Mastering Excel Modifications with Java Apache POI

Excel spreadsheets are indispensable tools for organizing, analyzing, and presenting data. However, when dealing with complex or repetitive tasks, manually manipulating Excel files can be time-consuming and error-prone. Enter Java Apache POI, a powerful library that allows developers to create, modify, and read Excel files programmatically. This comprehensive guide will walk you through the nuances of using Apache POI to modify Excel files in Java, providing step-by-step instructions, examples, and best practices.
What is Apache POI?

Apache POI stands for "Poor Obfuscation Implementation," a name reflecting its humble origins. However, POI has evolved into a robust suite of libraries that supports numerous file formats:
- .xls (BIFF8 format used by Excel 97-2003)
- .xlsx (Excel 2007+ format based on Open XML)
- Other formats like .doc, .ppt, etc. (though this guide focuses on Excel)
Why Use Apache POI for Excel Modifications?

- Automation: Automate data entry, update templates, or generate reports without human intervention.
- Data Integrity: Reduces the risk of human errors during data manipulation.
- Scalability: Efficiently handle large datasets or perform operations on multiple files.
- Integration: Seamlessly integrate Excel modifications into larger applications or systems.
Setting Up Your Java Environment

Before diving into modifying Excel files, ensure your development environment is ready:
- Install Java JDK: Download and install the latest JDK from Oracle or OpenJDK.
- Set Up Your IDE: Use an IDE like Eclipse, IntelliJ IDEA, or NetBeans.
- Add Apache POI Dependencies: Add the necessary libraries to your project:
org.apache.poi poi 5.0.0 org.apache.poi poi-ooxml 5.0.0
Basic Operations with Apache POI

Here are some basic operations you can perform with Apache POI:
Reading an Excel File

import org.apache.poi.ss.usermodel.*;
import java.io.FileInputStream;
public class ExcelReader {
public static void main(String[] args) throws Exception {
FileInputStream fis = new FileInputStream("yourfile.xlsx");
Workbook workbook = WorkbookFactory.create(fis);
Sheet sheet = workbook.getSheetAt(0);
// Iterate through rows and cells
for (Row row : sheet) {
for (Cell cell : row) {
// Determine cell type and print value
switch (cell.getCellType()) {
case STRING: System.out.print(cell.getStringCellValue() + "\t"); break;
case NUMERIC: System.out.print(cell.getNumericCellValue() + "\t"); break;
// ... handle other cell types
}
}
System.out.println();
}
workbook.close();
}
}
Writing to an Excel File

The following example demonstrates how to create an Excel file with some data:
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import java.io.FileOutputStream;
public class ExcelWriter {
public static void main(String[] args) throws Exception {
Workbook workbook = new XSSFWorkbook();
Sheet sheet = workbook.createSheet("Data Sheet");
// Create Rows and Cells
for (int rowIndex = 0; rowIndex < 5; rowIndex++) {
Row row = sheet.createRow(rowIndex);
for (int cellIndex = 0; cellIndex < 3; cellIndex++) {
Cell cell = row.createCell(cellIndex);
if (rowIndex == 0) {
cell.setCellValue("Column" + cellIndex);
} else {
cell.setCellValue("Data_" + rowIndex + "_" + cellIndex);
}
}
}
FileOutputStream out = new FileOutputStream("newfile.xlsx");
workbook.write(out);
out.close();
workbook.close();
}
}
Modifying an Existing Excel File

To modify an existing Excel file, follow these steps:
- Open the Workbook: Use
WorkbookFactory
to open the existing file. - Locate the Sheet: Get the sheet by name or index where modifications are needed.
- Update Data: Create or update cells with new data or formulas.
- Save Changes: Write the updated workbook back to the file.
Advanced Techniques

Handling Formulas

Apache POI can also deal with Excel formulas:
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import java.io.FileInputStream;
import java.io.FileOutputStream;
public class ExcelFormulaManip {
public static void main(String[] args) throws Exception {
FileInputStream fis = new FileInputStream("yourfile.xlsx");
Workbook workbook = WorkbookFactory.create(fis);
Sheet sheet = workbook.getSheetAt(0);
// Add or modify a formula in cell A1 of the first sheet
Cell cell = sheet.getRow(0).createCell(0);
cell.setCellFormula("SUM(B2:B6)");
Cell resultCell = sheet.getRow(0).createCell(1);
resultCell.setCellFormula("A1");
// Write changes back to file
FileOutputStream out = new FileOutputStream("yourfile.xlsx");
workbook.write(out);
out.close();
workbook.close();
}
}
⚠️ Note: Excel can be slow or inefficient when dealing with formulas in large datasets. Consider using functions like evaluateFormulaCell()
from the FormulaEvaluator
class for complex calculations.
Working with Excel Features
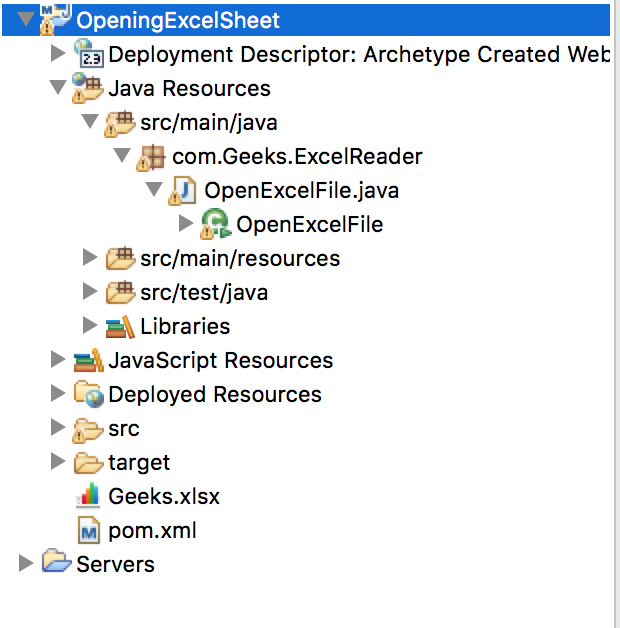
Merging Cells

To merge cells in an Excel worksheet, you can use the following code:
import org.apache.poi.ss.usermodel.*; import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class CellMerger { public static void main(String[] args) throws Exception { Workbook workbook = new XSSFWorkbook(); Sheet sheet = workbook.createSheet(“Merged Cells”); CellRangeAddress mergedRegion = new CellRangeAddress(1, 1, 0, 2); sheet.addMergedRegion(mergedRegion); Row row = sheet.createRow(1); Cell cell = row.createCell(0); cell.setCellValue(“This is merged”);
// Write to a file... }
}
Styling and Formatting

Apache POI provides extensive options to style cells:
import org.apache.poi.ss.usermodel.*;
public class CellStyler { public static void main(String[] args) throws Exception { Workbook workbook = new XSSFWorkbook(); Sheet sheet = workbook.createSheet(); Row row = sheet.createRow(0); Cell cell = row.createCell(0); cell.setCellValue(“Styled Cell”);
// Create a font for the cell Font font = workbook.createFont(); font.setBold(true); font.setFontHeightInPoints((short) 14); font.setColor(IndexedColors.BLUE.getIndex()); // Create a style and apply the font CellStyle style = workbook.createCellStyle(); style.setFont(font); cell.setCellStyle(style); // Apply different styles to other cells... }
}
Putting It All Together

By now, you have the tools to handle basic to advanced Excel manipulations using Java Apache POI. Here are some final tips for efficient Excel modification:
- Memory Management: When dealing with very large spreadsheets, consider reading in chunks or using XSSFEventUserModel for a lower memory footprint.
- Formulas Evaluation: Use
FormulaEvaluator
for immediate formula computation. - Testing: Always test modifications thoroughly in a non-production environment to avoid corrupting crucial data.
- Compatibility: Keep in mind that different versions of Excel might support different features or behave differently.
In summary, Java Apache POI provides a rich set of tools for developers to programmatically manipulate Excel files. From reading and writing data to applying complex styles and handling formulas, this library transforms how you work with spreadsheets in your applications. By mastering these techniques, you'll be equipped to automate, enhance, and streamline data management tasks with precision and efficiency.
What version of Apache POI should I use?

+
It’s advisable to use the latest stable release of Apache POI to benefit from the newest features, performance improvements, and bug fixes. At the time of writing, version 5.0.0 is the latest release. Ensure to check for updates regularly.
Can I modify older Excel formats?

+
Yes, Apache POI supports the older .xls format using the HSSF (Horrible SpreadSheet Format) library, although it has limitations compared to XSSF for .xlsx files.
Is there a performance hit when using Apache POI?

+
Apache POI is memory-intensive for large spreadsheets. For very large datasets, you might consider using SAX (Simple API for XML) parsing methods provided by POI, which reduces memory usage at the cost of some functionality.
How do I prevent file corruption when writing Excel files?
+To avoid file corruption, ensure that you close the workbook properly, use try-with-resources blocks, and always validate the workbook structure before saving.