3 Ways to Make Sheet Active in Excel VBA

Working with VBA in Microsoft Excel can significantly enhance your productivity and efficiency when automating tasks, especially when it comes to managing multiple worksheets. One common task in Excel VBA is activating or selecting sheets, which is essential for executing macro commands on the correct sheet. Here, we'll delve into three methods to make a sheet active in Excel using VBA.
Method 1: Using the ‘Activate’ Method
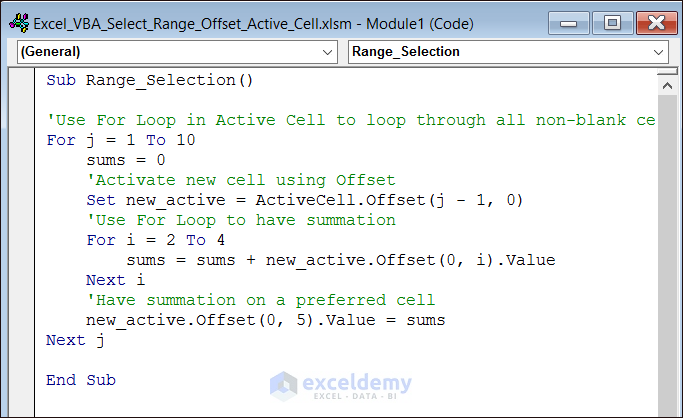

The 'Activate' method in VBA allows you to make a sheet the active sheet. This method can be particularly useful when you need to perform actions on a specific sheet.
Sub MakeSheetActive()
Worksheets("SheetName").Activate
End Sub
📌 Note: Replace "SheetName" with the actual name of the sheet you want to activate.
- User Scenario: You might use this method when you have a user form to jump between different worksheets for data entry or reporting.
- Workflow: Write the sheet name in your code, and when the macro runs, it will switch to that sheet.
Method 2: Using the ‘Select’ Method
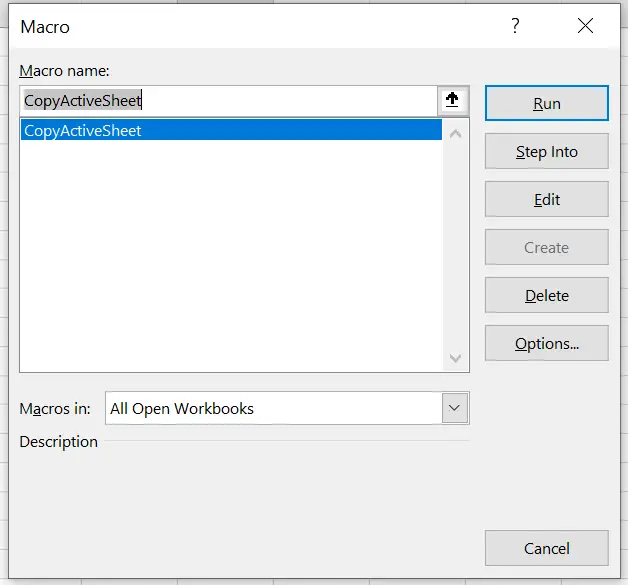

While 'Activate' makes a sheet the active one for actions, 'Select' works similarly but also selects the sheet in addition to activating it. This might be handy when you need to manipulate multiple sheets at once.
Sub SelectSheet()
Sheets("SheetName").Select
End Sub
⚠️ Note: 'Select' will change the active workbook if the sheet is not part of the active workbook, so ensure you're in the correct workbook first.
- Scenario: When performing operations that require multiple sheets to be active simultaneously, like chart creation.
- Approach: The 'Select' method allows you to select more than one sheet, which can be useful for mass operations.
Method 3: Using the ‘Name’ Property


Excel VBA also allows you to change the 'Name' property of a sheet. Although this doesn't directly make a sheet active, it can be part of a workflow where you then activate the sheet with the new name.
Sub ChangeSheetNameAndActivate()
Sheets("OldSheetName").Name = "NewSheetName"
Sheets("NewSheetName").Activate
End Sub
📄 Note: This method can be useful for dynamic sheet management where sheet names are subject to change.
- Application: This can be used in scenarios where sheet names change based on data input or time-based logic.
- Process: Change the sheet name first, then activate it for further manipulation.
Throughout this exploration of VBA methods for activating sheets, we've covered straightforward techniques that make navigation and manipulation of Excel worksheets more efficient. Here's a summary of the key points:
- Activate Method: Directly makes a sheet active for further VBA operations.
- Select Method: Activates and selects a sheet, useful for multi-sheet operations.
- Name Property: Allows dynamic renaming and subsequent activation of sheets, adding flexibility to your VBA scripts.
Remember, activating or selecting sheets should be done cautiously to avoid user confusion or accidental data changes. By understanding these methods, you can tailor your VBA scripts to work more effectively with the sheet structure in Excel, enhancing both your scripts' functionality and your overall productivity.
What is the difference between Activate and Select in Excel VBA?

+
Activate’ makes a sheet the active one where all subsequent actions are performed, whereas ‘Select’ both activates the sheet and selects it in the Excel interface, allowing for simultaneous operations on multiple sheets.
Can I undo the activation of a sheet in VBA?

+
Yes, you can store the current active sheet’s name in a variable before activating another sheet and then reactivate it using that variable. However, this should be done with caution as it can lead to confusion if not managed properly.
Is there a performance difference in using these methods?

+
While the difference in performance is minimal for small scripts, ‘Activate’ and ‘Select’ methods can slow down execution if used excessively in loops or with many sheets. For better performance, consider using sheet references directly where possible.