5 Ways to Merge Excel Sheets Using VBA Code

Excel spreadsheets are versatile tools for managing and analyzing data. However, when dealing with large amounts of data or when merging multiple sheets becomes a recurring task, manual processes can become inefficient. Here, VBA (Visual Basic for Applications) comes to the rescue. VBA scripts can automate the repetitive task of merging Excel sheets, saving time and reducing errors. In this article, we will explore five different methods to merge Excel sheets using VBA code. Each method will cater to different scenarios or user needs.
Method 1: Merging Sheets with Identical Headers

The simplest scenario is when all the sheets you want to merge have identical headers. Hereβs how you can automate this with VBA:
Sub MergeIdenticalHeaders()
Dim ws As Worksheet
Dim MasterSheet As Worksheet
Set MasterSheet = ThisWorkbook.Sheets.Add(After:=ThisWorkbook.Sheets(ThisWorkbook.Sheets.Count))
MasterSheet.Name = "Merged Sheet"
For Each ws In ThisWorkbook.Worksheets
If ws.Name <> MasterSheet.Name Then
ws.Rows(1).Copy Destination:=MasterSheet.Range("A1")
ws.UsedRange.Offset(1, 0).Copy MasterSheet.UsedRange.Rows(MasterSheet.UsedRange.Rows.Count).Offset(1, 0)
End If
Next ws
End Sub
How It Works:
- This VBA script adds a new worksheet named "Merged Sheet" at the end of your workbook.
- It then loops through all the sheets, copying the header row from the first sheet and data rows from each subsequent sheet.
- The script ensures that headers are not duplicated in the merged sheet.
π Note: Ensure your sheets' headers are in the first row, and all sheets have identical column orders.
Method 2: Merging Sheets with Different Headers

When your sheets have different headers, you can still merge them, but some thought needs to go into how you want to handle these differences:
Sub MergeDifferentHeaders()
Dim ws As Worksheet
Dim MasterSheet As Worksheet
Dim rng As Range
Dim dataCol As Range
Dim i As Long, col As Long
Set MasterSheet = ThisWorkbook.Sheets.Add(After:=ThisWorkbook.Sheets(ThisWorkbook.Sheets.Count))
MasterSheet.Name = "Merged Sheet"
' Collect all unique headers
For Each ws In ThisWorkbook.Worksheets
If ws.Name <> MasterSheet.Name Then
Set rng = ws.Range("A1", ws.Cells(1, Columns.Count).End(xlToLeft))
For Each dataCol In rng
If Application.Match(dataCol, MasterSheet.Rows(1), 0) Then
col = col + 1
MasterSheet.Cells(1, col) = dataCol
End If
Next dataCol
End If
Next ws
' Now, populate the data
For Each ws In ThisWorkbook.Worksheets
If ws.Name <> MasterSheet.Name Then
Set rng = ws.Range("A1", ws.Cells(ws.Rows.Count, ws.Columns.Count).End(xlUp).End(xlToLeft))
For i = 2 To rng.Rows.Count
For col = 1 To rng.Columns.Count
If Not IsEmpty(rng.Cells(i, col)) Then
On Error Resume Next ' Ignore errors if the header doesn't exist in the master sheet
colIndex = Application.Match(rng.Cells(1, col), MasterSheet.Rows(1), 0)
If Not IsError(colIndex) Then
MasterSheet.Cells(MasterSheet.Rows.Count, colIndex).End(xlUp).Offset(1, 0) = rng.Cells(i, col).Value
End If
End If
Next col
Next i
End If
Next ws
End Sub
How It Works:
- This script first gathers all unique headers from all sheets into the master sheet.
- It then aligns each sheet's data under the corresponding headers in the master sheet, ignoring headers that donβt match.
π Note: This method assumes that data under unmatched headers will be omitted, and you might need to manually align some columns post-merge.
Method 3: Merging Based on a Common Key

When you need to merge sheets but want to ensure data integrity based on a unique identifier:
Sub MergeWithKey()
Dim ws As Worksheet, MasterSheet As Worksheet
Dim lastRow As Long, keyCol As Long, colIndex As Long
Dim key As Variant, srcRow As Range, destRow As Range
Set MasterSheet = ThisWorkbook.Sheets.Add(After:=ThisWorkbook.Sheets(ThisWorkbook.Sheets.Count))
MasterSheet.Name = "Merged Sheet"
' Assume the key is in column A, change if necessary
keyCol = 1
' Add headers to MasterSheet
ws.Copy Destination:=MasterSheet.Range("A1")
For Each ws In ThisWorkbook.Worksheets
If ws.Name <> MasterSheet.Name Then
lastRow = ws.Cells(ws.Rows.Count, keyCol).End(xlUp).Row
For Each srcRow In ws.Range("A2", ws.Cells(lastRow, keyCol)).Cells
Set key = srcRow
Set destRow = MasterSheet.Columns(keyCol).Find(key, LookIn:=xlValues, LookAt:=xlWhole)
If destRow Is Nothing Then
' Key doesn't exist, add a new row
MasterSheet.Rows(MasterSheet.Rows.Count).End(xlUp).Offset(1, 0).EntireRow.Value = ws.Rows(srcRow.Row).Value
Else
' Match key, update relevant columns
For colIndex = ws.UsedRange.Columns.Count To 1 Step -1
If Not IsEmpty(ws.Cells(srcRow.Row, colIndex)) Then
MasterSheet.Cells(destRow.Row, colIndex).Value = ws.Cells(srcRow.Row, colIndex).Value
End If
Next colIndex
End If
Next srcRow
End If
Next ws
End Sub
How It Works:
- This method uses a key column to identify unique rows across sheets.
- If a key matches, it updates data from the source sheet into the master sheet; if not, it adds a new row.
π Note: This method assumes your key data is unique and exists in all sheets to be merged. Adjust the key column if necessary.
Method 4: Merging Sheets by Filtering

If you want to merge sheets but only include data that meets specific criteria:
Sub MergeWithFilter()
Dim ws As Worksheet
Dim MasterSheet As Worksheet
Dim critRange As Range, filterColumn As Long
Dim c As Range
Set MasterSheet = ThisWorkbook.Sheets.Add(After:=ThisWorkbook.Sheets(ThisWorkbook.Sheets.Count))
MasterSheet.Name = "Filtered Merged Sheet"
' Assuming you filter based on column A
filterColumn = 1
' Add headers to MasterSheet
ThisWorkbook.Sheets(1).Rows(1).Copy Destination:=MasterSheet.Range("A1")
' Loop through sheets except the master sheet
For Each ws In ThisWorkbook.Worksheets
If ws.Name <> MasterSheet.Name Then
' Set your criteria range, example: filter for items over 100 in column B
Set critRange = ws.Range("B1:B" & ws.Cells(ws.Rows.Count, 2).End(xlUp).Row)
For Each c In critRange
If c.Value > 100 Then
ws.Rows(c.Row).Copy Destination:=MasterSheet.UsedRange.Rows(MasterSheet.UsedRange.Rows.Count).Offset(1, 0)
End If
Next c
End If
Next ws
End Sub
How It Works:
- This method filters data from each sheet based on a condition (in this case, values greater than 100 in column B).
- The filtered data is then copied to the master sheet.
π Note: Change the filter condition to match your requirements.
Method 5: Custom Merge Based on User Input

For a more dynamic merging approach, you might want to let the user define which sheets to merge and how:
Sub CustomMerge()
Dim wb As Workbook, ws As Worksheet
Dim i As Integer, col As Long
Dim MasterSheet As Worksheet
Dim sourceSheets As Variant
Set wb = ThisWorkbook
Set MasterSheet = wb.Sheets.Add(After:=wb.Sheets(wb.Sheets.Count))
MasterSheet.Name = "User Defined Merge"
' Get the sheets to merge from user
sourceSheets = Application.InputBox("Enter the names of sheets to merge, separated by commas:", Type:=2)
If sourceSheets = False Then Exit Sub
' Loop through user-specified sheets
For Each wsName In Split(sourceSheets, ",")
Set ws = Nothing
On Error Resume Next ' in case sheet doesn't exist
Set ws = wb.Sheets(wsName)
On Error GoTo 0
If Not ws Is Nothing Then
' Copy headers from first sheet encountered
If MasterSheet.UsedRange.Rows.Count = 1 Then
ws.Rows(1).Copy Destination:=MasterSheet.Range("A1")
End If
' Start copying data from row 2 onwards
ws.UsedRange.Offset(1, 0).Copy MasterSheet.UsedRange.Rows(MasterSheet.UsedRange.Rows.Count).Offset(1, 0)
Else
MsgBox "Sheet '" & wsName & "' does not exist.", vbExclamation
End If
Next wsName
End Sub
How It Works:
- This script allows users to input the names of sheets to be merged through an InputBox.
- It then proceeds to copy data from these sheets into a master sheet, aligning the headers accordingly.
π Note: Users must provide the exact names of the sheets they wish to merge.
In conclusion, merging Excel sheets with VBA provides a powerful and flexible approach to handle repetitive data management tasks. Whether you need to merge sheets with identical headers, different headers, require key-based integration, or user-defined criteria, VBA has a method tailored for your needs. By automating these tasks, you can reduce errors, save time, and increase productivity. Itβs worth experimenting with these scripts and tweaking them to fit the exact data structures and business processes in your organization.
Can I merge sheets from different workbooks?

+
Yes, you can modify the VBA scripts to handle workbooks by opening external workbooks or using references to other workbooks within the VBA code.
Will these scripts work on all versions of Excel?
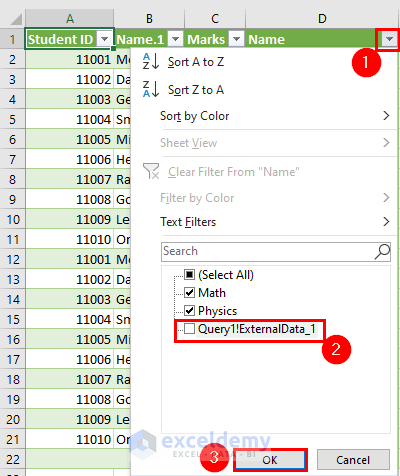
+
The VBA scripts provided should work on Excel 2010 and later versions. However, some functions or properties might differ in earlier versions, requiring adjustments.
How do I handle errors or duplicate data when merging?

+
You can incorporate error handling and checks for duplicate keys or data into your VBA scripts. This might involve using loops to check for existing data before adding new data.