5 Simple Steps to Merge Excel Sheets in C

If you're managing multiple Excel spreadsheets and need to consolidate data for comprehensive analysis or reporting, merging Excel sheets can streamline your workflow significantly. This article will guide you through five straightforward steps to merge Excel sheets using C#.
Setting Up Your Environment

Before diving into the coding aspect, you need to ensure your development environment is correctly set up:
- Install Visual Studio.
- Ensure you have .NET Framework or .NET Core installed.
- Include the necessary libraries like EPPlus or ExcelDataReader for Excel manipulation in C#.
Step 1: Loading and Accessing Excel Files

The first step in merging Excel sheets is to load the files into your C# program. Here’s how:
- Open each Excel file using EPPlus or similar libraries.
- Access the specific sheets you want to merge.
💡 Note: Ensure you have read permissions for all files you intend to load.
using OfficeOpenXml;
ExcelPackage.LicenseContext = LicenseContext.NonCommercial;
using var sourceFile = new ExcelPackage(new FileInfo("source.xlsx"));
var sourceSheet = sourceFile.Workbook.Worksheets["Sheet1"];
Step 2: Creating the Destination Workbook

Next, we'll create a new workbook where all data will be merged:
using var mergedWorkbook = new ExcelPackage();
var mergedSheet = mergedWorkbook.Workbook.Worksheets.Add("MergedSheet");
⚠️ Note: Be mindful of naming conventions for merged sheets to avoid confusion.
Step 3: Merging Data from Multiple Sheets

Now, we'll copy data from the source sheets to our new merged sheet:
// Start from row 2 to avoid header issues
int rowStart = 2;
foreach (var sheet in sourceFile.Workbook.Worksheets)
{
// Copy data from source to merged sheet
for (int row = rowStart; row <= sheet.Dimension.Rows; row++)
{
for (int col = 1; col <= sheet.Dimension.Columns; col++)
{
mergedSheet.Cells[mergedSheet.Dimension.Rows + 1, col].Value = sheet.Cells[row, col].Value;
}
}
}
Step 4: Handling Special Cases

When merging Excel sheets, you might encounter:
- Headers: Decide if you need to merge headers or keep them separate.
- Data Types: Handle different data types like dates, numbers, or formulas.
- Duplication: Manage duplicate entries or merging identical rows.
- Formatting: Preserve or adjust cell formatting.
🔍 Note: Consider how you want to handle empty cells or conflicts during the merge process.
Step 5: Saving the Merged Workbook

The final step is to save your new merged Excel file:
mergedWorkbook.SaveAs(new FileInfo("merged.xlsx"));
💾 Note: Choose an appropriate location to save the file, ensuring you have write permissions.
Key Takeaways

Merging Excel sheets in C# can be an efficient way to combine data from multiple sources. Here's what we've covered:
- Preparation of your development environment with necessary tools and libraries.
- Loading and accessing Excel files for merging.
- Creating a new workbook for merged data.
- Handling special cases like headers, data types, and formatting.
- Saving the final merged workbook.
In summary, while merging Excel sheets can be complex due to various formatting and data management issues, these steps offer a solid foundation to start with. With practice, you'll find even more ways to optimize and refine this process for your specific needs.
Can I merge Excel sheets without headers?
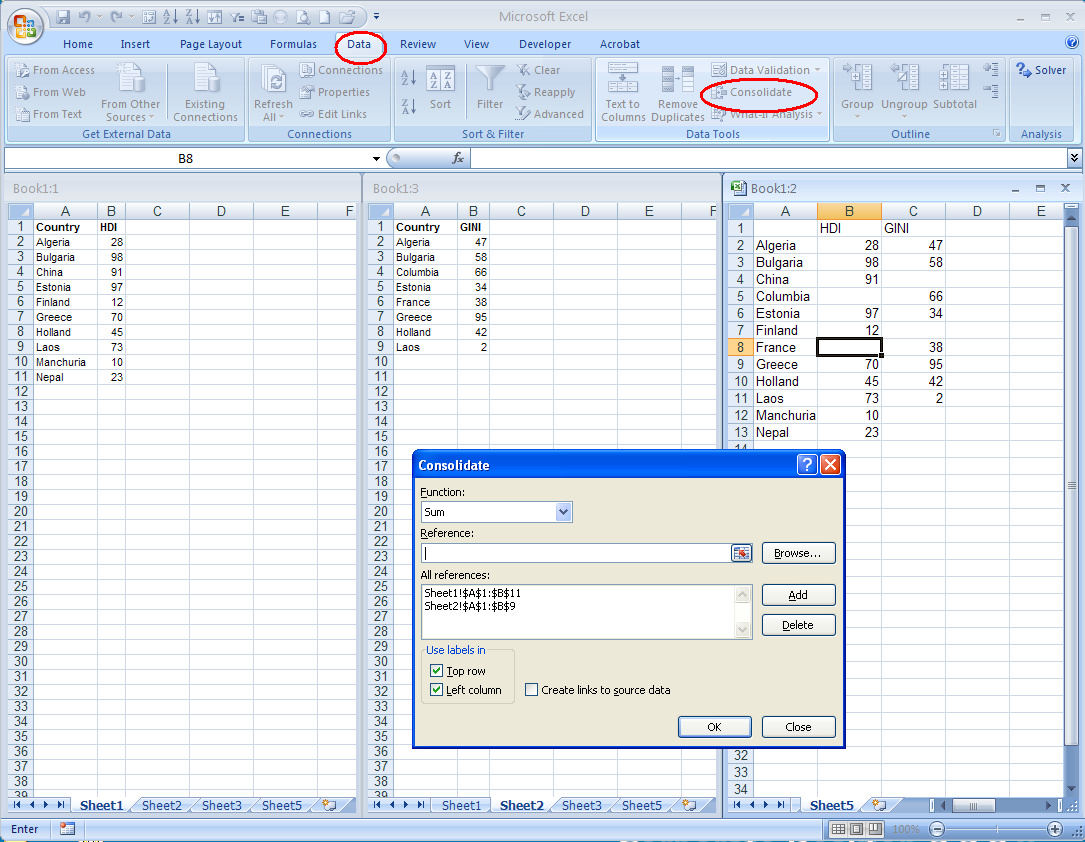
+
Yes, if you ensure that your source sheets do not have headers or you adjust your code to skip or manage headers appropriately.
What if my Excel files have different formats or structures?

+
You would need to normalize the data before or during the merge process. This might involve aligning column names, handling missing data, or restructuring data to fit a common format.
How do I deal with formulas when merging sheets?

+
You might need to convert formulas to values before merging or adjust the formulas post-merge to ensure they reference the correct cells.