5 Ways to Extract Excel Data for Selenium TestNG

Automating the process of data extraction from Excel into Selenium TestNG frameworks is an invaluable skill for efficient test script management and maintenance. The integration of Excel spreadsheets, known for their ease of use and data organization capabilities, with the automation prowess of Selenium, forms a potent combination for agile software testing. This blog post will detail five methodologies to perform this seamless data integration, each method catering to varying levels of technical familiarity and requirements.
Method 1: Apache POI

Apache POI, an intuitive library for working with Microsoft documents in Java, allows for robust Excel file manipulation. Here’s how to integrate it with Selenium TestNG:
- Download and include the POI library in your project’s build path.
- Use
XSSFWorkbook
for newer Excel formats (.xlsx) orHSSFWorkbook
for older ones (.xls). - Read data from cells and utilize it within TestNG test cases for parameterization.
// Pseudo code for reading data from an Excel file using POI
FileInputStream excelFile = new FileInputStream("yourpath/excelfile.xlsx");
XSSFWorkbook workbook = new XSSFWorkbook(excelFile);
XSSFSheet sheet = workbook.getSheetAt(0);
Row row = sheet.getRow(rowNum);
Cell cell = row.getCell(cellNum);
String cellValue = cell.getStringCellValue();
// Your logic to use cellValue in test case
💡 Note: While Apache POI is versatile, managing large datasets can consume significant system resources.
Method 2: Using JDBC-ODBC Bridge

The JDBC-ODBC bridge provides a way to connect to Excel files as if they were databases:
- Set up an ODBC data source in your Windows OS for your Excel file.
- Use JDBC to query this ODBC source and retrieve the data directly into your tests.
// Sample code to connect to an Excel file via JDBC-ODBC
Class.forName("sun.jdbc.odbc.JdbcOdbcDriver");
Connection conn = DriverManager.getConnection("jdbc:odbc:Driver={Microsoft Excel Driver (*.xls, *.xlsx, *.xlsm, *.xlsb)};DBQ=yourpath/excelfile.xlsx;");
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery("SELECT * FROM [Sheet1$]");
// Iterate through the result set to read data
while (rs.next()) {
String yourdata = rs.getString("YourColumn");
// Use yourdata in your test case
}
💡 Note: The JDBC-ODBC approach can be tricky to set up but offers a familiar SQL-like interface for data access.
Method 3: JExcelApi

JExcelApi is another library for working with Excel files, providing an alternative to Apache POI:
- Download and add the JExcelApi library to your project.
- Interact with Excel sheets and cells to read data or update them.
// Pseudocode for using JExcelApi to read Excel data
Workbook workbook = Workbook.getWorkbook(new File("yourpath/excelfile.xls"));
Sheet sheet = workbook.getSheet(0);
Cell cell = sheet.getCell(colNum, rowNum);
String contents = cell.getContents();
// Use the contents variable in your test case
💡 Note: JExcelApi is lighter than POI but only supports .xls files, not the newer .xlsx format.
Method 4: Using a Database

Storing Excel data in a database and then accessing it through JDBC in Selenium:
- Import Excel data into your chosen database (e.g., MySQL).
- Connect to the database from your Selenium TestNG tests using JDBC.
- Retrieve test data for your automation scripts.
// Example JDBC connection to a MySQL database
Connection con = DriverManager.getConnection("jdbc:mysql://localhost/testdb", "username", "password");
Statement stmt = con.createStatement();
ResultSet rs = stmt.executeQuery("SELECT * FROM test_data");
while (rs.next()) {
String data = rs.getString("data_column");
// Use this data within your tests
}
💡 Note: This method requires setting up and maintaining a separate database but offers advantages for scaling data access.
Method 5: JSON Configuration Files

Using Excel as a source, you can export the data to JSON files and read them:
- Convert Excel data into JSON format.
- Use libraries like Gson to read JSON files in Selenium.
// Sample code for reading JSON data into Selenium
import com.google.gson.JsonObject;
import com.google.gson.JsonParser;
String jsonInput = readFromFile("yourpath/data.json");
JsonObject jobj = new JsonParser().parse(jsonInput).getAsJsonObject();
String value = jobj.get("yourkey").getAsString();
// Utilize value in test cases
💡 Note: JSON files provide an alternative, less resource-intensive option but might not be as maintainable for large datasets.
To summarize, there are multiple ways to extract data from Excel for Selenium TestNG automation. Each method comes with its own advantages:
- Apache POI for detailed Excel manipulation.
- JDBC-ODBC Bridge for SQL-like data access.
- JExcelApi for simpler use with .xls files.
- Using databases for data storage and retrieval.
- Exporting to JSON for lighter file operations.
The choice depends on project requirements, team expertise, and the specific use case for data management within automated testing.
Which method is best for reading large datasets?

+
For handling large datasets, Apache POI and JDBC-ODBC bridge might face memory constraints. Opting for a database solution or JSON configuration could be more efficient, as they can handle bulk data operations with less memory overhead.
Can I update Excel sheets during automation?

+
Yes, libraries like Apache POI or JExcelApi allow both reading from and writing to Excel files. This can be particularly useful for logging test results back to the Excel file.
What are the advantages of using JSON over Excel?
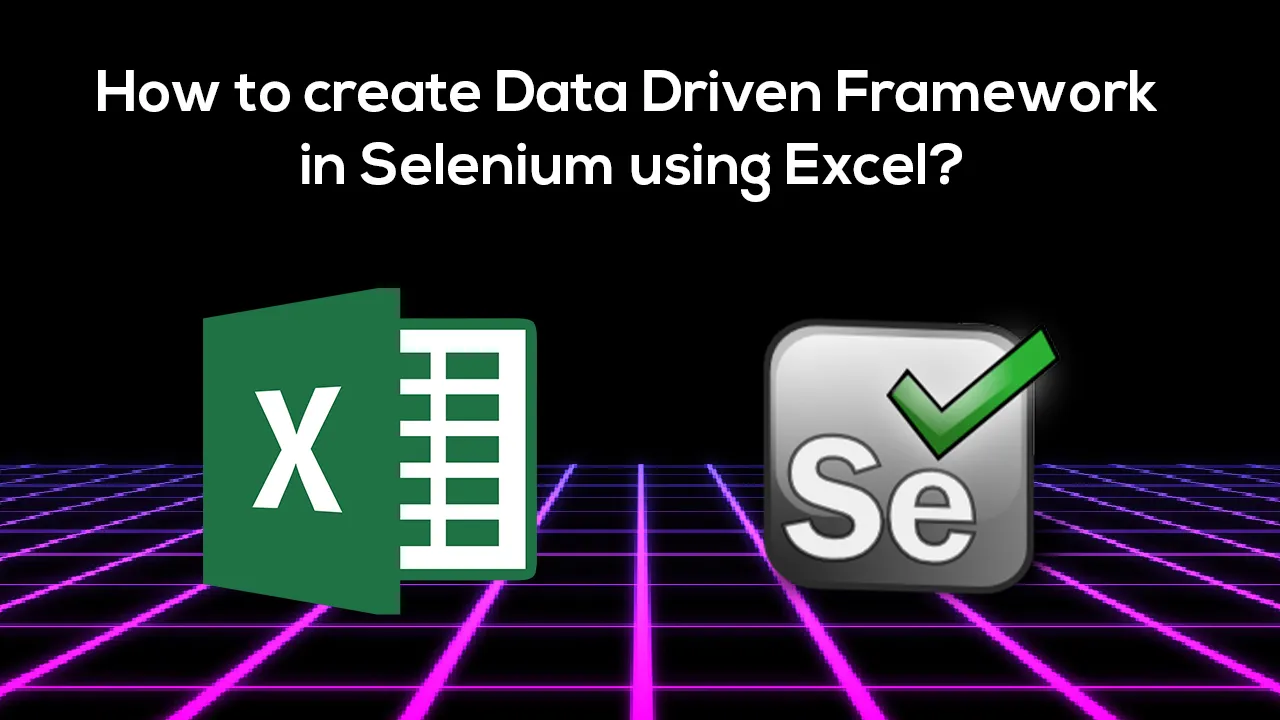
+
JSON is lightweight, easy to read/write with modern languages, and can be shared across different applications more seamlessly. It’s particularly suitable when you need to pass data in web services or API testing scenarios.