5 Ways to Extract Excel Data in JavaScript

In today's data-driven world, integrating Excel data into web applications has become increasingly important. JavaScript, being the language of the web, offers multiple avenues to achieve this. Here are five ways you can extract data from Excel files using JavaScript:
1. Using XLSX Library

One of the most popular methods for dealing with Excel files is by using the XLSX library. Here’s how you can leverage this tool:
- Install the Library: First, you need to include or install the XLSX library. You can do this through npm by running:
- Read the File: Use the following code to read an Excel file:
npm install xlsx
import * as XLSX from ‘xlsx’;
const file = './path_to_your_file.xlsx';
const workbook = XLSX.readFile(file);
const sheetName = workbook.SheetNames[0]; // Assuming you want the first sheet
const worksheet = workbook.Sheets[sheetName];
const jsonData = XLSX.utils.sheet_to_json(worksheet);
console.log(jsonData);
</code>
</pre>
<p class="pro-note">🔍 Note: Ensure the Excel file is in a supported format like .xlsx or .xls.</p>
2. Browser’s File API
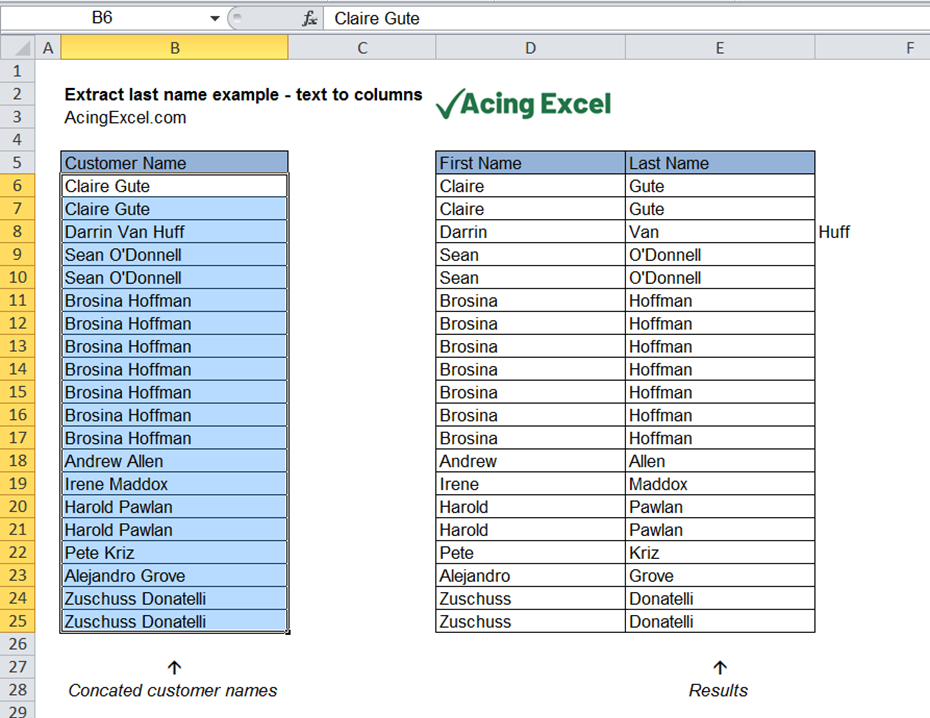
If your application allows users to upload Excel files directly, you can use the FileReader API to read the file:
- HTML Input: Add an input field for file upload:
JavaScript Function: Define the function to handle file reading:
function handleFile(event) {
const file = event.target.files[0];
const reader = new FileReader();
reader.onload = function(e) {
const data = new Uint8Array(e.target.result);
const workbook = XLSX.read(data, {type: ‘array’});
const firstSheetName = workbook.SheetNames[0];
const worksheet = workbook.Sheets[firstSheetName];
const jsonData = XLSX.utils.sheet_to_json(worksheet);
console.log(jsonData);
};
reader.readAsArrayBuffer(file);
}
💡 Note: This method requires user interaction to select and upload a file.
3. Using Papa Parse with ExceltoCSV

Papa Parse is renowned for its CSV parsing capabilities, but combined with a conversion step, you can extract data from Excel:
- Install Dependencies: Install Papa Parse and a tool to convert Excel to CSV like ‘exceljs’:
npm install papaparse exceljs
- Convert Excel to CSV: Use ExcelJS to convert the file:
const Excel = require(‘exceljs’);
const workbook = new Excel.Workbook();
const file = ‘./path_to_file.xlsx’;
workbook.xlsx.readFile(file).then(() => {
const worksheet = workbook.getWorksheet(1);
const data = worksheet.getSheetValues();
const csv = Papa.unparse(data);
console.log(csv);
});
🔗 Note: Remember that Papa Parse is not primarily designed for Excel files, but works when you convert Excel to CSV first.
4. Backend Solution with REST API

For scalability and server-side processing, you can implement a backend API to handle Excel file data:
- Server Side: Use Node.js with a library like ‘node-xlsx’:
npm install node-xlsx
- API Endpoint:
const express = require(‘express’);
const app = express();
const xlsx = require(‘node-xlsx’);
app.post('/upload', (req, res) => {
const excelFile = req.files.excelFile;
const workSheetsFromFile = xlsx.parse(excelFile.data);
const data = workSheetsFromFile[0].data;
res.json({data: data});
});
</code>
</pre>
<li><strong>Client Side</strong>: Make an AJAX call to send the file to the server:</li>
<pre>
<code>
function uploadExcel() {
let formData = new FormData();
formData.append('excelFile', document.getElementById('fileInput').files[0]);
fetch('/upload', {
method: 'POST',
body: formData
}).then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
}
</code>
</pre>
<p class="pro-note">🔐 Note: Ensure you handle file uploads securely on the server side, especially if dealing with public-facing applications.</p>
5. Google Sheets API

If your Excel data is actually in Google Sheets, you can utilize the Google Sheets API to extract data:
- Enable Google Sheets API: Create a project in Google Developers Console, enable the Sheets API, and set up OAuth credentials.
- JavaScript Code:
function listMajorDimension() {
const range = ‘Sheet1!A1:B’; // Define the range
gapi.client.sheets.spreadsheets.values.get({
spreadsheetId: ‘YOUR_SPREADSHEET_ID’,
range: range,
}).then((response) => {
let result = response.result;
console.log(result.values);
});
}
📋 Note: This method requires setup with Google Cloud Platform to enable access to your Google Sheets.
Each of these methods has its unique advantages and considerations:
- XLSX Library provides a straightforward way to work with Excel files locally or in the browser.
- The Browser's File API enables direct user interaction for file uploads.
- Papa Parse with ExceltoCSV offers an alternative for Excel-CSV conversion with the powerful CSV parsing capabilities of Papa Parse.
- Backend API solutions can handle larger files and improve server performance.
- Google Sheets API extends functionality to cloud-based spreadsheets, providing seamless integration with web applications.
In wrapping up, JavaScript offers multiple robust options for extracting data from Excel files, each tailored to different needs and scenarios. Choosing the right method depends on factors like file size, whether the data is client or server-based, and how you wish to interact with it. Understanding these methods allows you to select the most effective tool for integrating Excel data into your web projects, enhancing both user experience and system efficiency.
How can I convert an Excel file to JSON?

+
You can use libraries like XLSX to convert an Excel file to JSON. The process involves reading the file and then using the sheet_to_json method to convert the worksheet data into a JSON array.
What are the limitations of using the XLSX library?

+
The XLSX library has limitations with file size (browser memory constraints) and with certain complex Excel features like formulas, charts, or macros.
Can I use JavaScript to modify an Excel file?

+
Yes, with libraries like XLSX, you can modify Excel files by manipulating the worksheet data, although browser memory constraints can limit the size of the files you can handle.