5 Ways to Access Excel's First Sheet in C
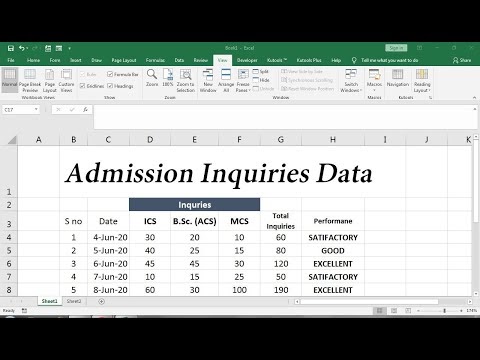
Understanding Excel File Structure

In the world of data handling, Microsoft Excel remains a widely used tool, especially in business environments. Excel files, which use the .xlsx format, are organized into workbooks, sheets, and cells. Understanding how to interact with these components programmatically can vastly improve efficiency in data manipulation tasks.
A workbook contains one or more sheets, which can be Excel worksheets, charts, or other types of sheets like dialog sheets or macro sheets. Within Excel, the first sheet you see upon opening a file is significant for many operations:
- It's often used for summary data or as an entry point for navigating the workbook.
- Data analysts might use this sheet for instructions, overview, or frequently accessed data.
- Developers might need to access this sheet to prepare the workbook for subsequent data processing or to extract key information quickly.
Why Access the First Sheet?

Accessing the first sheet programmatically in Excel through C# provides several benefits:
- Automating Initial Setup: Automate tasks like setting up data validation rules or formatting specific cells on the first sheet.
- Data Retrieval: Quickly retrieve key data or perform initial analysis from the first sheet.
- Sheet Validation: Ensure the structure of the first sheet meets certain criteria before further processing or report generation.
- Performance Optimization: By starting with the first sheet, operations can be optimized, especially when dealing with large workbooks.
Method 1: Using EPPlus Library

EPPlus is an open-source library for reading and writing Excel files using .NET framework. Here’s how to access the first sheet with EPPlus:
using OfficeOpenXml;
public void AccessFirstSheet(string filePath)
{
using (var package = new ExcelPackage(new FileInfo(filePath)))
{
// Access the first sheet
var worksheet = package.Workbook.Worksheets.First();
// Example: Print sheet name
Console.WriteLine("First Sheet: " + worksheet.Name);
}
}
Pros:
- Lightweight and fast.
- Supports most Excel features.
Cons:
- Does not support all Excel file formats; primarily focuses on .xlsx.
- Not suitable for editing very large files due to memory constraints.
💡 Note: EPPlus works with Office 365 Excel but has limitations with some newer features introduced after its last major update.
Method 2: Microsoft.Office.Interop.Excel

This method involves interacting with Excel through COM Interop, which provides access to the Excel application object:
using Excel = Microsoft.Office.Interop.Excel;
public void AccessFirstSheet(string filePath)
{
Excel.Application excelApp = new Excel.Application();
Excel.Workbook workbook = excelApp.Workbooks.Open(filePath);
Excel.Worksheet worksheet = workbook.Worksheets[1];
// Example: Print sheet name
Console.WriteLine("First Sheet: " + worksheet.Name);
workbook.Close();
excelApp.Quit();
}
Pros:
- Provides comprehensive control over Excel's features and automation capabilities.
- Can handle all Excel file formats.
Cons:
- Performance can be slow for large datasets due to COM interop.
- Requires Excel to be installed on the server.
- Memory management can be an issue if not handled properly.
⚠️ Note: Ensure proper cleanup to avoid "zombie" Excel processes lingering on the server.
Method 3: Open XML SDK

The Open XML SDK allows direct manipulation of the XML structure of Excel files without needing Excel installed:
using DocumentFormat.OpenXml.Packaging;
using DocumentFormat.OpenXml.Spreadsheet;
public void AccessFirstSheet(string filePath)
{
using (SpreadsheetDocument document = SpreadsheetDocument.Open(filePath, false))
{
WorkbookPart workbookPart = document.WorkbookPart;
WorksheetPart worksheetPart = workbookPart.WorksheetParts.First();
Worksheet worksheet = worksheetPart.Worksheet;
// Example: Print sheet name
var sheetName = workbookPart.Workbook.Descendants<Sheet>().First().Name;
Console.WriteLine("First Sheet: " + sheetName);
}
}
Pros:
- Excellent for high-performance applications.
- No dependency on Excel.
Cons:
- Complex for beginners, as it deals with XML structure directly.
- Doesn't handle Excel file features as directly as COM Interop or EPPlus.
Method 4: ExcelDataReader

ExcelDataReader offers a lightweight library for reading Excel files, especially useful for large datasets:
using ExcelDataReader;
public void AccessFirstSheet(string filePath)
{
using (var stream = File.Open(filePath, FileMode.Open, FileAccess.Read))
{
using (var reader = ExcelReaderFactory.CreateReader(stream))
{
// Get the first worksheet
var result = reader.AsDataSet(new ExcelDataSetConfiguration
{
ConfigureDataTable = (_) => new ExcelDataTableConfiguration
{
UseHeaderRow = false
}
});
var firstSheetName = result.Tables[0].TableName;
Console.WriteLine("First Sheet: " + firstSheetName);
}
}
}
Pros:
- Efficient for large datasets.
- Can read both .xls and .xlsx formats.
Cons:
- Limited capabilities for writing back to Excel.
- Doesn't support all Excel features out of the box.
Method 5: Custom Code with SpreadsheetGear

SpreadsheetGear offers a more advanced, high-performance alternative for Excel file manipulation:
using SpreadsheetGear;
public void AccessFirstSheet(string filePath)
{
using (IWorkbook workbook = Factory.GetWorkbook(filePath))
{
IWorksheet worksheet = workbook.Worksheets[0];
// Example: Print sheet name
Console.WriteLine("First Sheet: " + worksheet.Name);
}
}
Pros:
- High performance, suitable for large datasets.
- Can generate reports, manipulate data, and create charts.
Cons:
- Requires a license for commercial use.
- Not as straightforward as some other libraries.
To sum up our exploration, accessing Excel's first sheet in C# can be achieved through various libraries, each offering unique strengths and trade-offs. Whether you opt for the simplicity of EPPlus, the comprehensive control of Microsoft.Office.Interop.Excel, the XML-centric approach of Open XML SDK, the efficiency of ExcelDataReader, or the advanced features of SpreadsheetGear, your choice will largely depend on your project's needs, file size considerations, and your familiarity with each library. With these methods, you can automate Excel tasks, streamline data operations, and significantly enhance productivity. Understanding these tools empowers developers to manipulate Excel files programmatically, bridging the gap between raw data and meaningful insights.
Which library is best for beginners?

+
EPPlus or ExcelDataReader would be good starting points for beginners due to their ease of use and relatively simple APIs.
Can I use these libraries on a machine without Excel installed?

+
Yes, EPPlus, ExcelDataReader, Open XML SDK, and SpreadsheetGear do not require Excel to be installed to manipulate .xlsx files.
Are there performance concerns with any of these methods?

+
Yes, Microsoft.Office.Interop.Excel can be slow for large datasets. EPPlus, Open XML SDK, and SpreadsheetGear are generally more efficient for performance-critical applications.
How do I ensure that memory is properly managed when using these libraries?

+
Use the ‘using’ keyword for disposable objects to ensure they are properly closed and resources are released. Also, for libraries like ExcelDataReader, ensure large datasets are streamed rather than loaded entirely into memory.
Can these methods handle password-protected Excel files?

+
Handling password-protected files varies by library. Open XML SDK and Microsoft.Office.Interop.Excel can open password-protected files if the password is provided, while EPPlus does not support this natively.