Quick VBA Guide: Delete Multiple Excel Sheets

When working with Microsoft Excel, especially in tasks that involve data analysis or management, managing workbook sheets becomes essential. Often, users need to delete multiple Excel sheets quickly and efficiently. This guide will walk you through the VBA (Visual Basic for Applications) code to streamline this process, ensuring productivity and time management in your daily Excel operations.
Understanding VBA and Its Necessity

VBA is an event-driven programming language from Microsoft that is part of the Microsoft Office suite. VBA allows Excel users to automate repetitive tasks, thus saving time and reducing the potential for human error. Deleting multiple sheets manually can be tedious, particularly when dealing with workbooks containing numerous sheets. VBA comes to the rescue, allowing for bulk operations with a single command.
Steps to Delete Multiple Excel Sheets

Here’s how you can use VBA to delete multiple Excel sheets:
- Open the VBA Editor: Press
Alt + F11
to open the VBA Editor or navigate via the Developer tab if it's enabled. - Insert a New Module: Right-click on any of the objects in the Project Explorer, select "Insert," then choose "Module."
- Paste the Code: Copy and paste the following VBA code into the new module: ```vba Sub DeleteSheets() Dim ws As Worksheet Dim i As Integer Dim sheetName As Variant Dim sheetArray As Variant ' Define the names of the sheets to delete in an array sheetArray = Array("Sheet2", "Sheet3", "Sheet4") ' Loop through each sheet in the workbook For i = ThisWorkbook.Sheets.Count To 1 Step -1 Set ws = ThisWorkbook.Sheets(i) For Each sheetName In sheetArray If ws.Name = sheetName Then ' Application.DisplayAlerts = False prevents confirmation prompts Application.DisplayAlerts = False ws.Delete Application.DisplayAlerts = True Exit For End If Next sheetName Next i End Sub ```
- Run the Macro: Either press
F5
or go back to Excel, pressAlt + F8
, select "DeleteSheets," and click "Run."
👉 Note: Ensure you've saved your workbook before running this macro, as VBA cannot undo the deletion of sheets.
Customizing Your VBA Code

- Sheet Names: Replace
"Sheet2", "Sheet3", "Sheet4"
with the actual names of the sheets you wish to delete. - Criteria for Deletion: If you want to delete sheets based on a different condition, such as sheet type or content, adjust the
If
condition in the loop accordingly.
Error Handling

When scripting with VBA, error handling is crucial. Here’s how you might incorporate error handling:
Sub DeleteSheetsWithErrorHandling()
On Error GoTo ErrorHandler
' Your existing DeleteSheets code here
Exit Sub
ErrorHandler:
MsgBox "An error occurred: " & Err.Description
End Sub
📌 Note: Error handling ensures your VBA script does not break unexpectedly, providing you with an error message instead.
Limitations and Considerations

- Order of Deletion: Sheets are deleted from the last to the first to avoid index shifting errors.
- Active Sheet: Be cautious when deleting the currently active sheet, as it might cause the macro to fail.
- User Prompts: The macro disables prompts to ensure automation, but remember to re-enable alerts after the operation.
Benefits of Automating Sheet Deletion

Automating the process to delete multiple Excel sheets offers several advantages:
- Time-Saving: Automates tedious tasks, allowing you to focus on more strategic work.
- Efficiency: Reduces the chance of errors by removing manual interaction.
- Repeatability: The macro can be reused for similar tasks across different workbooks.
Understanding and utilizing VBA to manage Excel sheets can transform the way you handle data within Microsoft Excel. By automating routine tasks like deleting multiple sheets, you can enhance productivity, ensure data integrity, and streamline your workflow.
Excel, though powerful for data manipulation, requires users to engage with VBA to unlock its full potential for automation. This VBA code snippet for deleting sheets demonstrates the simplicity and utility of VBA in everyday tasks, fostering efficiency and accuracy in data management.
Can I delete sheets based on their position rather than their names?

+
Yes, you can modify the VBA code to loop through sheets by their index. For example, to delete the first three sheets, you would use: For i = 1 To 3
Is it possible to recover a sheet after it’s been deleted using VBA?

+
Once a sheet is deleted using VBA, it is not recoverable through VBA. Ensure you save your work beforehand or use Excel’s ‘Undo’ feature if possible.
What if I want to delete all sheets except one?

+
You can modify the loop condition to skip the sheet you want to keep. For example, if you want to keep “Sheet1,” modify the code to only delete sheets where ws.Name <> “Sheet1”
.
How can I make sure my VBA code runs for all open workbooks?
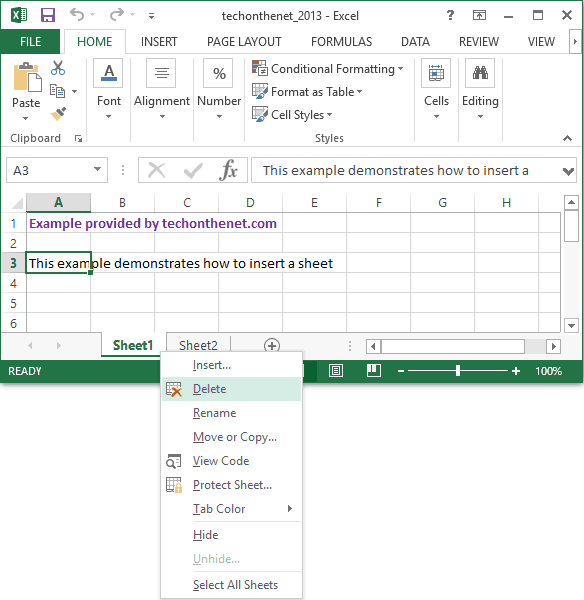
+
You’ll need to loop through all open workbooks with a loop like For Each wb In Application.Workbooks
, then apply your deletion logic within this loop.