5 Ways to Find Sheet Names in Excel Scripts

Many Excel users find themselves in a situation where they need to automate tasks involving multiple sheets in a workbook. Identifying and manipulating sheet names programmatically is often essential for these tasks. Excel provides several methods for finding sheet names through VBA (Visual Basic for Applications) scripts. This comprehensive guide delves into five different ways to achieve this, ensuring that you have a versatile set of tools at your disposal for different scenarios.
VBA Code to Loop Through All Sheets

One of the simplest and most direct methods to find sheet names involves looping through all the sheets in a workbook:
Sub ListSheetNames()
Dim ws As Worksheet
Dim sheetNames As String
For Each ws In ThisWorkbook.Worksheets
sheetNames = sheetNames & ws.Name & vbNewLine
Next ws
MsgBox sheetNames, vbInformation, "Sheet Names"
End Sub
This script will loop through each worksheet and concatenate their names into a single string, which is then displayed via a message box. Here's how to use it:
- Open the Visual Basic Editor in Excel.
- Insert a new module and paste the above code.
- Run the subroutine
ListSheetNames
.
Retrieve Sheet Names into an Array

If you require the sheet names in an array for further processing, here's how you can do it:
Sub SheetNamesToArray()
Dim sheets() As String
Dim i As Integer
ReDim sheets(1 To ThisWorkbook.Worksheets.Count)
For i = 1 To ThisWorkbook.Worksheets.Count
sheets(i) = ThisWorkbook.Worksheets(i).Name
Next i
' Print names to immediate window for debugging
For i = LBound(sheets) To UBound(sheets)
Debug.Print sheets(i)
Next i
End Sub
This script populates an array with sheet names:
- It first defines the array with the same size as the number of sheets.
- Then, it fills this array with each sheet's name.
Using the Worksheet Objects Directly

For immediate access to a specific sheet, you can directly use worksheet objects:
Sub FindSpecificSheet()
Dim ws As Worksheet
Set ws = ThisWorkbook.Sheets("Sheet1")
If Not ws Is Nothing Then
MsgBox "Sheet found: " & ws.Name, vbInformation
Else
MsgBox "Sheet not found", vbExclamation
End If
End Sub
Here, the script tries to reference a sheet by name. If it exists, a message box confirms its existence.
Iterating Over Only Visible Sheets
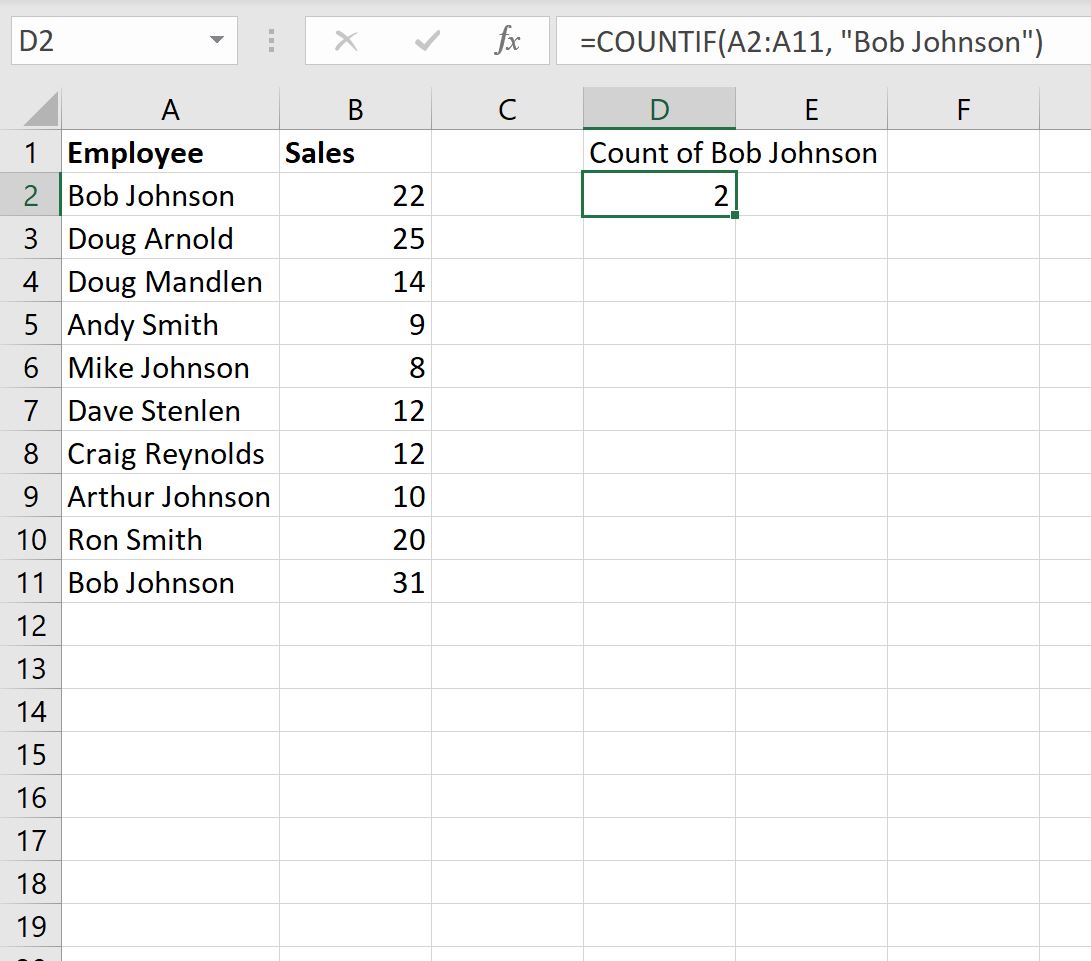
In some cases, you might only want to list the names of visible sheets:
Sub ListVisibleSheets()
Dim ws As Worksheet
Dim sheetNames As String
For Each ws In ThisWorkbook.Worksheets
If ws.Visible = xlSheetVisible Then
sheetNames = sheetNames & ws.Name & vbNewLine
End If
Next ws
MsgBox sheetNames, vbInformation, "Visible Sheet Names"
End Sub
This script checks the `Visible` property of each sheet before adding it to the string of names.
Using SpecialCells for Selected Sheets

If you’re dealing with sheets that are currently selected by the user, you can retrieve those names like this:
Sub SelectedSheetNames()
Dim ws As Worksheet
Dim sheetNames As String
On Error Resume Next
For Each ws In ActiveWindow.SelectedSheets
If ws.Type = xlWorksheet Then
sheetNames = sheetNames & ws.Name & vbNewLine
End If
Next ws
On Error GoTo 0
If sheetNames = "" Then
MsgBox "No sheets selected", vbInformation
Else
MsgBox sheetNames, vbInformation, "Selected Sheet Names"
End If
End Sub
This method will list only the sheets that are currently selected:
- It uses error handling to avoid crashes if no sheets are selected.
- It prints the names of the selected sheets to a message box.
⚙️ Note: Always ensure your VBA macros are enabled in Excel for these scripts to run.
In this exploration, we’ve covered multiple strategies for listing, checking, and processing sheet names in an Excel workbook using VBA. Whether you need to loop through all sheets, work with visible sheets only, or gather names from selected sheets, these methods provide you with the flexibility needed for various Excel tasks. Each approach has its use case, allowing you to adapt your script to the specific requirements of your project.
The ability to dynamically retrieve sheet names not only enhances automation but also aids in developing more robust and adaptable Excel tools. By understanding these VBA techniques, you can streamline your workflow, reduce manual labor, and tackle complex data management challenges with ease.
How can I get sheet names without VBA?

+
While VBA provides the most dynamic and automated solutions, you can manually get sheet names by right-clicking the Excel workbook’s bottom tab area, selecting ‘View Code’, and then using the Project Explorer to see all sheet names.
Can I use these VBA scripts to delete sheets?

+
Yes, once you have the names or references to sheets, you can modify the scripts to delete them. Be cautious with deletion operations to avoid unintended loss of data.
What if my workbook has hundreds of sheets?

+
Even with a large number of sheets, these scripts will work efficiently. However, for optimal performance, consider using a workbook with fewer sheets or utilize advanced techniques like collections or dictionaries for faster access.