Edit Excel Sheets Easily with Python: A Beginner's Guide
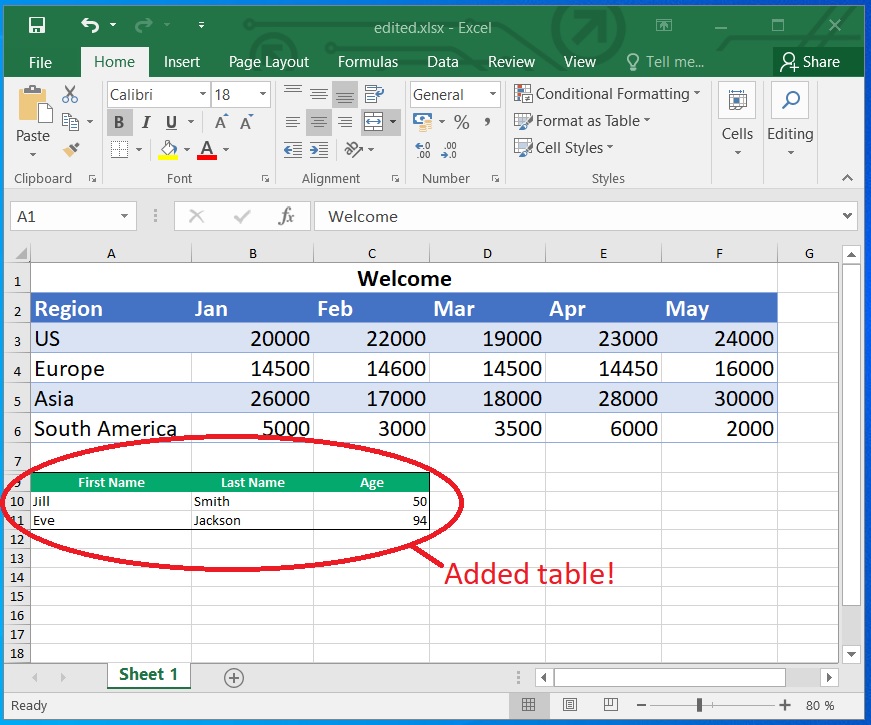
Editing Excel sheets can seem daunting at first, especially for those who are new to data manipulation or are not familiar with Microsoft Excel's complex features. However, with Python, this task becomes manageable even for beginners. Python's pandas library, designed specifically for data analysis and manipulation, offers a straightforward and efficient way to edit, analyze, and automate changes in Excel files. Here's how you can get started:
Setting Up Your Environment

Before you dive into coding, ensure you have Python installed on your computer. Here’s what you need to do:
- Install Python from the official Python website or via your OS package manager if you haven’t already.
- Open your command line (Command Prompt for Windows, Terminal for macOS/Linux) and install pandas:
pip install pandas
- While pandas is the main tool for data manipulation, installing openpyxl can help with Excel file I/O:
pip install openpyxl
Loading an Excel File

Begin by loading an Excel file into a pandas DataFrame. This allows you to work with the data in a more manageable way:
import pandas as pd
df = pd.read_excel(‘data.xlsx’)
📝 Note: If your Excel file has multiple sheets, you can specify which one to load by using the sheet_name
parameter.
Viewing and Understanding Your Data

Once the file is loaded, you’ll want to inspect your data:
- Use
df.head()
to see the first few rows of the DataFrame. df.tail()
for the last few rows.df.info()
provides a summary of the DataFrame, including column data types.
Editing Your Data
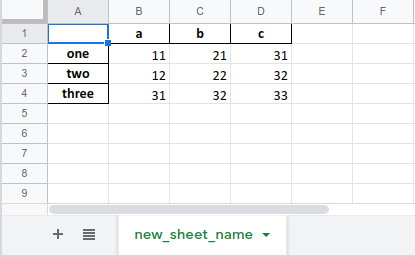
Pandas offers several methods to edit your Excel data:
Adding or Removing Columns
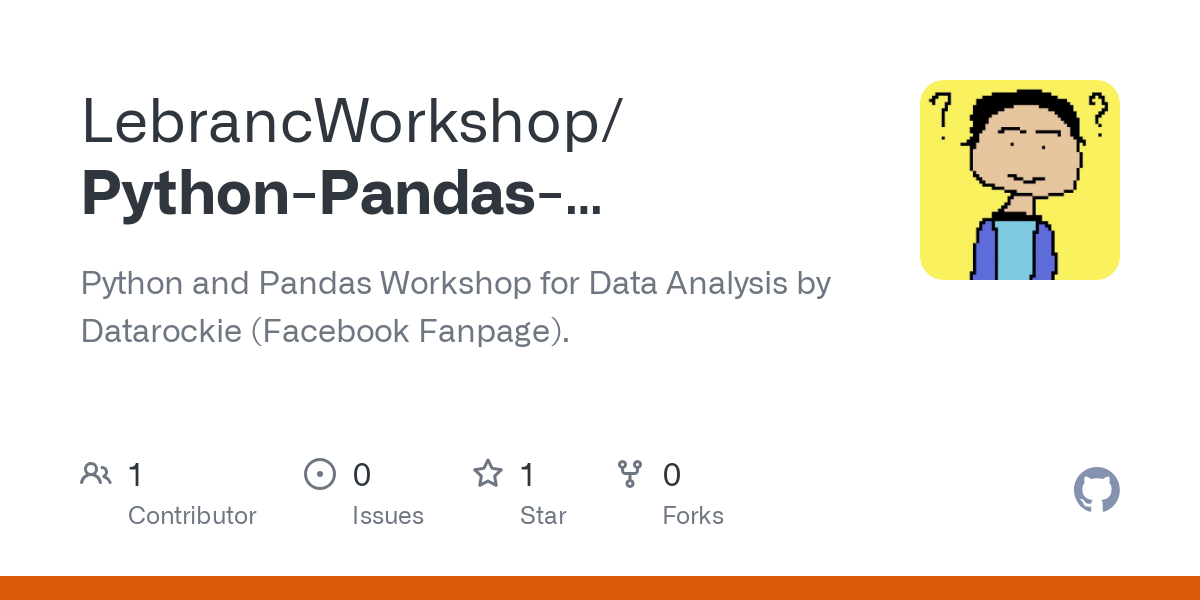
If you need to add a new column based on existing data:
df[‘New Column’] = df[‘Existing Column’].apply(lambda x: x * 2)
Or, remove a column:
df = df.drop(‘Column To Remove’, axis=1)
Editing Cell Values

To change values in specific cells:
df.at[0, ‘Name’] = ‘New Value’
Applying Operations

You can apply functions across entire columns or rows:
df[‘Column’] = df[‘Column’].apply(lambda x: x + 10 if x > 10 else x)
Using Conditions

Conditionally change values:
df.loc[df[‘Score’] > 90, ‘Grade’] = ‘A’
Saving Your Edited Excel File

Once your edits are complete, you can save the DataFrame back to an Excel file:
df.to_excel(‘edited_data.xlsx’, index=False)
📝 Note: Setting `index=False` avoids writing row numbers as an additional column in the Excel sheet.
In wrapping up this guide to editing Excel sheets with Python, let's reflect on the key takeaways. Python, through the pandas library, provides a powerful and accessible tool for those looking to automate or simplify data management tasks in Excel. Here’s what we've covered:
- How to set up your Python environment for Excel manipulation.
- The process of loading Excel files into Python for editing.
- Various methods to view and understand your data in a DataFrame.
- Techniques for editing, adding, or removing data within your spreadsheets.
- Saving your changes back to Excel format.
The advantage of using Python for Excel manipulation is not just in the ease of execution but also in the scalability and automation capabilities it offers. For beginners, this approach can significantly cut down the learning curve associated with complex Excel features while maintaining flexibility and control over data operations. Whether you're performing basic edits or complex data transformations, Python's clear syntax and the structured nature of pandas make it an excellent tool for Excel data management. As you become more comfortable with these techniques, you'll find yourself more efficient and perhaps even enjoying the process of data manipulation with Python.
Do I need to know Excel to use Python for editing Excel sheets?
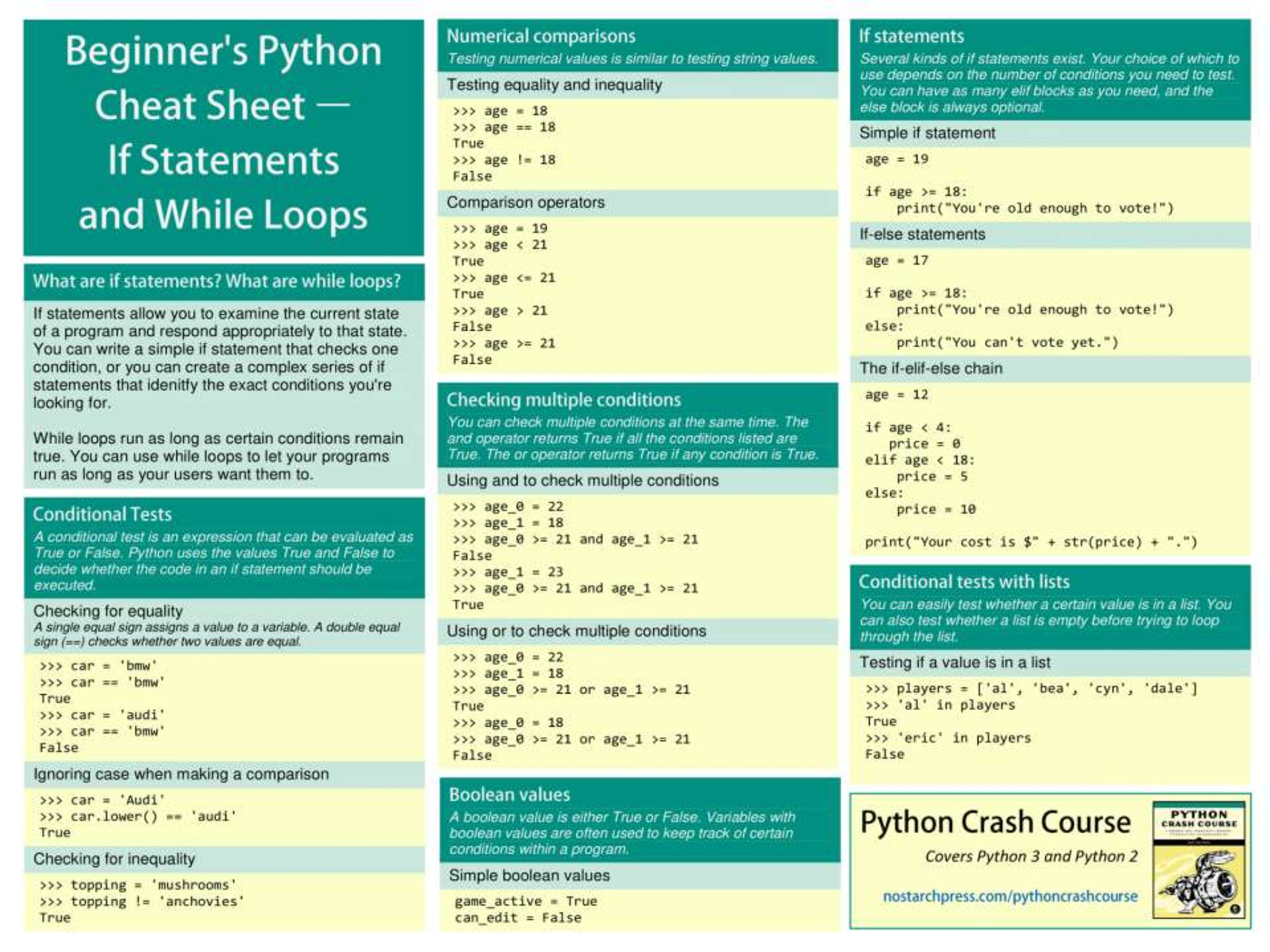
+
No, Python allows you to manipulate Excel files without needing deep Excel knowledge. However, understanding basic Excel concepts can help in translating your needs into Python operations.
Can Python edit large Excel files?

+
Yes, Python’s pandas library is designed to handle large datasets efficiently. However, for extremely large files, you might need to consider memory management or process the data in chunks.
Is Python faster than using Excel for data manipulation?

+
It depends on the task. For automating repetitive tasks or processing large datasets, Python can be significantly faster. For individual edits, Excel might be more user-friendly.
Can I use Python to format cells or apply styles in Excel?

+
Yes, with additional libraries like openpyxl or xlsxwriter, you can control cell formatting, styles, charts, and even protect sheets.
What if I need to perform very complex calculations or data analysis in Excel?

+
Python, with its extensive data science libraries like numpy and scipy, can handle complex calculations and analyses with ease. You can automate Excel data processing and integrate with data analysis libraries for advanced operations.