Delete Data Across Multiple Excel Sheets with VBA

Managing large datasets in Excel can be a daunting task, especially when you need to delete specific data across multiple sheets within a workbook. VBA (Visual Basic for Applications) scripting can automate this task, saving you countless hours of manual work. In this post, we'll explore how you can use VBA to efficiently delete data across multiple sheets in Microsoft Excel. This approach is not only efficient but also reduces the risk of human error, ensuring consistency and accuracy.
Why Use VBA for Data Deletion?

VBA in Excel allows you to extend the functionality of spreadsheets beyond what’s available through the regular Excel interface. Here are some reasons why using VBA for data deletion is advantageous:
- Automation: Automate repetitive tasks, reducing the time and effort required.
- Consistency: Ensure that data deletion occurs uniformly across multiple sheets.
- Precision: Use conditions to delete specific data, making your operations more precise.
Setting Up Your Environment for VBA

Before diving into scripting, ensure your environment is set up for VBA programming:
- Open Excel: Start Microsoft Excel.
- Enable Developer Tab: If not already enabled, you can do this via Excel Options > Customize Ribbon > check Developer.
- Access VBA Editor: Press
Alt + F11
to open the Visual Basic for Applications editor. - Insert a New Module: Right-click on any of the objects in the “Microsoft Excel Objects” folder in the Project Explorer, select “Insert” > “Module” to create a new module for your VBA code.
VBA Code for Deleting Data Across Multiple Sheets

Below is a comprehensive VBA script designed to delete data from multiple sheets based on specific criteria. This script can be customized to fit various needs:
Sub DeleteDataAcrossSheets()
Dim ws As Worksheet
Dim lastRow As Long
Dim lastCol As Long
Dim delColumn As Range
Dim i As Integer
'Loop through all worksheets in the workbook
For Each ws In ThisWorkbook.Worksheets
With ws
'Find the last row and column with data
lastRow = .Cells(.Rows.Count, 1).End(xlUp).Row
lastCol = .Cells(1, .Columns.Count).End(xlToLeft).Column
'Define the range to search in
Set delColumn = .Range(.Cells(1, 1), .Cells(lastRow, lastCol))
'Loop through each row
For i = lastRow To 1 Step -1
If .Cells(i, 1).Value = "ToDelete" Then 'Replace "ToDelete" with your criteria
.Rows(i).Delete
End If
Next i
'Optional: If you want to clear specific cells instead of deleting rows:
'delColumn.ClearContents
End With
Next ws
MsgBox "Data deletion process complete!", vbInformation
End Sub
Explaining the Code

- Workbook and Worksheet Handling: The script iterates through each worksheet in the current workbook using a For Each loop.
- Finding Last Row and Column: It dynamically finds the last row and column with data to ensure it covers all used cells.
- Search and Delete: The script checks each cell in the first column for the text “ToDelete” (which can be changed to match any criteria) and deletes the row if found. You could also clear content instead of deleting rows by using
ClearContents
instead ofDelete
.
🛈 Note: Ensure you back up your data before running scripts that delete information. Mistakes in VBA can result in data loss.
Best Practices for Using VBA to Delete Data

- Backup Your Data: Always create a backup of your workbook before running any deletion scripts.
- Test Your Script: Run your script on a small subset or copy of the data to ensure it behaves as expected.
- Clear Temporary Variables: Make sure to clear any temporary variables or ranges after use to free up memory.
- Use Error Handling: Incorporate error handling into your scripts to manage unexpected issues gracefully.
Optimizing and Troubleshooting Your VBA Scripts

If your script runs slowly or causes Excel to crash, consider these tips:
- Disable Screen Updating: Add
Application.ScreenUpdating = False
at the start of your script and turn it back on at the end to speed up processing. - Turn Off Calculations: Temporarily disable automatic calculations using
Application.Calculation = xlCalculationManual
. Restore withxlCalculationAutomatic
at the end. - Debugging: Use breakpoints and the Debug.Print statement to monitor what’s happening within loops.
By following these steps and best practices, you’ll be able to manage and delete data across multiple Excel sheets with ease and precision, enhancing your productivity in data management tasks.
For those looking to dive deeper into VBA or facing specific challenges, consider exploring online forums or VBA documentation for more advanced techniques.
Finally, always remember that while automation like this can save time, it’s crucial to approach data deletion with caution, as mistakes can be costly. Regularly save your work and ensure you have backups before executing scripts that modify your data.
Can VBA delete data from all sheets at once?

+
Yes, VBA can loop through all sheets in a workbook, applying the same deletion logic to each one as demonstrated in the provided script.
Is there a risk of losing important data with this method?

+
There is always a risk when automating data deletion. Make sure to back up your workbook and test the script on a small sample first.
Can this VBA script be run on protected sheets?
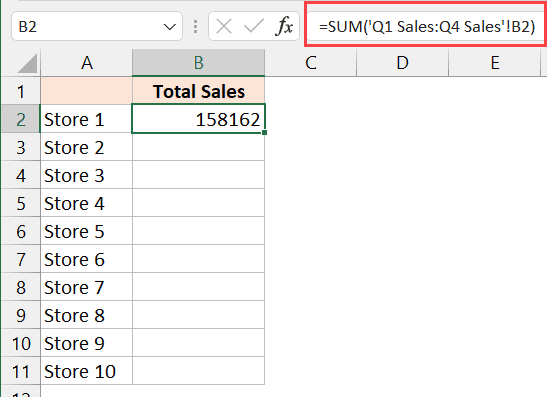
+
If the sheets are password-protected, the script would need to include commands to unprotect and then reprotect the sheets, which could pose security risks if not handled properly.
What happens if the keyword for deletion appears in the header row?

+
As the script starts from the last row and moves upwards, headers would typically not be affected since they’re often in the first row. However, ensure your script accounts for headers if they contain the deletion keyword.
How can I optimize my VBA script for better performance?
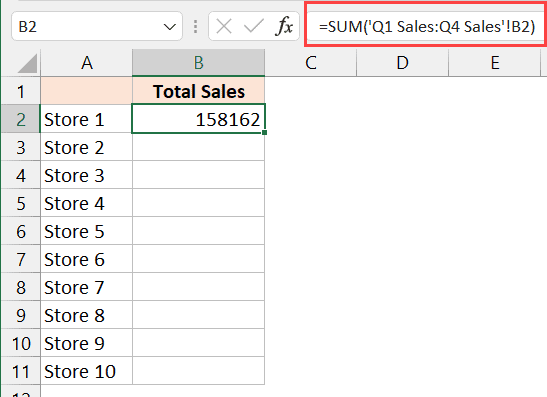
+
Turn off screen updates and automatic calculations during script execution. Also, consider using arrays for large data sets to minimize direct interactions with the worksheet.