5 Steps to Convert Excel Sheets into R Data Files

Working with data in R can often require importing datasets from various sources, with Excel spreadsheets being one of the most common. Converting Excel sheets into R data files streamlines this process, allowing for more efficient analysis and data manipulation. This comprehensive guide outlines five straightforward steps to transition from Excel to R, ensuring your data is easily accessible for advanced statistical computing and graphics.
Step 1: Setting Up Your Environment

Before diving into the conversion process, itโs crucial to ensure your R environment is ready:
- Install R and RStudio: RStudio provides an IDE for R, making it easier to manage packages and scripts.
- Install necessary packages:
- openxlsx - for reading and writing Excel files
- readxl - for reading Excel files
# Install packages
install.packages("openxlsx")
install.packages("readxl")
library(openxlsx)
library(readxl)
๐ Note: Ensure you have the latest versions of the packages to avoid compatibility issues.
Step 2: Loading Excel Data into R

Once your environment is set up, you can load the Excel data into R:
- Specify the path to your Excel file. If the file is in your working directory, you can use the file name directly.
- Use either
read.xlsx()
fromopenxlsx
orread_excel()
fromreadxl
:
# Using openxlsx
data <- read.xlsx("yourfile.xlsx", sheet = 1, colNames = TRUE)
# Using readxl
data <- read_excel("yourfile.xlsx", sheet = 1)
๐ ๏ธ Note: If your spreadsheet uses multiple sheets, specify the sheet number or name in the function call.
Step 3: Preprocessing Your Data
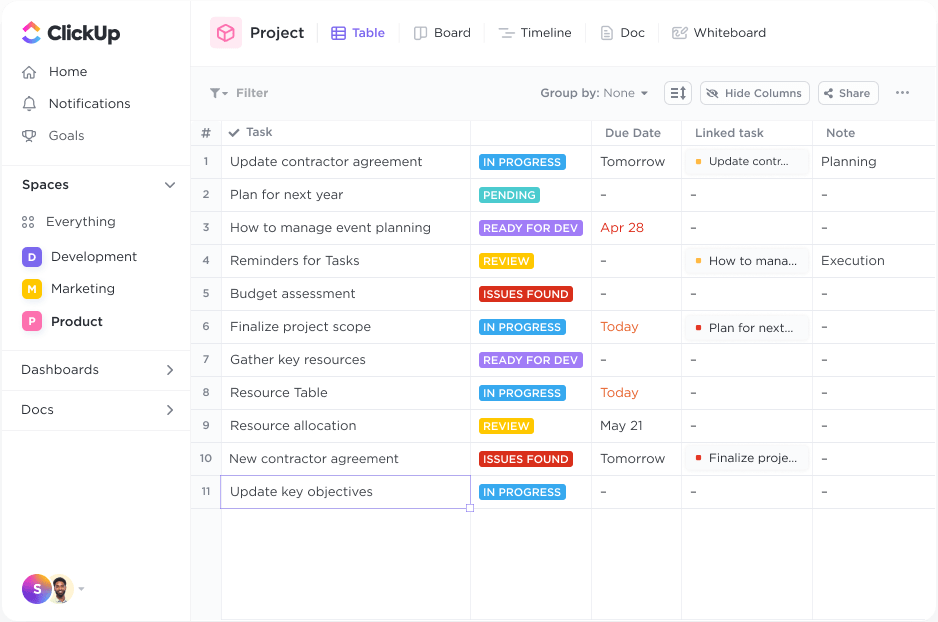
Now that your data is in R, you might need to preprocess it:
- Check and clean data: deal with missing values, ensure correct data types, and more.
# Checking for missing values
summary(is.na(data))
# Removing rows with NAs
data <- na.omit(data)
# Converting date strings to date objects if needed
data$Date <- as.Date(data$Date, format = "%m/%d/%Y")
Step 4: Saving as R Data File

With your data ready, you can now save it in an R-specific format for quick loading in future sessions:
# Save the data as an .rds file
saveRDS(data, "yourdatafile.rds")
# Or save as .RData
save(data, file = "yourdatafile.RData")
๐๏ธ Note: The .rds format is recommended for single objects, while .RData can store multiple R objects.
Step 5: Reloading and Using Your R Data File

To use your converted data in future R sessions:
# To load an .rds file
loaded_data <- readRDS("yourdatafile.rds")
# To load an .RData file
load("yourdatafile.RData")
Having followed these steps, you've successfully converted your Excel spreadsheets into R data files, making it much easier to manage and analyze data within the R ecosystem.
These steps facilitate not only data import but also contribute to better data management practices. By keeping your data in R's native formats, you ensure that you're ready to perform analysis without the constant need to import data, thus enhancing productivity and workflow efficiency in your R-based projects.
Can I convert multiple sheets at once?

+
Yes, you can convert multiple sheets by specifying each sheet in the Excel file or by automating the process with loops in R to handle several sheets in one go.
How do I handle complex data structures from Excel in R?

+
R packages like tidyverse (which includes dplyr for data manipulation) can be used to restructure and clean complex data from Excel spreadsheets.
What are the benefits of using R for data analysis?

+
R is widely used for statistical analysis, data visualization, and machine learning. Itโs free, open-source, and has a vast community supporting its development, which means there are numerous libraries and tools available for almost any data-related task.