5 Ways to Export Data to Multiple Excel Sheets in PHP
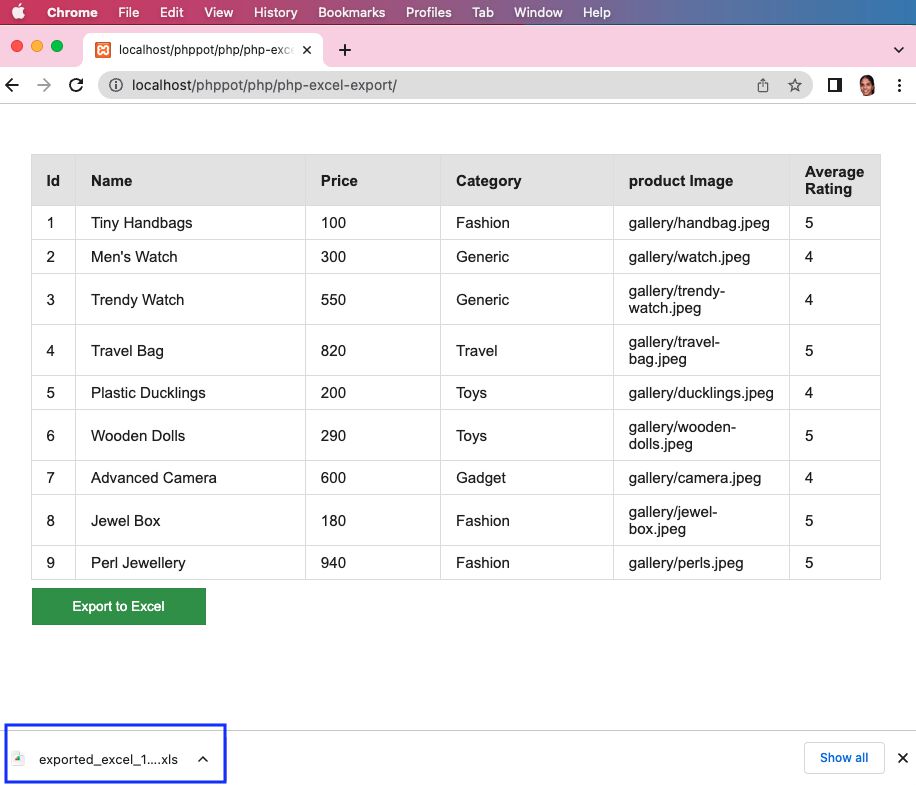
Data management in PHP often involves exporting datasets to Microsoft Excel files for analysis, reporting, or data preservation. However, when dealing with large datasets or various categories of data, it becomes necessary to split this information into multiple sheets within one Excel workbook. In this blog post, we'll explore five different methods to export data to multiple Excel sheets in PHP. Each method has its own merits, and choosing the right one depends on your project's specific needs, including performance requirements, library dependencies, and complexity of data.
Method 1: Using PHPExcel Library

The PHPExcel library is a well-regarded tool for handling Excel files in PHP due to its robust functionality. Here’s how you can use PHPExcel to export data into multiple sheets:
- Download or install PHPExcel via Composer:
- Include PHPExcel in your PHP script:
- Create an Excel workbook and define multiple sheets:
- Populate each sheet with data from different sources or databases.
Example Code:
```php require 'path/to/PHPExcel/Classes/PHPExcel.php'; $objPHPExcel = new PHPExcel(); $objPHPExcel->setActiveSheetIndex(0)->setTitle('Sheet1'); // Populate first sheet with data $objPHPExcel->createSheet()->setTitle('Sheet2'); $objPHPExcel->setActiveSheetIndex(1)->setCellValue('A1', 'Second Sheet Data'); // Populate second sheet $objWriter = PHPExcel_IOFactory::createWriter($objPHPExcel, 'Excel2007'); $objWriter->save('path/to/save/myFile.xlsx'); ```📝 Note: PHPExcel is no longer maintained since version 1.8.1 (Jan 2016), and users are encouraged to switch to PhpSpreadsheet, its successor.
Method 2: Using PhpSpreadsheet Library

PhpSpreadsheet is the modern replacement for PHPExcel, offering similar functionality with ongoing support and development. Here’s how you can use it:
- Install PhpSpreadsheet via Composer.
- Create a new workbook, add sheets, and populate with data:
Example Code:
```php use PhpOffice\PhpSpreadsheet\Spreadsheet; use PhpOffice\PhpSpreadsheet\Writer\Xlsx; $spreadsheet = new Spreadsheet(); $spreadsheet->getActiveSheet()->setTitle('Sheet1'); // Populate first sheet with data $sheet2 = $spreadsheet->createSheet()->setTitle('Sheet2'); $sheet2->setCellValue('A1', 'Second Sheet Data'); // Populate second sheet $writer = new Xlsx($spreadsheet); $writer->save('path/to/save/myFile.xlsx'); ```Method 3: Using fputcsv and ZipStream

This method involves writing CSV files for each sheet and then using the ZipStream library to combine them into a single Excel workbook. Here's how:
- Create CSV files for each sheet of data.
- Use ZipStream to merge these CSV files into one Excel file:
Example Code:
```php // Assuming data for each sheet is in an array $sheetData = [ ['First Sheet' => ['A1', 'B1', 'C1']], ['Second Sheet' => ['X1', 'Y1', 'Z1']] ]; $zip = new ZipStream\ZipStream('example.xlsx'); foreach ($sheetData as $sheetName => $data) { $tmpFile = tempnam(sys_get_temp_dir(), 'sheet'); $file = fopen($tmpFile, 'w'); foreach ($data as $row) { fputcsv($file, $row); } fclose($file); $zip->addFileFromPath($sheetName . '.csv', $tmpFile); } $zip->finalize(); // closes the zip file ```📝 Note: This method provides good performance for large datasets but doesn't maintain Excel-specific features like formatting.
Method 4: Using Spout Library

Spout is optimized for reading and writing spreadsheet files with low memory consumption:
- Install Spout via Composer.
- Use Spout to create multiple sheets in an Excel file:
Example Code:
```php use Box\Spout\Writer\WriterFactory; use Box\Spout\Common\Type; $writer = WriterFactory::create(Type::XLSX); $writer->openToFile('example.xlsx'); $sheet1 = $writer->getCurrentSheet(); $sheet1->setName('Sheet1'); // Populate first sheet with data $sheet2 = $writer->addNewSheetAndMakeItCurrent(); $sheet2->setName('Sheet2'); // Populate second sheet with data $writer->close(); ```Method 5: Direct Manipulation with PHP

This method involves manual manipulation of the Excel XML format to create sheets. Although not as straightforward, it's useful for custom needs:
- Create an XML structure for the Excel file.
- Insert data into different sheets within this structure:
Example Code:
```php $xml = 'By exploring these methods, developers can choose the most appropriate technique for their PHP application to export data efficiently into multiple Excel sheets. Each approach offers unique benefits, whether it be simplicity, performance, support, or flexibility. Understanding these methods allows for a well-rounded approach to data management and reporting.
Which library is best for large datasets?

+
PhpSpreadsheet and Spout are optimized for handling large datasets. They offer better performance and lower memory consumption compared to other methods.
Can I format cells when exporting data?

+
Yes, libraries like PHPExcel and PhpSpreadsheet allow for detailed cell formatting, including font styles, colors, and number formats.
Is there a way to avoid PHP libraries?

+
You can manually manipulate Excel XML as shown in Method 5, but it’s complex and not recommended for regular use due to the intricacy of Excel’s XML structure.
What if I need real-time Excel creation?

+
Streaming libraries like ZipStream and Spout allow for real-time Excel creation, where data can be written as it’s processed without loading the entire dataset into memory.
Can I merge multiple existing Excel files?

+
Yes, you can read existing Excel files using libraries like PHPExcel or PhpSpreadsheet, then combine or append their content into a new workbook.