5 Easy Ways to Open Excel Sheet in C# .NET
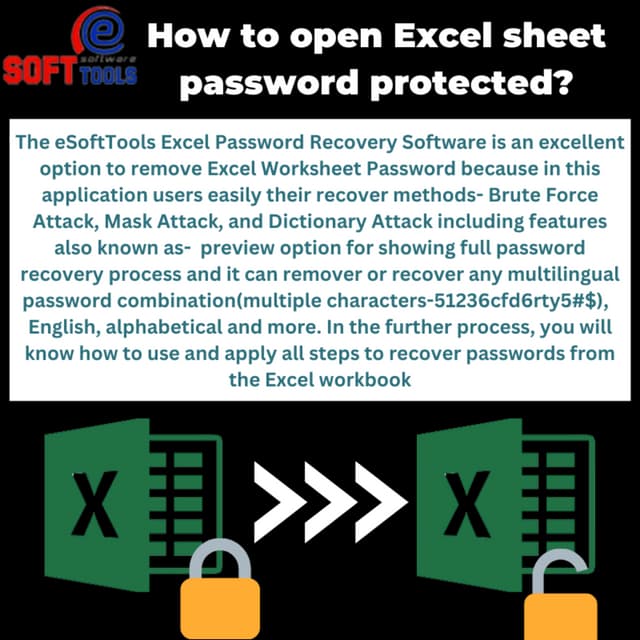
In the world of programming, Microsoft Excel is a powerful tool widely used in business settings for storing, analyzing, and reporting data. Excel sheets can often serve as data sources for many applications, particularly when integrated with C# .NET. Here are five easy methods to open Excel sheets using C#:
1. Using Excel Interop

One of the most straightforward ways to work with Excel in C# is through the Microsoft Office Interop libraries.
<ul>
<li>Ensure you have Microsoft Excel installed on the machine where your C# application will run.</li>
<li>Reference the Microsoft.Office.Interop.Excel assembly in your project.</li>
</ul>
Here’s how you can use Interop to open an Excel file:
<pre>
<code>
var excelApp = new Microsoft.Office.Interop.Excel.Application();
excelApp.Visible = true;
Workbook workbook = excelApp.Workbooks.Open(@"C:\path\to\your\file.xlsx");
Worksheet worksheet = workbook.ActiveSheet;
</code>
</pre>
🚨 Note: This method requires Excel to be installed on the system, which might not be ideal for server applications.
2. Using EPPlus

EPPlus is a library that reads and writes Excel files using the Open Office XML format (.xlsx).
- Installation: Use NuGet Package Manager or Command Line:
<pre> <code> Install-Package EPPlus </code> </pre>
Here’s how to open an Excel file with EPPlus:
<pre>
<code>
using (var package = new ExcelPackage(new FileInfo("path/to/your/file.xlsx")))
{
ExcelWorksheet worksheet = package.Workbook.Worksheets[1];
// Access your data here
}
</code>
</pre>
3. Using OpenXML SDK

OpenXML SDK is another robust option for dealing with Excel files directly from C#.
- Installation: Install from NuGet:
<pre> <code> Install-Package DocumentFormat.OpenXml </code> </pre>
Here’s an example:
<pre>
<code>
using (SpreadsheetDocument document = SpreadsheetDocument.Open(@"path/to/your/file.xlsx", false))
{
WorkbookPart workbookPart = document.WorkbookPart;
WorksheetPart worksheetPart = workbookPart.WorksheetParts.First();
SheetData sheetData = worksheetPart.Worksheet.Elements<SheetData>().First();
// Process sheetData
}
</code>
</pre>
4. Using ClosedXML

ClosedXML is another library built on top of the OpenXML SDK but provides a more user-friendly interface.
- Installation: Install via NuGet:
<pre> <code> Install-Package ClosedXML </code> </pre>
Here’s how you can open an Excel file with ClosedXML:
<pre>
<code>
using (var workbook = new XLWorkbook("path/to/your/file.xlsx"))
{
var worksheet = workbook.Worksheet(1);
// Access your data here
}
</code>
</pre>
5. Using OleDb Connection

If all you need is to read data from an Excel sheet without manipulating it, using an OLE DB Connection can be efficient.
<pre>
<code>
string connectionString = @"Provider=Microsoft.ACE.OLEDB.12.0;Data Source=path\to\your\file.xlsx;Extended Properties=Excel 12.0;";
using (OleDbConnection connection = new OleDbConnection(connectionString))
{
connection.Open();
OleDbCommand command = new OleDbCommand("SELECT * FROM [Sheet1$]", connection);
using (OleDbDataReader dataReader = command.ExecuteReader())
{
while (dataReader.Read())
{
// Process rows
}
}
}
</code>
</pre>
🚨 Note: Ensure you have the Microsoft Access Database Engine installed for this method to work.
In summary, there are various methods to open and interact with Excel sheets in C#:
- Excel Interop works well if Excel is installed and you need basic automation.
- EPPlus and ClosedXML are excellent for manipulating and reading Excel files without requiring Excel installation.
- OpenXML SDK provides more control over Excel file formats but can be less straightforward.
- OleDb Connection is perfect for simple data extraction tasks.
Selecting the right approach depends on your project requirements, whether it’s for reading, writing, or manipulating Excel data.
What are the system requirements for Excel Interop?

+
Excel Interop requires Microsoft Excel to be installed on the system where the application will run.
Can EPPlus work with both .xls and .xlsx formats?

+
EPPlus primarily supports .xlsx files. For .xls, consider using a different library or convert the files first.
Is there a free alternative to EPPlus?

+
Yes, you can use libraries like ClosedXML, which is built upon OpenXML SDK but provides a simpler API.