Modify Excel Sheets with Python: Quick Guide

As businesses and organizations collect and manage larger volumes of data, Microsoft Excel has solidified its position as a crucial tool for data analysis, organization, and reporting. However, as Excel files grow in complexity, manually updating and modifying them can become a tedious and time-consuming process. This is where Python, with libraries like openpyxl and pandas, comes to the rescue, offering an efficient way to automate these tasks. In this detailed guide, we'll explore how you can leverage Python to modify Excel sheets, saving time and reducing errors.
Getting Started with Python for Excel

The journey to automate your Excel tasks begins with understanding the Python libraries designed for spreadsheet manipulation:
- openpyxl: This is a Python library to read/write Excel 2010 xlsx/xlsm/xltx/xltm files. It's straightforward for tasks like reading from or writing to existing spreadsheets.
- pandas: A powerful data manipulation library that can read, write, and analyze tabular data. It supports Excel files, CSV, SQL, and many other formats.
📘 Note: Before starting, ensure you have Python installed. If not, head over to the Python official site to download the latest version.
Setting Up the Environment

To get started, you'll need to install the necessary libraries:
pip install openpyxl pandas
Reading Excel Files

The first step in modifying an Excel sheet is to be able to read it. Here's how you can do it using both libraries:
Using openpyxl

import openpyxl
wb = openpyxl.load_workbook('filename.xlsx')
sheet = wb.active
Using pandas

import pandas as pd
df = pd.read_excel('filename.xlsx')
📝 Note: Always ensure the Excel file path is correct, or you'll get an error saying the file cannot be found.
Modifying Excel Sheets with Python

Once you've read the data, modifying it is the next logical step. Here's how you can perform some common operations:
1. Changing Cell Values
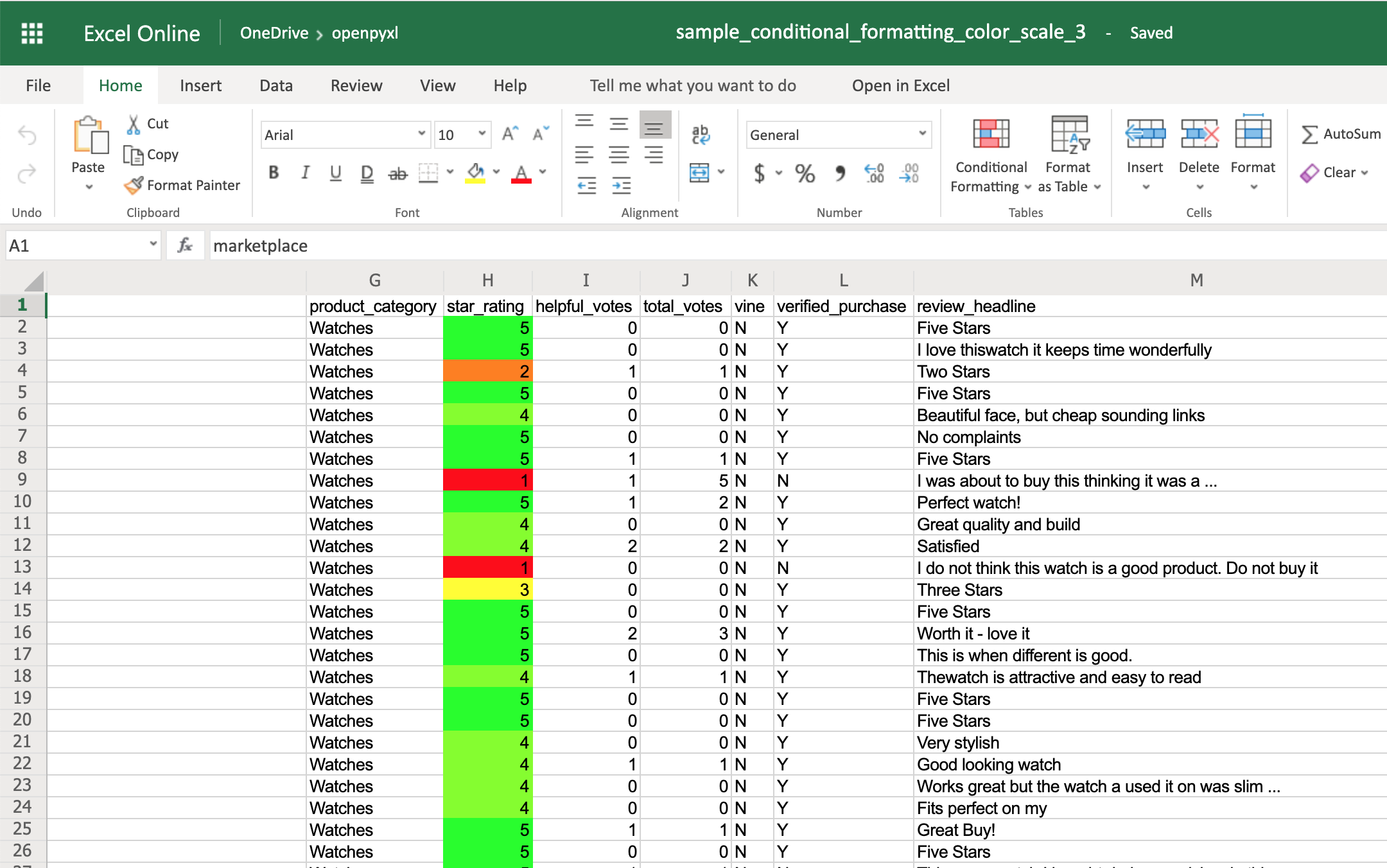
With openpyxl, changing a cell's value is as simple as:
sheet['A1'] = 'New Value'
For pandas, you would change DataFrame values:
df.at[0, 'Column Name'] = 'New Value'
df.to_excel('output.xlsx', index=False)
2. Adding New Rows or Columns

openpyxl:
sheet.append(['Row Data']) # Add a new row
sheet['A1'] = '' # Add a new column by updating an empty cell
pandas:
df.loc[len(df)] = ['Row Data'] # Append a new row
df['New Column'] = '' # Add a new column
3. Deleting Rows or Columns

openpyxl:
sheet.delete_rows(2) # Delete the second row
sheet.delete_cols(2) # Delete the second column
pandas:
df.drop(index=1, inplace=True) # Delete the second row
df.drop(columns='Column Name', inplace=True) # Delete a column
📝 Note: Always use caution when deleting data, as the changes are permanent once saved.
Automation and Efficiency

Here are some ways Python can automate Excel-related tasks for efficiency:
- Batch Operations: You can process multiple Excel files in one go, for example, renaming sheets or cleaning data across all files in a folder.
- Data Validation: Automatically check for data integrity, ensuring that values are within specified limits or match certain criteria.
- Formatting: Apply uniform formatting across multiple sheets or workbooks. This includes colors, fonts, and cell borders.
- Dynamic Reports: Generate reports that can be automatically updated as data changes.
Example: Automating Formatting

import openpyxl
wb = openpyxl.load_workbook('filename.xlsx')
sheet = wb.active
# Make the first row bold
for cell in sheet[1]:
cell.font = openpyxl.styles.Font(bold=True)
# Save the changes
wb.save('filename.xlsx')
Wrapping Up

Harnessing Python for Excel modification not only reduces manual effort but also significantly reduces the risk of human error. By automating routine Excel tasks, you can:
- Focus on more strategic work rather than getting bogged down by data management.
- Ensure consistency and accuracy across complex spreadsheets.
- Save time by letting Python handle repetitive tasks at scale.
In this guide, we've covered the basics of setting up your Python environment for Excel manipulation, reading from Excel files, modifying them, and some ways to automate your tasks for greater efficiency. Whether you're dealing with financial reports, customer data, or any other spreadsheet tasks, Python's power can transform how you interact with Excel.
What is openpyxl?

+
openpyxl is a Python library designed to read/write Excel 2010 xlsx/xlsm/xltx/xltm files. It allows you to automate tasks related to Excel spreadsheets, making data manipulation and analysis easier.
Can I modify charts in Excel using Python?

+
Yes, openpyxl can be used to read and modify chart elements in Excel files, although it has limitations when it comes to complex charts or interactive elements.
How can I automate Excel tasks if I don’t know Python?
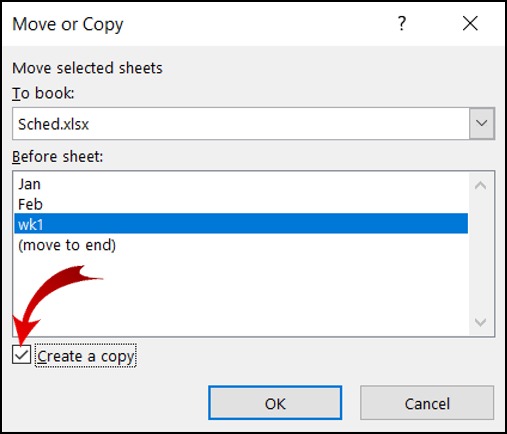
+
If you’re not familiar with Python, you might look into other automation tools like VBA (Visual Basic for Applications) within Excel itself, or even third-party software designed for Excel automation.
Is it possible to merge multiple Excel files with Python?

+
Yes, using libraries like pandas, you can easily read multiple Excel files, merge them based on certain conditions, and save the result to a new Excel file.