5 Ways to Import Excel Sheets in Python

Importing Excel Sheets with openpyxl

The openpyxl library is a robust tool for handling Excel files in Python. It's particularly useful for reading, writing, and modifying Excel 2010 xlsx/xlsm/xltx/xltm files without the need for Microsoft Excel.
- Install openpyxl using pip:
pip install openpyxl
To import an Excel sheet:
- Load the workbook:
from openpyxl import load_workbook
workbook = load_workbook('example.xlsx')
- Select the active sheet or specify the sheet by name:
sheet = workbook.active
- or
sheet = workbook['Sheet1']
- Iterate over rows and columns:
for row in sheet.iter_rows(min_row=1, max_row=1, values_only=True):
for cell in row:
print(cell.value)
💡 Note: While openpyxl is quite efficient for handling smaller files, it might not be the best choice for very large Excel files due to its memory consumption.
Using pandas to Read Excel Files
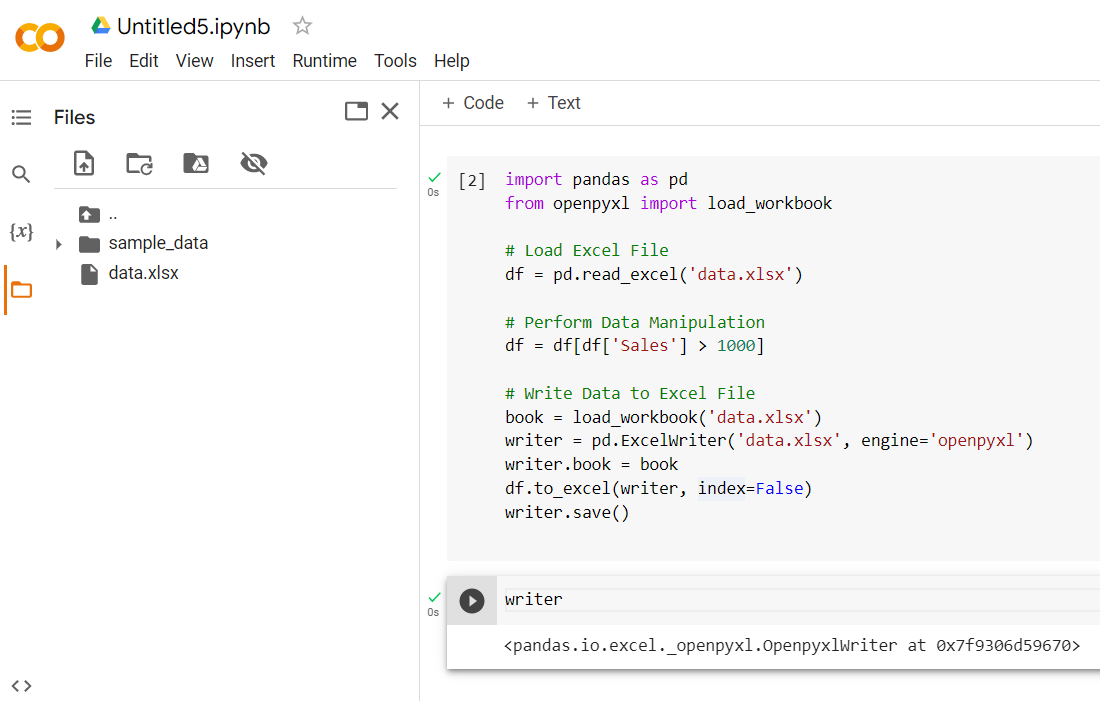
Pandas, known for its data manipulation capabilities, also offers straightforward methods for importing Excel files.
- Install pandas:
pip install pandas
- Import pandas and read the Excel file:
import pandas as pd
data = pd.read_excel('example.xlsx', sheet_name='Sheet1')
- Access and manipulate data:
print(data.head())
- View the first few rows.print(data['Column Name'])
- Access specific columns.
Pandas reads the entire Excel sheet into a DataFrame, which can be further manipulated using pandas functions.
🧐 Note: Make sure the Excel file has a simple structure. Pandas can handle headers and different data types but might struggle with complex formatting.
Handling Large Excel Files with xlsx2csv

When dealing with very large Excel files, converting them to CSV can save memory and computation time.
- Install xlsx2csv:
pip install xlsx2csv
- Convert Excel to CSV:
from xlsx2csv import Xlsx2csv
Xlsx2csv('example.xlsx', 'output.csv').convert()
- Now you can easily read the CSV file with pandas:
data = pd.read_csv('output.csv')
🌟 Note: This method helps in efficiently handling large datasets, but it loses the original formatting of the Excel sheet.
Reading Excel with xlrd

xlrd is an older library but still widely used for reading Excel files, especially for legacy applications.
- Install xlrd:
pip install xlrd
- Import and use xlrd:
import xlrd
workbook = xlrd.open_workbook('example.xlsx')
sheet = workbook.sheet_by_index(0)
for row in range(sheet.nrows):
print(sheet.row_values(row))
xlrd allows you to read data, cell formats, and even formulas, but it has limitations with newer Excel formats.
Advanced Data Extraction with pyexcel

pyexcel is a library that simplifies working with multiple spreadsheet formats by abstracting the underlying libraries like openpyxl and xlrd.
- Install pyexcel with the necessary backends:
pip install pyexcel pyexcel-xls pyexcel-xlsx
- Reading an Excel file:
import pyexcel as pe
data = pe.get_book(file_name='example.xlsx')
sheet_data = data['Sheet1']
pyexcel can automatically detect the file format, making it versatile for handling different types of spreadsheets.
🔍 Note: While convenient, pyexcel might add a slight performance overhead due to its abstract nature, so consider the file size and complexity.
To wrap up, let's reflect on the various methods for importing Excel sheets into Python:
- openpyxl is great for reading, writing, and modifying recent Excel formats.
- pandas offers a high-level interface for data manipulation after importing Excel data.
- xlsx2csv can be particularly useful when dealing with large datasets for memory efficiency.
- xlrd provides basic functionalities for older Excel files.
- pyexcel simplifies operations across different spreadsheet formats.
Choosing the right tool depends on your specific needs, like handling large files, the necessity for specific Excel features, or ease of data manipulation. Each of these libraries has its strengths, and often, combining them can offer a robust workflow for any Excel-related tasks in Python.
Can I modify and save changes back to Excel with any of these libraries?

+
Yes, both openpyxl and pandas (with openpyxl as an engine) can save changes back to Excel files. However, for simple read-only operations, pandas might be sufficient.
Is there a limit on the size of the Excel file I can import?

+
The limit depends on available memory and processing power. For very large files, consider using xlsx2csv to convert to CSV first.
What if my Excel file uses macros or is password-protected?

+
openpyxl does not support macros or encrypted files out of the box. For password-protected files, you might need specialized libraries or manual decryption.
Are these libraries compatible with all versions of Excel?

+
The compatibility varies. openpyxl supports .xlsx, .xlsm, and some other formats starting from Excel 2007. xlrd supports up to Excel 2003 file formats, with limited support for newer formats.
Do I need Microsoft Excel installed on my machine to use these libraries?

+
No, all these libraries work independently of Microsoft Excel. They are pure Python solutions for handling Excel files.