5 Ways to Iterate Through Excel Sheets in MATLAB
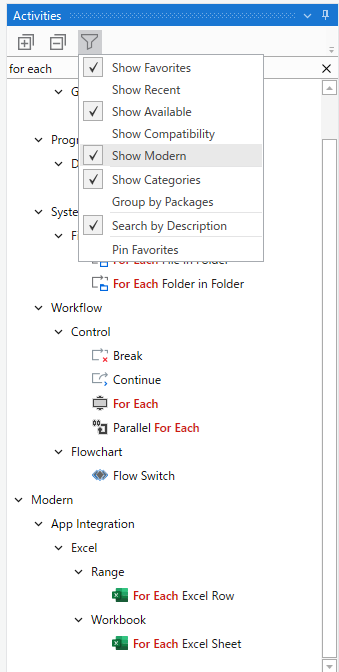
Working with Excel in MATLAB is a common task for data analysts, engineers, and scientists. MATLAB provides robust tools for handling Excel spreadsheets, allowing users to automate data processing and analysis. In this post, we'll explore five effective methods to iterate through Excel sheets using MATLAB. Each method offers different approaches, suited to various scenarios, enhancing your workflow with Excel data manipulation.
Method 1: Using the xlsfinfo
Function

The xlsfinfo
function provides preliminary information about an Excel file, including the number of sheets and their names.
- Step 1: Retrieve sheet information with
xlsfinfo
:[status,sheets,xlFormat] = xlsfinfo(‘your_excel_file.xlsx’);
- Step 2: Loop through the sheets:
for i = 1:length(sheets) % Here you can read or manipulate each sheet [data, text] = xlsread(‘your_excel_file.xlsx’, sheets{i}); % Further processing on the data or text end
📌 Note: The `xlsfinfo` function only retrieves basic information, not the actual data. You must use `xlsread` or another function to access data.
Method 2: Utilizing ActiveX

ActiveX can interact with Excel as an automation server, allowing for dynamic sheet interaction:
- Step 1: Open Excel and workbook:
Excel = actxserver(‘Excel.Application’); Workbook = Excel.Workbooks.Open(‘your_excel_file.xlsx’);
- Step 2: Iterate through sheets:
for i = 1:Workbook.Sheets.Count Worksheet = Workbook.Sheets.Item(i); % You can manipulate or read the worksheet here end
🔔 Note: This method requires Excel to be installed on your machine.
Method 3: Leveraging Database Toolbox

If you have the Database Toolbox installed, you can query Excel data like a database:
- Step 1: Set up the database connection:
conn = database(“,”, ‘driver={Microsoft Excel Driver (*.xls, *.xlsx, *.xlsm, *.xlsb)};DBQ=your_excel_file.xlsx’);
- Step 2: Execute SQL-like queries on each sheet:
sheets = {sheetname1, sheetname2, sheetname3}; for i = 1:length(sheets) query = [‘SELECT * FROM [’, sheets{i},‘$]’]; data = fetch(conn, query); % Process your data end
Method 4: Using xlsread
with Range

xlsread
can be used to read specific ranges from sheets, providing flexibility for larger datasets:
- Step 1: Determine the number of sheets:
[num,txt,raw] = xlsread(‘your_excel_file.xlsx’, 1, “); % First sheet numSheets = size(get(getappdata(0, ‘Excel’), ‘Sheets’), 1);
- Step 2: Loop through sheets with specified ranges:
for sheetNum = 1:numSheets [num,txt,raw] = xlsread(‘your_excel_file.xlsx’, sheetNum, ‘A1:G50’); % Adjust range as needed % Further data processing or analysis end
Method 5: Batch Reading with dir
and xlsread

This method is useful when you have multiple Excel files in a directory to process:
- Step 1: Get all Excel files in the directory:
files = dir(‘*.xlsx’);
- Step 2: Process each file:
for i = 1:length(files) filename = files(i).name; [num, txt, raw] = xlsread(filename); % Iterate through sheets within each file if needed end
Each of these methods offers different levels of interaction with Excel files in MATLAB, allowing you to choose the one that best fits your task:
- Basic Information: Use `xlsfinfo` for a high-level overview.
- Dynamic Interactivity: ActiveX offers the most control for interacting with Excel.
- Database-Like Operations: The Database Toolbox provides SQL-like capabilities.
- Range Reading: `xlsread` with range specifiers for targeted data extraction.
- Batch Processing: Handling multiple files or sheets in a folder.
Remember, choosing the right method depends on your specific needs, the complexity of your data, and the control you need over Excel sheets. MATLAB's versatility in dealing with spreadsheets makes it an invaluable tool for data analysts and anyone dealing with large datasets stored in Excel. Whether you're automating data entry, analysis, or exporting results back to Excel, MATLAB offers a range of techniques to optimize your workflow.
Can I write to Excel files using MATLAB?

+
Yes, you can write data to Excel files using MATLAB. Functions like xlswrite
or the ActiveX
server can be used to create, modify, and save Excel spreadsheets.
How do I know if Excel is installed to use ActiveX?

+
Use the ver
command in MATLAB to list installed toolboxes and check for Microsoft Excel.
Is it possible to perform conditional processing on each sheet?

+
Yes, within the loops provided in the methods, you can set conditions to process or manipulate data based on specific criteria, such as the sheet name or content.