5 Ways to Extract Excel Data onto Separate Sheets

Extracting data from an Excel spreadsheet onto separate sheets can significantly enhance your ability to organize, analyze, and present information. Whether you are dealing with large datasets, need to categorize information, or simply want to streamline your reporting processes, understanding how to automate this task can save time and reduce errors. Here, we will delve into five effective methods to extract Excel data onto separate sheets, tailored to suit various user levels and needs.
Using Excel’s Built-in Features

Excel's native features provide some of the simplest ways to manage data:
- Advanced Filter: This tool allows you for filtering data based on complex criteria, which can then be copied to new sheets.
- Data Validation: Set up data validation rules to dynamically categorize data into different sheets.
- Subtotal: While not exactly for extracting data onto separate sheets, this function can group and summarize data that you could then manually extract.
To use Advanced Filter:
- Select your data range or entire table.
- Go to the 'Data' tab > 'Sort & Filter' > 'Advanced'.
- Choose where you want to filter the list (in place or to another location).
- Enter your criteria range if needed and click 'OK'.
- If copying to another location, specify the destination. You can then manually copy filtered data to new sheets or automate with VBA.
Employing VBA Macros

VBA (Visual Basic for Applications) allows for a high degree of automation in Excel, including the task of extracting data to separate sheets:
Creating a VBA Macro to Extract Data
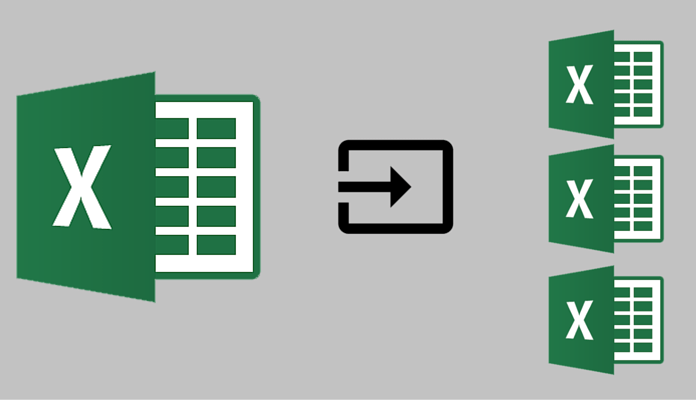
Here's a basic example of how to write a VBA script to extract data:
Sub ExtractDataByCriteria()
Dim wsMain As Worksheet, ws As Worksheet
Dim rngData As Range, cell As Range
Dim dict As Object
Set dict = CreateObject("Scripting.Dictionary")
Set wsMain = ThisWorkbook.Sheets("Sheet1")
Set rngData = wsMain.UsedRange
For Each cell In rngData.Columns(1).Cells 'Assuming Column A contains your criteria
If Not dict.Exists(cell.Value) Then
dict.Add cell.Value, cell.Value
Set ws = ThisWorkbook.Sheets.Add(After:=Sheets(Sheets.Count))
ws.Name = cell.Value
End If
Next cell
dict.RemoveAll
Set dict = Nothing
End Sub
💡 Note: This script assumes that your criteria for categorization are in Column A. Modify the column reference if your data structure differs.
Leveraging Power Query

Power Query, an Excel add-in, excels in data transformation and can be used to:
- Group and categorize data.
- Load the results into separate sheets or even new workbooks.
Here is how you can use Power Query to extract data:
- Select your data range.
- Go to 'Data' tab > 'From Table/Range'.
- In the Query Editor, group by your criteria column:
- Right-click the column you want to group by > 'Group By'.
- Choose 'All Rows' as the operation to keep all related data.
- Click on 'Close & Load' and choose 'Load To' > 'Table' in a new worksheet.
Using Microsoft Power Pivot

Power Pivot expands on Power Query by allowing:
- Analysis of large datasets with pivot tables.
- Direct data extraction into new sheets through connected tables or reports.
How to Use Power Pivot for Data Extraction:

- Activate Power Pivot in Excel by enabling the add-in.
- Import data into Power Pivot from your source sheet.
- Create calculated columns or measures to categorize your data.
- Build pivot tables or charts from Power Pivot, choosing where you want the output to be placed.
Implementing Python with Excel

Python can be a powerful tool for Excel data manipulation when combined with libraries like `openpyxl` or `pandas`. Here's a sample Python script to extract data to separate sheets:
import openpyxl
wb = openpyxl.load_workbook('yourfile.xlsx')
source_sheet = wb.active
data = []
# Assuming data is in columns A and B
for row in source_sheet.iter_rows(min_row=2, max_col=2, values_only=True):
data.append(row)
# Extract unique categories from data
categories = {item[0] for item in data if item[0] is not None}
for category in categories:
new_sheet = wb.create_sheet(category)
for row in data:
if row[0] == category:
new_sheet.append(row)
wb.save('newfile.xlsx')
This script creates new sheets based on the categories in column A and copies the corresponding data.
🔄 Note: Ensure you have the `openpyxl` library installed before running this script. Use `pip install openpyxl` to install it if you haven't already.
Summing Up
Each method described here has its own set of advantages, catering to different user skills and data analysis needs. From the straightforward Excel features for quick tasks to the sophisticated use of Python for extensive automation, your choice depends on the complexity of your data, your comfort with programming, and your specific project requirements. By leveraging these techniques, you can not only enhance your data management capabilities but also increase productivity, ensure data integrity, and facilitate better decision-making.
What are the benefits of extracting data to separate sheets?

+
Extracting data to separate sheets allows for better data organization, easier analysis, and improved reporting capabilities. It simplifies access to specific data sets, reduces data clutter, and can facilitate collaborative work when different team members need to work on separate parts of the data.
Can I automate the process of extracting data?

+
Yes, you can automate this process using tools like VBA macros, Power Query, or Python scripts, which can run according to predefined criteria and significantly reduce manual work.
Which method should I use for large datasets?

+
For large datasets, methods like Power Query or Python scripts are more efficient due to their capability to handle complex data transformations and volume with less performance impact.