5 Ways to Open Excel Sheet Instantly with VBA
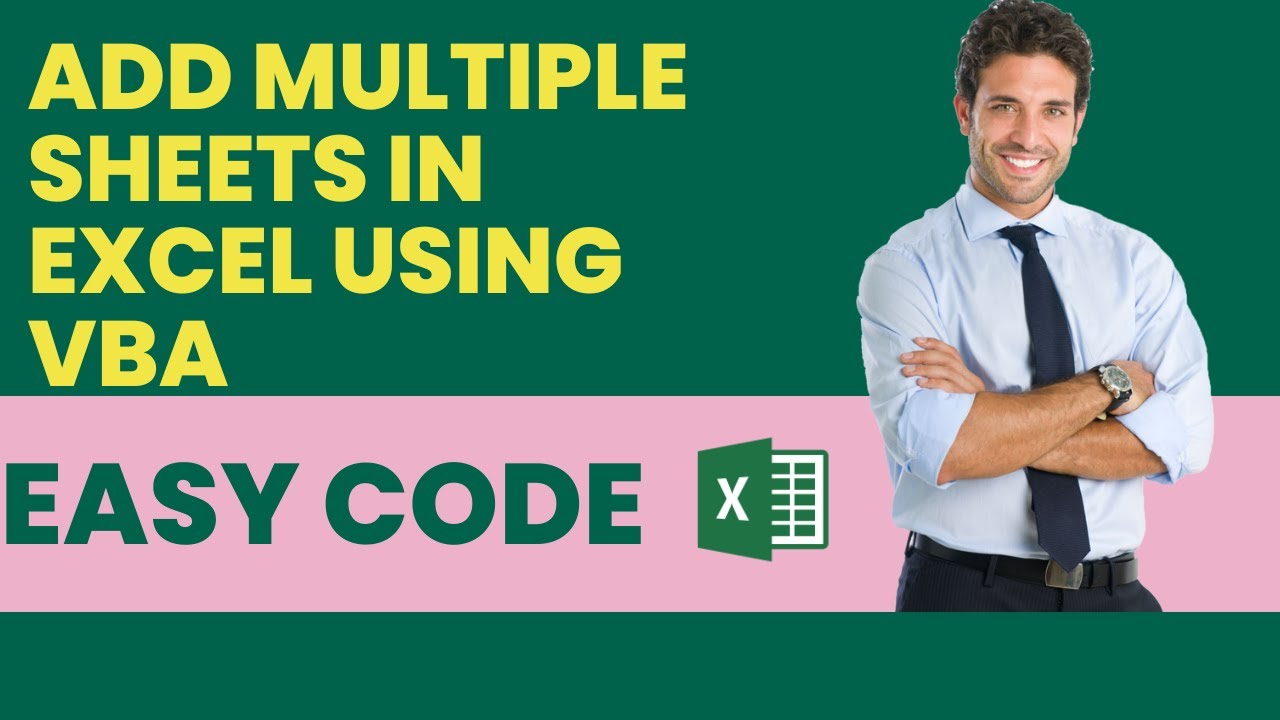
Mastering VBA can significantly boost productivity, especially when it comes to performing routine tasks like opening Excel sheets. VBA, or Visual Basic for Applications, is the programming language of Microsoft's Office Suite, including Excel. It provides a way to automate almost any task you perform frequently in Excel. Here are five efficient methods to open an Excel workbook instantly using VBA:
1. Using the Workbooks.Open Method

The most straightforward method to open an Excel file is by using the Workbooks.Open
method. This function takes the file path as its argument and opens the specified workbook.
Sub OpenWorkbook()
Workbooks.Open "C:\Path\To\Your\File.xlsx"
End Sub
๐ Note: Ensure that the file path is correct; Excel will throw an error if the file does not exist at the specified location.
2. Open a Specific Sheet from the Workbook

You might not only want to open a workbook but also go directly to a specific sheet. Here's how you can automate that:
Sub OpenSpecificSheet()
Dim wb As Workbook
Set wb = Workbooks.Open("C:\Path\To\Your\File.xlsx")
wb.Sheets("SheetName").Select
End Sub
Here, replace "SheetName" with the actual name of the sheet you want to activate upon opening the workbook.
๐ Note: Make sure the sheet name is exact; Excel is case-sensitive with sheet names.
3. Open with a Specific View or Setting

You can customize the workbook opening experience by setting specific views or options upon launch:
Sub OpenWithSpecificView()
Dim wb As Workbook
Set wb = Workbooks.Open("C:\Path\To\Your\File.xlsx", UpdateLinks:=0, ReadOnly:=True)
With wb.Windows(1)
.DisplayGridlines = False
.DisplayHeadings = False
End With
End Sub
This code opens the workbook in read-only mode and sets the gridlines and headings to be hidden.
๐ Note: Different workbooks might have different needs; adjust settings as per your specific requirements.
4. Opening Multiple Workbooks at Once

If you need to open several files simultaneously, you can automate this process with VBA:
Sub OpenMultipleWorkbooks()
Dim i As Integer, files(1 To 3) As String
files(1) = "C:\Path\To\File1.xlsx"
files(2) = "C:\Path\To\File2.xlsx"
files(3) = "C:\Path\To\File3.xlsx"
For i = LBound(files) To UBound(files)
Workbooks.Open files(i)
Next i
End Sub
This method opens three workbooks, but you can adjust the number according to your needs.
5. Dynamic File Open Using File Dialog

Instead of hardcoding the file paths, you can use a file dialog box to select the file at runtime:
Sub OpenFileViaDialog()
Dim FilePath As String
With Application.FileDialog(msoFileDialogFilePicker)
.Title = "Select Excel File"
.AllowMultiSelect = False
.Filters.Clear
.Filters.Add "Excel Files", "*.xls; *.xlsx; *.xlsm"
If .Show Then FilePath = .SelectedItems(1)
End With
If FilePath <> "" Then Workbooks.Open FilePath
End Sub
This method allows users to choose which file to open, making it very user-friendly.
๐ Note: This approach requires user interaction, which might not be ideal for fully automated processes.
VBA offers many ways to enhance your Excel experience, particularly when it comes to managing and accessing your workbooks efficiently. Whether you need to open files programmatically, set up specific views, or deal with multiple workbooks, VBA provides the tools you need to streamline your workflow. Remember, while VBA is powerful, it's also necessary to understand the environment in which it's used, including the implications of macros security settings in Excel. With these techniques in your toolkit, you'll be well-equipped to handle file management tasks with ease.
Can I open files from different directories at the same time?

+
Yes, you can open multiple files from different directories using a loop and specifying each file path within the loop as shown in the โOpen Multiple Workbooksโ example.
Is it possible to open a specific range or cell after opening the workbook?

+
Definitely. After opening the workbook, you can use methods like Range(โA1โ).Select
to focus on a particular cell or range.
How can I ensure security when opening files with VBA?

+
Use the ReadOnly
argument when opening a file to prevent accidental changes. Additionally, be cautious with files from unknown sources, and consider using the Excel Trust Center settings to manage macro security.