5 Ways to Load Excel Sheets into MATLAB Efficiently
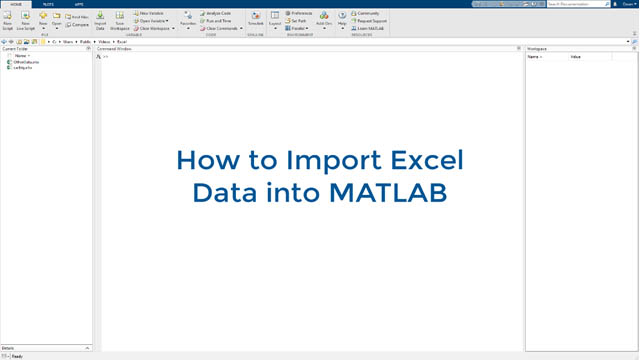
If you're an engineer, data scientist, or researcher, you've likely encountered the need to import Excel spreadsheets into MATLAB. MATLAB's powerful computational tools are excellent for data analysis, but getting data into MATLAB efficiently can be a bottleneck in your workflow. This guide walks you through five effective methods to load Excel sheets into MATLAB, ensuring you can spend more time analyzing data rather than preparing it.
Method 1: Using the Import Tool
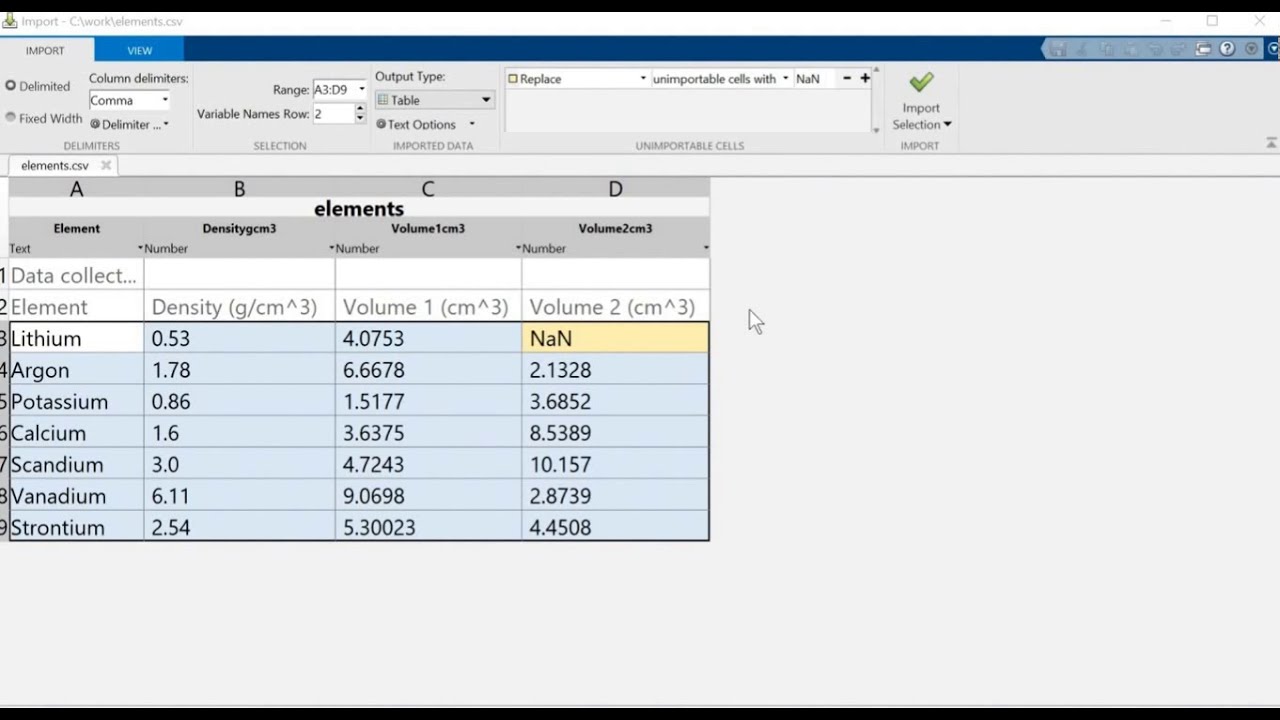
MATLAB’s Import Tool is a user-friendly interface for importing data from files like Excel:
- Open MATLAB and navigate to the Import Data option from the Home tab.
- Select your Excel file. MATLAB will attempt to auto-detect the headers, data types, and ranges.
- Specify the sheet name or number you wish to import, and review the data preview.
- Select the variables to import, setting MATLAB-friendly names for the imported data.
- After importing, the data is automatically structured into MATLAB arrays or tables.
👉 Note: The Import Tool works best for standard Excel files with clear data structures. If your Excel sheet has merged cells or complex structures, you might need a more customized approach.
Method 2: Using Built-in Functions

MATLAB offers functions like readtable
, xlsread
, and readcell
for direct Excel data loading:
- readtable - Imports data into a table object, which maintains variable names and types:
data = readtable(‘filename.xlsx’, ‘Sheet’, ‘SheetName’);
- xlsread - Best for importing into simple numeric arrays:
[numericData, txt] = xlsread(‘filename.xlsx’, ‘SheetName’, ‘A1:B10’);
- readcell - Ideal when your data includes cells, especially non-rectangular or mixed data:
data = readcell(‘filename.xlsx’, ‘Sheet’, ‘SheetName’);
Function | Use Case | Example Syntax |
---|---|---|
readtable | Structured Data | data = readtable(‘filename.xlsx’, ‘Sheet’, ‘Sheet1’); |
xlsread | Numeric Data | [data, ~] = xlsread(‘filename.xlsx’, ‘Sheet1’); |
readcell | Complex Data | data = readcell(‘filename.xlsx’, ‘Sheet’, ‘Sheet1’); |

🚀 Note: Selecting the correct function can significantly reduce data loading time and complexity.
Method 3: Using Database Connection

Another option for large or frequently updated data sets is connecting to a database:
- Import Excel data into a database like MySQL or SQL Server.
- In MATLAB, use the
database
function to establish a connection:
conn = database(‘databasename’, ‘username’, ‘password’);
data = fetch(conn, ‘SELECT * FROM Sheet1’);
close(conn);
💡 Note: This method requires additional setup but excels in scenarios where data is frequently updated or shared.
Method 4: Custom Functions

Custom functions give you the flexibility to address specific data import needs:
- Create a function in MATLAB to automate the data import process:
function importedData = importDataFromExcel(filename, sheetName)
% Your custom logic to read the Excel file and return data in the desired format
end
Method 5: Automation with External Libraries
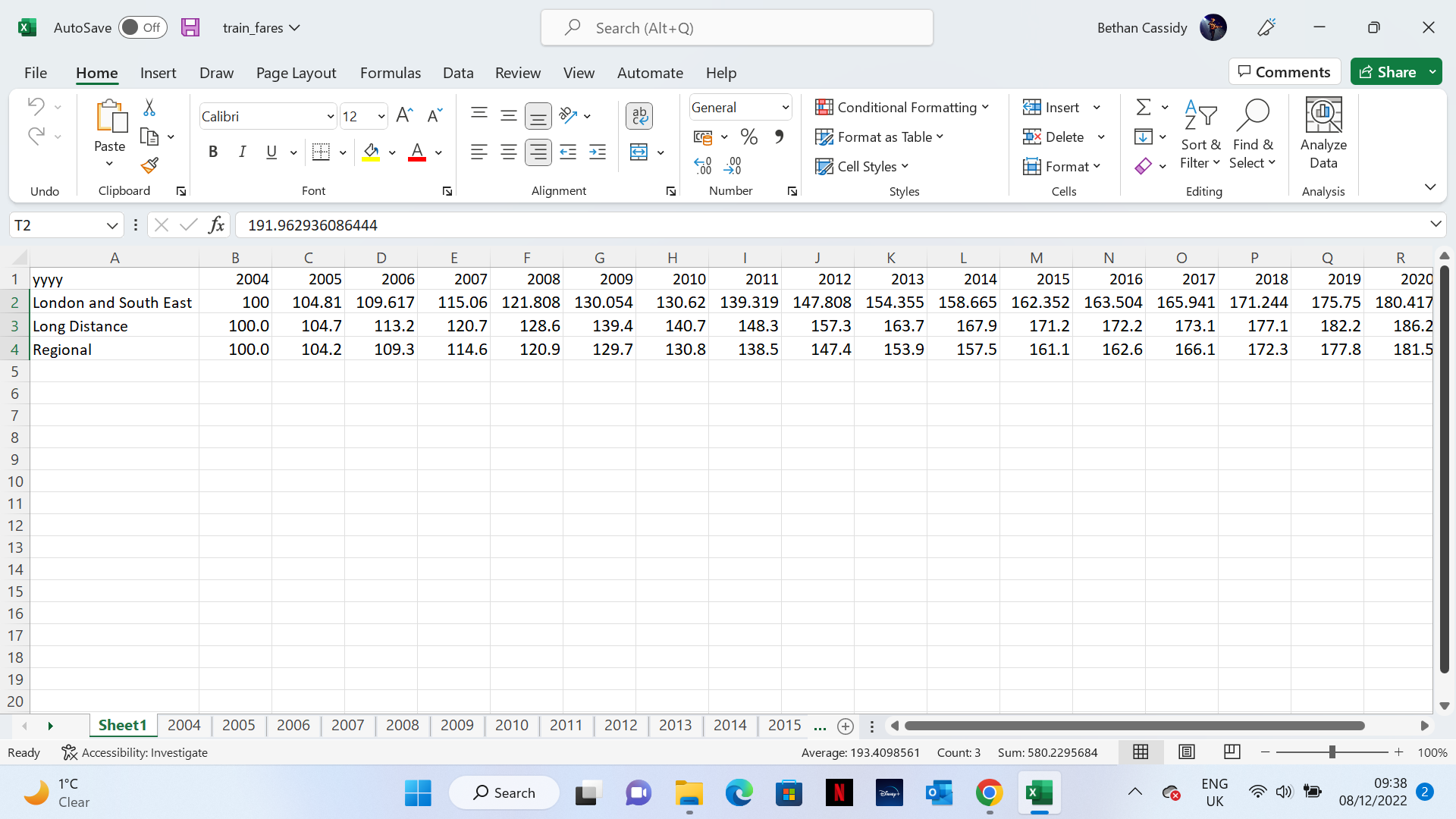
For advanced data manipulation:
- Use libraries like Python’s Pandas to read Excel files, then call Python from MATLAB via the
py
interface:import pandas as pd data = pd.read_excel(‘filename.xlsx’, sheet_name=‘Sheet1’)
matlabData = py.Pandas.read_excel(‘filename.xlsx’, ‘Sheet1’);
- Convert the Python data into MATLAB compatible formats if needed.
💾 Note: External libraries can provide robust solutions but require additional setup and dependencies.
To wrap up, loading Excel data into MATLAB can be streamlined by choosing the right method for your data’s structure and update frequency. Whether using MATLAB’s native tools, databases, custom functions, or external libraries, each method offers advantages tailored to different scenarios. By mastering these techniques, you ensure efficient data loading, enabling you to focus on analysis rather than data preparation.
What is the fastest way to load data from Excel into MATLAB?
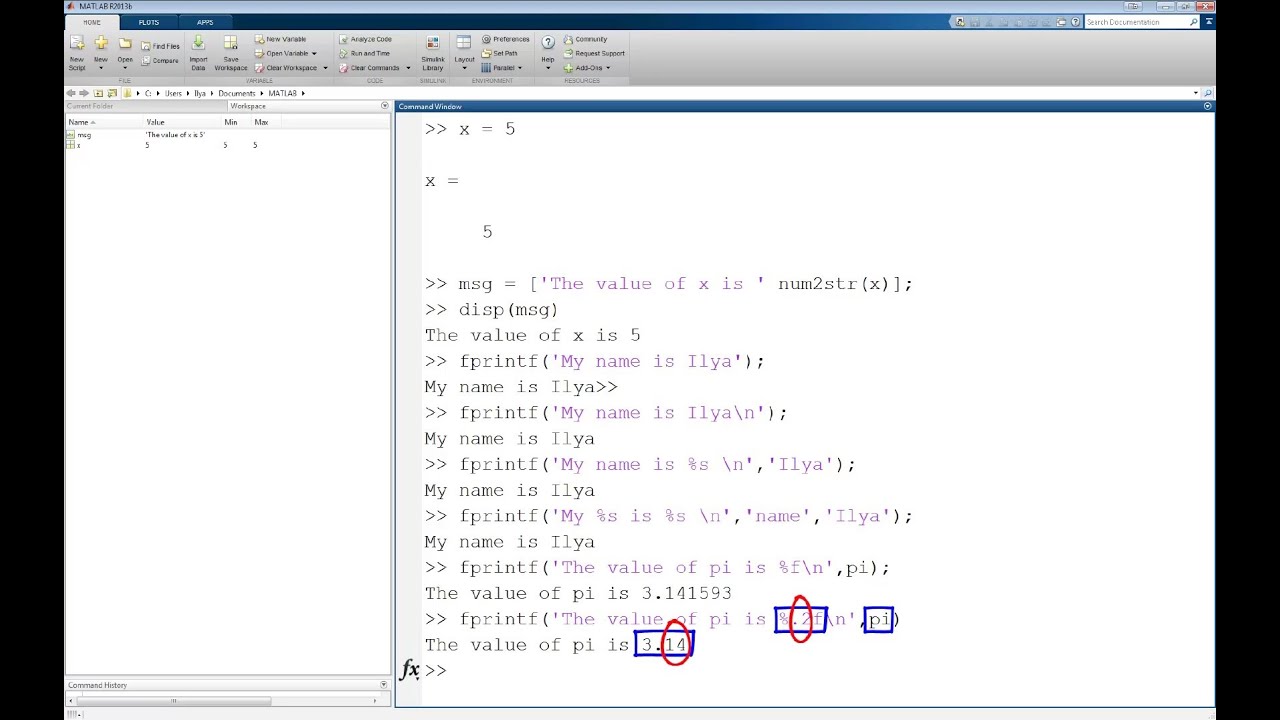
+
The fastest method often depends on the dataset size. For standard Excel files, using readtable
or readcell
can be quick. For larger datasets, consider using database connections or custom functions optimized for your data structure.
How do I handle merged cells in Excel when loading into MATLAB?

+
Excel’s merged cells can complicate data import. Using readcell
or writing a custom function can help maintain data integrity while handling merged cells. For merged cells, ensure your function accounts for these to avoid losing or duplicating data.
Can I update MATLAB data with Excel data automatically?
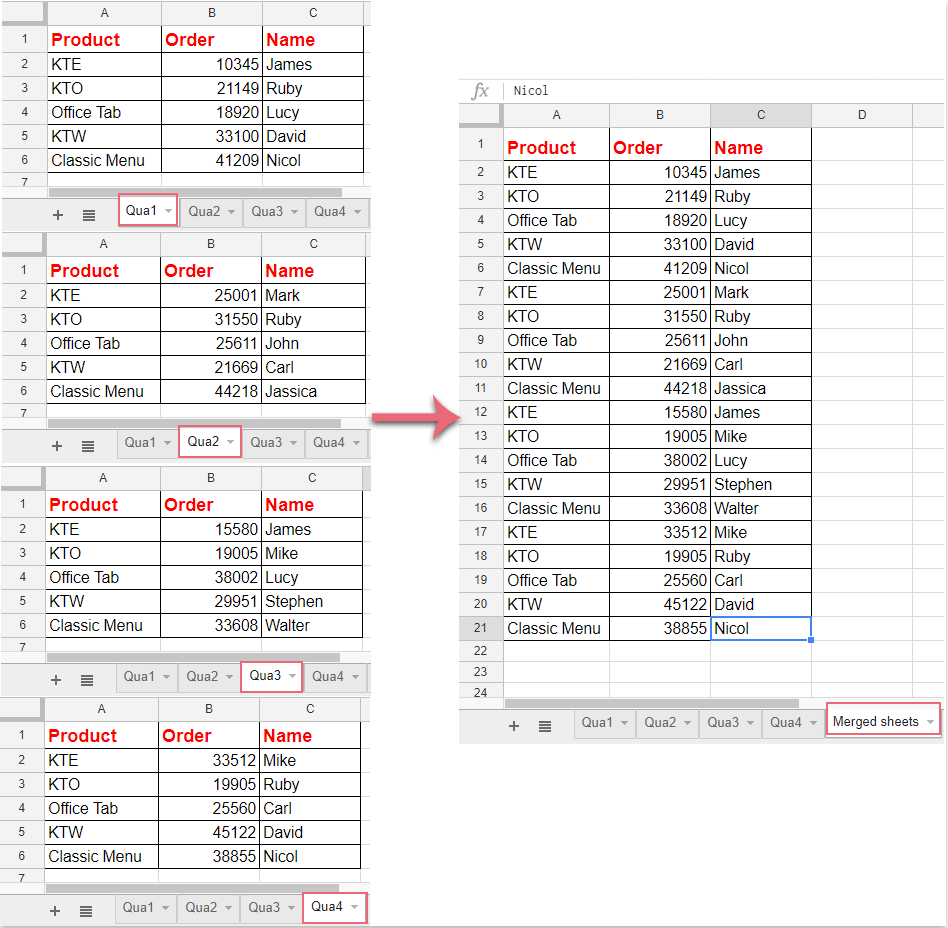
+
Yes, by using database connections or automation scripts. For instance, connect to a database or create a script in MATLAB or Python that monitors Excel file changes and updates MATLAB data accordingly.