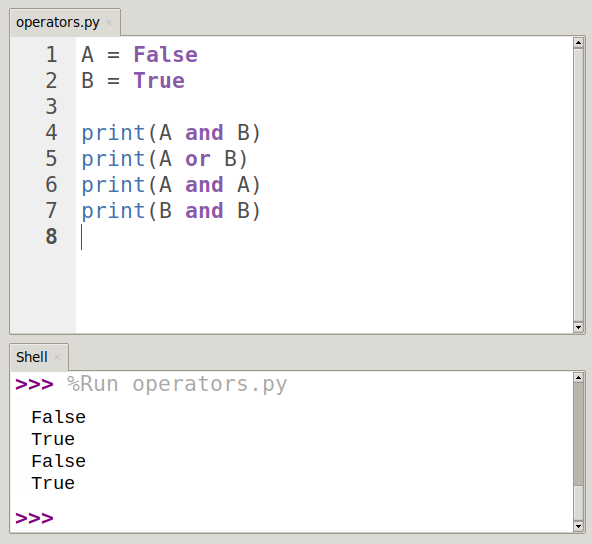
There are several libraries you can use to interact with Excel:
- openpyxl: Ideal for reading, writing, and modifying .xlsx files.
- pandas: Offers robust data manipulation with Excel, but primarily focuses on data structures.
- xlrd/xlwt: Used for .xls files, though these are becoming less common.
Reading Excel Files

Here's how you can read an Excel file:
import pandas as pd
df = pd.read_excel('data.xlsx', sheet_name='Sheet1')
print(df.head())
This code reads 'Sheet1' from 'data.xlsx' and prints the first five rows of the data.
🔍 Note: When using pandas, you can specify the sheet name, the range of cells to read, or skip rows with headers if necessary.
Writing to Excel Files

Here's a way to write data to an Excel file:
import pandas as pd
data = {'Name': ['Alice', 'Bob', 'Cathy'], 'Age': [29, 27, 23]}
df = pd.DataFrame(data)
df.to_excel('output.xlsx', sheet_name='Users', index=False)
This code creates an Excel file named 'output.xlsx' with a sheet 'Users' containing the specified data.
✍️ Note: When writing, you can control various aspects like the formatting of cells or adding styles.
Modifying Excel Files
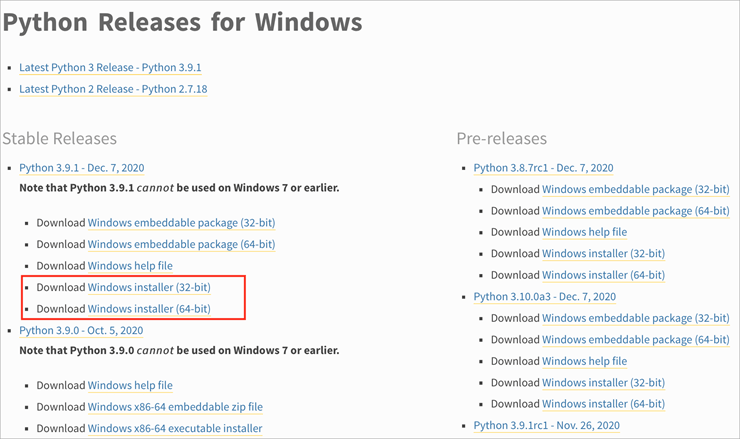
Modifying existing Excel files can be more complex, but here’s a basic example using openpyxl:
from openpyxl import load_workbook
wb = load_workbook('data.xlsx')
ws = wb.active
ws['A1'] = 'Updated'
wb.save('data_updated.xlsx')
This modifies cell A1 in the active sheet and saves it to a new file.
Automating Common Excel Tasks

Python can automate numerous Excel tasks, from basic data entry to complex data analysis:
- Data Validation: Check for duplicate entries or validate email formats.
- Data Cleaning: Remove extra spaces, convert formats, or standardize data entries.
- Conditional Formatting: Highlight cells based on conditions using Python's logic.
- Formulas: Insert calculated fields or automate complex formula insertion.
Example: Automating Data Entry

Let's say you have a list of names to enter into an Excel sheet:
from openpyxl import Workbook
wb = Workbook()
ws = wb.active
names = ['Alice', 'Bob', 'Cathy']
for index, name in enumerate(names, start=1):
ws[f'A{index}'] = name
wb.save('names.xlsx')
Using Python for Excel Analysis
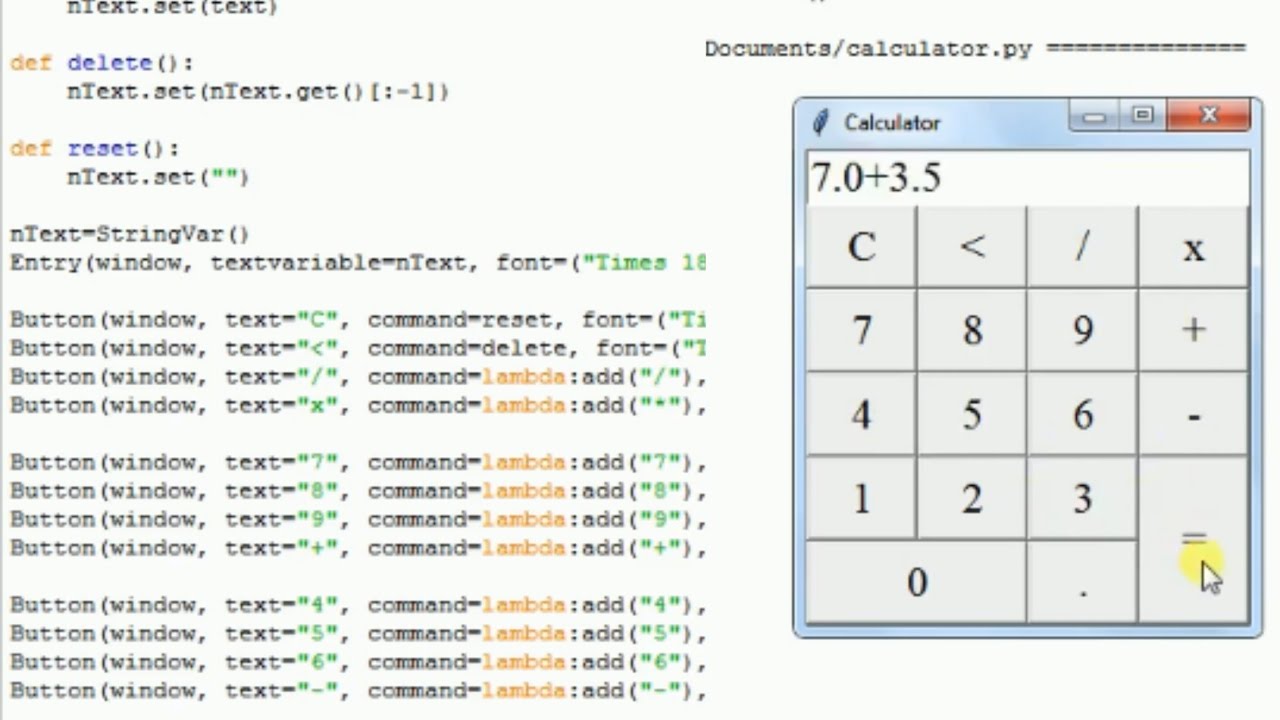
Beyond simple read/write operations, Python with Excel allows for sophisticated data analysis:
- Data Visualization: Use libraries like Matplotlib or Seaborn to generate charts and graphs from your Excel data.
- Machine Learning: Incorporate Scikit-learn to analyze trends or predict outcomes based on Excel data.
- Web Scraping and Integration: Automate the process of pulling data from websites into your Excel spreadsheets.
Example: Data Visualization

Here’s an example of reading Excel data into a pandas DataFrame, then creating a line plot:
import pandas as pd
import matplotlib.pyplot as plt
df = pd.read_excel('data.xlsx')
df['Date'] = pd.to_datetime(df['Date']) # Convert 'Date' to datetime if necessary
plt.plot(df['Date'], df['Value'])
plt.title('Data Analysis Over Time')
plt.xlabel('Date')
plt.ylabel('Value')
plt.show()
Having explored the integration of Python with Excel, it's clear that the capabilities extend far beyond basic spreadsheet manipulation. Whether for automating mundane tasks or for leveraging the analytical power of Python, Excel and Python together form a dynamic duo for data professionals. This guide has taken you from setting up your Python environment to performing complex data manipulations and visualizations, showcasing the breadth of possibilities when these two tools are used in harmony.
Can Python read older .xls file formats?
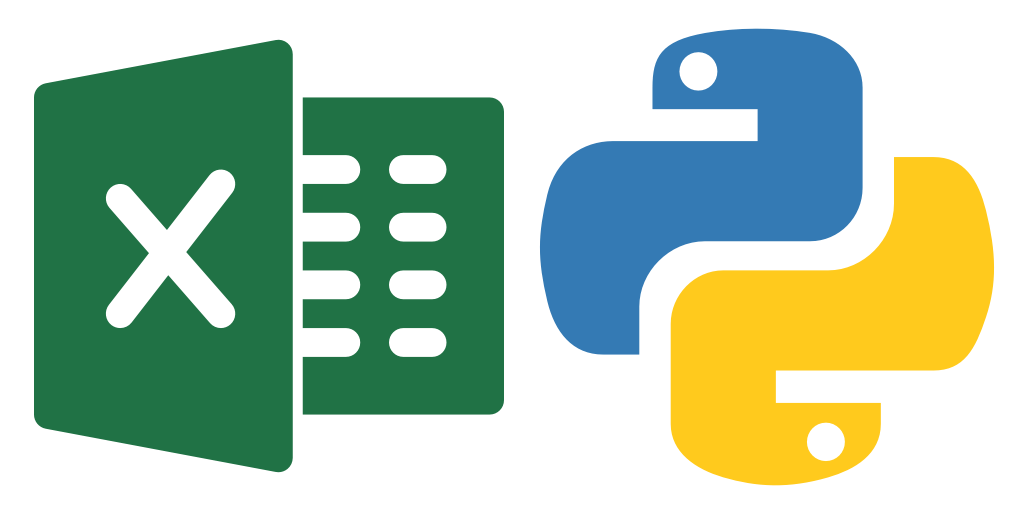
+
Yes, Python can read older .xls files using the xlrd library. You can use pd.read_excel(‘file.xls’, engine=‘xlrd’) with pandas to read these files.
How do I handle complex Excel formatting in Python?

+
Libraries like openpyxl provide extensive support for formatting. You can set cell styles, conditional formatting, and much more through Python.
Is there any performance difference between using Excel directly and Python?

+
Python can process large datasets much faster than Excel, especially when using optimized libraries like pandas. However, for smaller datasets, the difference might be negligible.
Can Python be used for real-time Excel data processing?
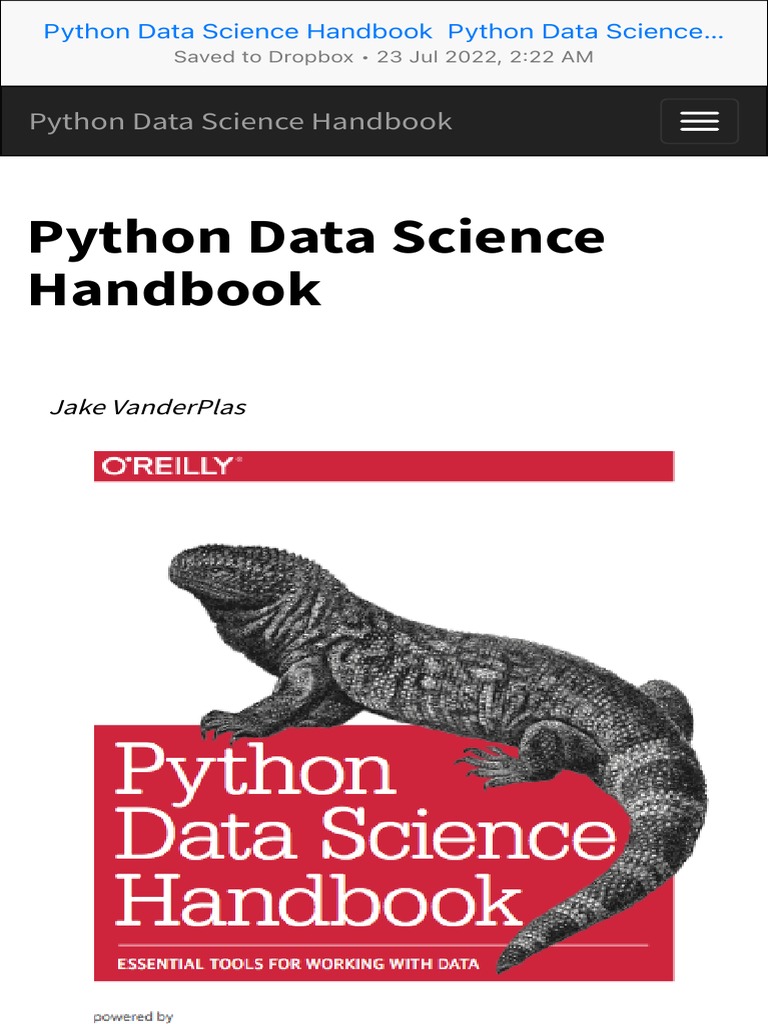
+
While Python isn’t designed for real-time Excel updates, you can simulate real-time data processing by frequently refreshing or updating an Excel file with Python scripts.