Merge Excel Cells Easily with C: Step-by-Step Guide
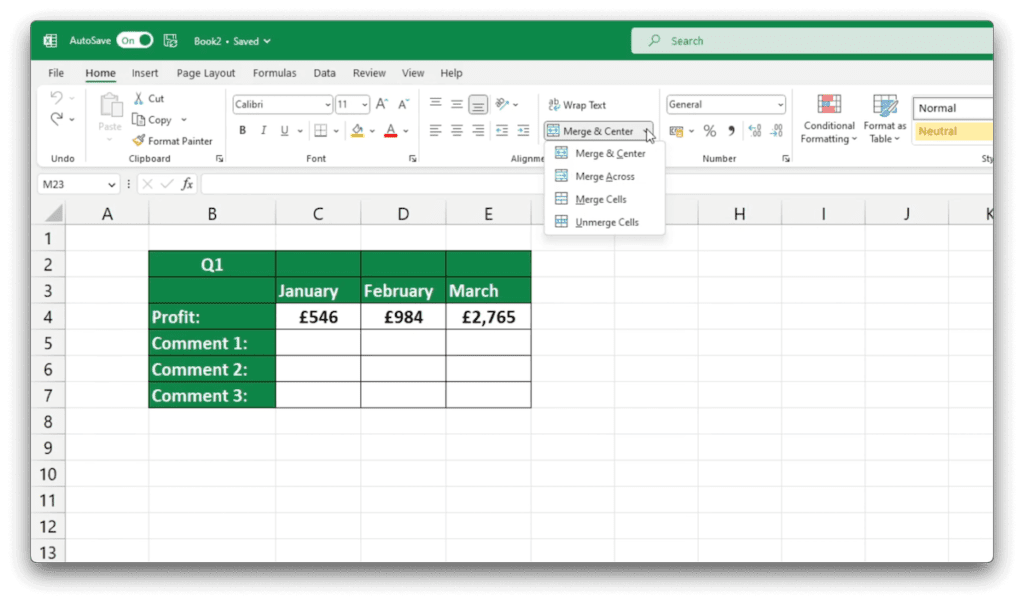
Dealing with Excel spreadsheets can often present unique challenges, particularly when it comes to formatting and data management. One common task users frequently encounter is the need to merge cells in Excel. While this can be easily done with the Excel application's graphical interface, using programming languages like C to automate and manage these operations offers both efficiency and control. This comprehensive guide will walk you through the steps required to merge cells in Excel using C, providing an innovative way to enhance your data manipulation capabilities in Excel files.
Prerequisites

Before diving into the code, make sure you have:
- A C compiler installed on your computer.
- A basic understanding of C programming.
- The Microsoft Excel Object Model installed on your system.
⚠️ Note: Ensure that Excel is installed and licensed on your system, as the C code will interact with the Excel Object Model.
Setting Up Excel Object Model in C

To merge cells in Excel from C, you need to interact with Excel via its Object Model. Here are the steps to set this up:
- Include necessary libraries in your C program.
- Initialize the COM (Component Object Model) interface for Excel.
#include
#include
#include
#include
#include
#include
🔗 Note: These libraries provide functions to interact with COM, which is essential for Excel automation.
Initializing Excel Application

The following steps initialize the Excel application:
- Start the COM library.
- Create an instance of Excel Application.
- Make the application visible to see what’s happening (optional).
// Initialize COM library
HRESULT hr = CoInitialize(NULL);
if (SUCCEEDED(hr)) {
// Create Excel application object
IUnknown *pUnk;
hr = CoCreateInstance(CLSID_Application, NULL, CLSCTX_LOCAL_SERVER,
IID_IUnknown, (void)&pUnk);
if (SUCCEEDED(hr)) {
IDispatch *pDisp;
hr = pUnk->QueryInterface(IID_IDispatch, (void)&pDisp);
if (SUCCEEDED(hr)) {
EXCEL::_Application *pExcel;
hr = pDisp->QueryInterface(&__uuidof(EXCEL::_Application), (void)&pExcel);
if (SUCCEEDED(hr)) {
pExcel->Visible = VARIANT_TRUE; // Optional to make Excel visible
// Now, pExcel can be used to control Excel
}
}
}
}
Merging Cells with C

Here’s how you can merge cells:
- Open a workbook.
- Select the worksheet.
- Select the range of cells to merge.
- Use the Merge method to combine the selected cells.
EXCEL::Workbook *pWorkbooks; EXCEL::Workbook *pWorkbook; EXCEL::Worksheet *pSheet; EXCEL::_Worksheet *pActivesheet; EXCEL::Range *pRange; // Open the first workbook hr = pExcel->Workbooks->Open("C:\\Path\\To\\Your\\Workbook.xlsx", &pWorkbook); if (SUCCEEDED(hr)) { // Get the active worksheet hr = pWorkbook->ActiveSheet->QueryInterface(__uuidof(EXCEL::_Worksheet), (void
)&pActivesheet); if (SUCCEEDED(hr)) { // Get range (A1:D1) for merging hr = pActivesheet->Range[vt_bstr("A1:D1"), (IDispatch**)&pRange]; if (SUCCEEDED(hr)) { // Merge the cells hr = pRange->Merge(); } } }
Handling Errors and Cleanup

When automating Excel from C, it’s crucial to handle errors and clean up resources properly:
- Check for failures in each COM method call.
- Release Excel objects and deinitialize COM.
// Error handling
if (FAILED(hr)) {
// Log error or display message
}
// Cleanup
if (pRange) pRange->Release();
if (pActivesheet) pActivesheet->Release();
if (pSheet) pSheet->Release();
if (pWorkbook) pWorkbook->Close(0);
if (pExcel) pExcel->Quit();
if (pDisp) pDisp->Release();
if (pUnk) pUnk->Release();
CoUninitialize();
⚙️ Note: Proper cleanup is essential to avoid Excel hanging or system crashes.
Automating Excel with C: Further Applications

Beyond cell merging, automation with C can include:
- Formatting cells (font, color, alignment).
- Adding, editing, or retrieving data.
- Creating charts or performing calculations.
This guide provides a solid foundation for automating Excel tasks with C, but remember, Excel's object model is vast, allowing for complex manipulations and custom functionalities based on your specific needs.
Why would someone merge cells in Excel using C?

+
Automating Excel tasks can save time, especially for repetitive actions across large datasets. Merging cells using C can be integrated into broader automation scripts for report generation, data analysis, or system integration where Excel is used as a backend data format.
Can I automate other Excel operations with C?

+
Yes, you can perform a wide range of operations including data entry, formatting, calculations, and even creating macros or add-ins for Excel through COM automation using C.
What are the limitations of using C to interact with Excel?

+
The main limitations include:
- Complexity in setup and learning curve for COM manipulation.
- Potential issues with Excel compatibility or updates changing the object model.
- Performance might not be as optimized for real-time tasks as Excel's own scripting capabilities.
Having explored the various steps involved in merging Excel cells with C, you now have the tools at your disposal to automate this process within your workflow. This ability to script Excel through programming not only streamlines your data manipulation tasks but also opens up possibilities for integrating Excel functionalities into more extensive software systems, making your work with spreadsheets more efficient, precise, and scalable.