Duplicate Excel Sheets Quickly with VBA Script

In today’s fast-paced business environment, Microsoft Excel remains an indispensable tool for organizing, analyzing, and presenting data. However, handling large datasets often requires duplicating sheets, which can become a repetitive and time-consuming task. In this blog post, we’ll delve into how you can automate the process of duplicating Excel sheets using Visual Basic for Applications (VBA). This script will not only save you time but also increase your efficiency by eliminating manual errors.
Why Automate Sheet Duplication?

Duplicating a sheet in Excel manually involves several steps, from right-clicking on the tab, selecting ‘Move or Copy’, choosing the destination, and ensuring the ‘Create a copy’ checkbox is ticked. Here’s why automation is beneficial:
- Consistency: VBA ensures each sheet is copied exactly as intended, reducing the risk of errors.
- Time Efficiency: Automating repetitive tasks allows for more focus on strategic analysis or other critical tasks.
- Customization: Scripts can be tailored to perform additional actions beyond basic duplication.
Writing the VBA Script

Here’s how you can write a simple VBA script to duplicate sheets in Excel:
Step 1: Open the VBA Editor

To access VBA:
- Open Excel and press
ALT + F11
to open the Visual Basic Editor.
Step 2: Insert a Module
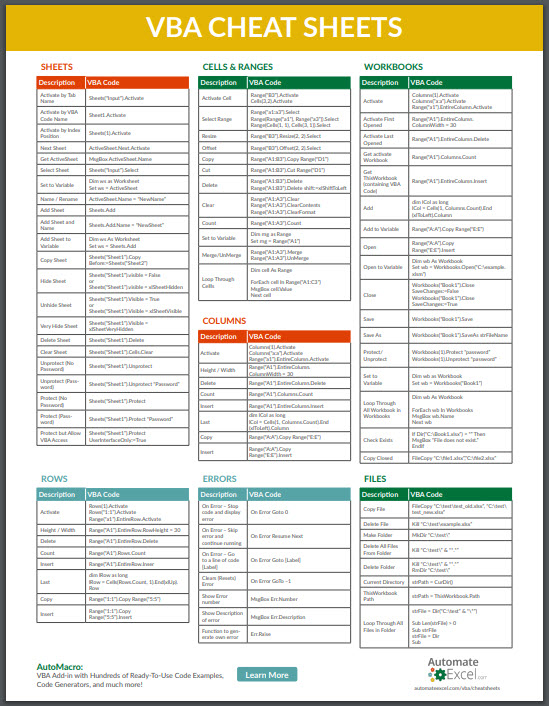
In the VBA Editor:
- Click
Insert > Module
to add a new module where you’ll write your script.
Step 3: Write the Script

Here’s the VBA code to duplicate a sheet:
Sub CopySheet()
Dim ws As Worksheet
Dim wsCopy As Worksheet
Set ws = ThisWorkbook.Sheets("Sheet1") 'Replace "Sheet1" with the name of your source sheet
ws.Copy After:=ThisWorkbook.Sheets(ThisWorkbook.Sheets.Count)
Set wsCopy = ActiveSheet
wsCopy.Name = ws.Name & " Copy"
'If you want to apply additional formatting or changes to the copied sheet:
'wsCopy.Range("A1").Value = "This is a duplicate of " & ws.Name
End Sub
💡 Note: This script copies the specified sheet and places the duplicate after the last sheet in your workbook, naming it with " Copy" appended to the original name. You can modify the script for different behaviors, like naming or positioning.
Step 4: Run the Script

- To execute the script, press
F5
in the VBA Editor, or return to Excel and run it from theDeveloper
tab underMacros
.
Optimizing the VBA Script

For more advanced use:
- Batch Copy: Loop through multiple sheets to duplicate them in one go.
- Conditional Copying: Copy sheets based on certain conditions (e.g., sheets with a particular name or date).
- User Interface: Add a form for selecting which sheets to copy.
Here’s an example of how you might modify the script for batch copying:
Sub BatchCopySheets()
Dim ws As Worksheet
Dim wsCopy As Worksheet
Dim i As Integer
For i = 1 To 3 'This will duplicate the first three sheets
Set ws = ThisWorkbook.Sheets(i)
ws.Copy After:=ThisWorkbook.Sheets(ThisWorkbook.Sheets.Count)
Set wsCopy = ActiveSheet
wsCopy.Name = ws.Name & " Copy"
Next i
End Sub
This script will create copies of the first three sheets in your workbook.
Handling Edge Cases

When scripting, consider potential issues:
- Duplicate Names: VBA will not allow two sheets with the same name. Ensure your script handles renaming or prompts for user input.
- Protected Sheets: Your script might fail if attempting to copy or modify protected sheets. Include error handling:
Sub CopySheetWithErrorHandling()
On Error GoTo ErrorHandler
'Your copying code here
Exit Sub
ErrorHandler:
MsgBox "An error occurred: " & Err.Description
End Sub
Summary

Automation in Excel, especially using VBA, can transform how you handle repetitive tasks. By scripting the duplication of sheets, you not only streamline your workflow but also minimize errors, allowing for more focus on data analysis and interpretation.
Can VBA scripts handle all types of Excel files?

+
Yes, VBA scripts can be used with any Excel file format (.xls, .xlsx, .xlsm). However, some features might only work with macros-enabled files (.xlsm).
What if my workbook contains data validation?

+
Data validations are typically copied over when you duplicate a sheet, but you might need to adjust references manually if the copied sheet changes contexts.
Is it possible to schedule VBA scripts to run automatically?

+
While Excel doesn’t provide native scheduling capabilities, you can use Windows Task Scheduler to open Excel, run your macro, and close it using a batch file or other automation tools.