5 Ways to Open Specific Excel Sheets with Python

When working with Excel spreadsheets in Python, you have a multitude of tasks you might need to accomplish, from simple data extraction to complex data manipulation. One of the first steps in any data analysis or automation involving Excel is to open and interact with specific sheets within an Excel file. Here are five effective methods to open specific Excel sheets with Python:
1. Using openpyxl
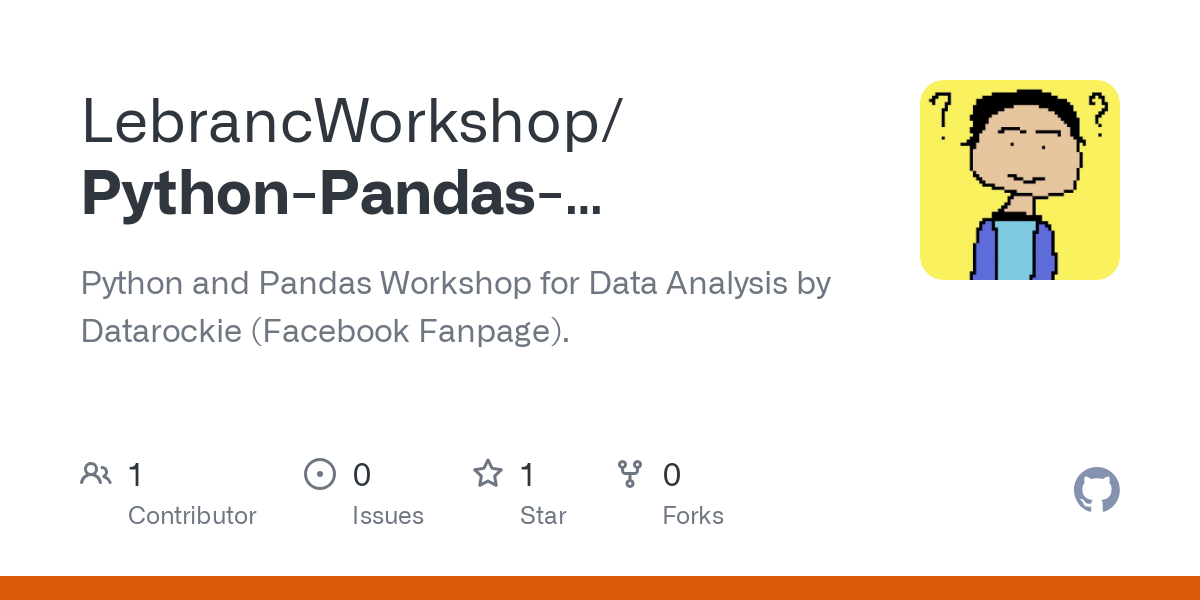
One of the most popular libraries for working with Excel files is openpyxl. This library provides tools to read, write, and manipulate Excel 2010 xlsx/xlsm/xltx/xltm files.
- Installation: Ensure you have openpyxl installed in your environment by running
pip install openpyxl
. - Code Sample:
from openpyxl import load_workbook
workbook = load_workbook(filename=“example.xlsx”)
sheet = workbook[“Sheet1”]
After loading the workbook, you can access or manipulate any sheet by its name. This method is particularly useful when you know the exact name of the sheet you’re interested in.
2. Using pandas

Pandas is another widely used Python library, mainly used for data manipulation and analysis, but it can also handle Excel files effectively.
- Installation: Install pandas with
pip install pandas openpyxl
, as pandas needs openpyxl to read Excel files. - Code Sample:
import pandas as pd
df = pd.read_excel(‘example.xlsx’, sheet_name=‘Sheet1’)
This method is excellent if your goal is to perform data analysis or manipulation since pandas DataFrames offer many built-in methods for data handling.
3. Using xlrd for Older Excel Files

If you are dealing with older Excel files (.xls format), xlrd is the library you need.
- Installation: You can install xlrd using
pip install xlrd
. - Code Sample:
import xlrd
book = xlrd.open_workbook(“example.xls”)
sheet = book.sheet_by_name(‘Sheet1’)
👉 Note: xlrd cannot read Excel 2007+ files (.xlsx).
4. Dynamic Sheet Selection

In cases where the sheet names are not known beforehand, or they might change, you can dynamically select sheets:
- With openpyxl:
from openpyxl import load_workbook
wb = load_workbook(“example.xlsx”)
sheet_names = wb.sheetnames
sheet = wb[sheet_names[0]]
This approach is beneficial when working with files where sheet names are not predetermined, allowing for more flexibility in data processing.
5. Automation with openpyxl and Automate

For automation tasks, you might want to not only open sheets but also to perform operations on them:
- Example:
from openpyxl import load_workbook
wb = load_workbook(filename=“example.xlsx”)
for sheet in wb.worksheets: # Perform operations on each sheet for row in range(1, sheet.max_row + 1): for col in range(1, sheet.max_column + 1): # Example: Change font size of all cells sheet.cell(row=row, column=col).font = sheet.cell(row=row, column=col).font.copy(size=14) wb.save(“example_modified.xlsx”)
This example showcases how to open all sheets and automate changes across them. It’s particularly useful when your automation involves formatting or data validation across multiple sheets.
The methods outlined above offer a comprehensive guide on how to access and manipulate Excel sheets in Python. Whether you are performing simple data extraction, in-depth analysis, or automating complex Excel tasks, Python has the tools to make these processes straightforward and efficient. Remember, choosing the right library for your task depends on the version of Excel files you're dealing with and the operations you need to perform. With these tools in your arsenal, handling Excel with Python becomes an enjoyable part of your data workflow.
Can I use these libraries to write data to Excel files as well?

+
Yes, both openpyxl and pandas can be used to write data back to Excel files. Openpyxl provides methods to modify cells directly, while pandas can write DataFrames to Excel sheets.
What if my Excel file has a password?

+
openpyxl does not support reading password-protected Excel files out of the box. You might need to remove the password or use third-party tools to decrypt the file first.
Is it possible to handle Excel files with charts and images?

+
While openpyxl can manage basic operations, handling charts and images requires more advanced libraries like xlsxwriter, which supports creating charts but has limited reading capabilities from existing Excel files.
How do I deal with Excel files on different operating systems?

+
Python code for working with Excel files should work on any OS as long as the required libraries are installed, but always ensure your file paths are correctly formatted for the OS you’re working on.