5 Ways to Create Excel Tables Using Java
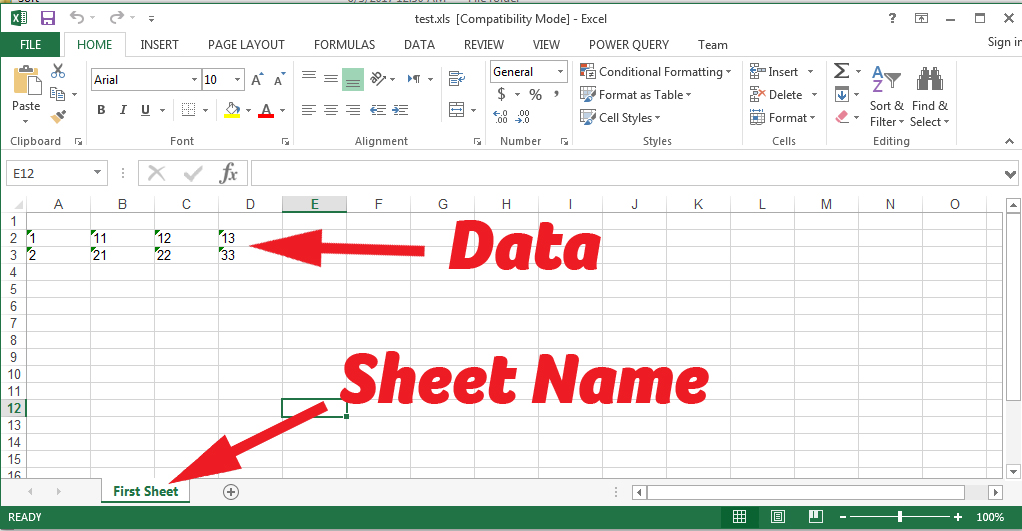
Working with spreadsheets is a common task in business applications, data analysis, and numerous other fields. Java, as a versatile programming language, provides several options for creating Excel tables through libraries that offer robust functionalities. In this blog post, we'll explore five different methods to create Excel tables using Java, each with its own advantages, allowing you to choose the best approach for your specific needs.
1. Using Apache POI

Apache POI is the most widely used library for working with Microsoft Office documents, including Excel files. Here's how you can create a simple Excel table using Apache POI:
- Download Apache POI from their official site.
- Add the necessary dependencies to your project.
- Write the following code to create an Excel table:
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class POIExcelTable {
public static void main(String[] args) throws Exception {
Workbook workbook = new XSSFWorkbook();
Sheet sheet = workbook.createSheet("Sample Sheet");
Row rowHeader = sheet.createRow(0);
Cell cellHeader = rowHeader.createCell(0);
cellHeader.setCellValue("Header");
Cell cellData = sheet.createRow(1).createCell(0);
cellData.setCellValue("Data");
FileOutputStream fileOut = new FileOutputStream("sample.xlsx");
workbook.write(fileOut);
fileOut.close();
workbook.close();
}
}
📋 Note: Apache POI supports both XLS and XLSX file formats, with XLSX being the modern format.
2. Using JExcelAPI

JExcelAPI is another library for manipulating Excel files, with a focus on performance for read and write operations. Here's how to create an Excel table with JExcelAPI:
- Download JExcelAPI from SourceForge.
- Incorporate the library into your Java project.
- Implement the following code:
import jxl.*;
import jxl.write.*;
import java.io.File;
public class JExcelAPIExcelTable {
public static void main(String[] args) throws Exception {
WorkbookSettings wbSettings = new WorkbookSettings();
Workbook workbook = Workbook.createWorkbook(new File("sample.xls"), wbSettings);
WritableSheet sheet = workbook.createSheet("Sheet 1", 0);
Label label = new Label(0, 0, "Header");
sheet.addCell(label);
label = new Label(0, 1, "Data");
sheet.addCell(label);
workbook.write();
workbook.close();
}
}
3. Using Aspose.Cells

Aspose.Cells provides comprehensive features for Excel processing, including formula calculation, charting, and formatting. Here’s how to create a basic Excel table with Aspose.Cells:
- Add Aspose.Cells to your project (available via Maven).
- Use the following code snippet:
import com.aspose.cells.*;
public class AsposeExcelTable {
public static void main(String[] args) throws Exception {
//Instantiate a new Workbook
Workbook wb = new Workbook();
Worksheet worksheet = wb.getWorksheets().get(0);
//Input a value in cell A1
Cells cells = worksheet.getCells();
cells.get("A1").setValue("Header");
cells.get("A2").setValue("Data");
//Save workbook
wb.save("sample.xlsx");
}
}
4. Using JXL (Jakarta Poi API)

JXL is an older library but still relevant for legacy systems or specific use cases where performance is key. Here’s how you can use JXL:
- Include JXL in your project.
- Write the following code to generate an Excel file:
import jxl.*;
public class JXLExcelTable {
public static void main(String[] args) throws Exception {
WorkbookSettings wbSettings = new WorkbookSettings();
Workbook workbook = Workbook.createWorkbook(new File("sample.xls"), wbSettings);
WritableSheet sheet = workbook.createSheet("Sheet 1", 0);
Label label = new Label(0, 0, "Header");
sheet.addCell(label);
label = new Label(0, 1, "Data");
sheet.addCell(label);
workbook.write();
workbook.close();
}
}
5. Using ExcelBuilder

ExcelBuilder, though not as popular as Apache POI or Aspose.Cells, offers a straightforward API for building Excel files. Here's how to create an Excel table using ExcelBuilder:
- Include the ExcelBuilder library in your project.
- Use the code snippet below to create a basic Excel table:
import com.example.excelbuilder.*;
public class ExcelBuilderExample {
public static void main(String[] args) throws Exception {
Workbook wb = new Workbook();
Worksheet sheet = wb.addSheet("Sample Sheet");
sheet.getCell(0, 0).setCellValue("Header");
sheet.getCell(0, 1).setCellValue("Data");
wb.write(new File("sample.xlsx"));
}
}
💡 Note: Some libraries might require commercial licenses for production use.
In summary, creating Excel tables in Java can be achieved through various libraries, each with its unique strengths. Apache POI is renowned for its robust capabilities and wide adoption. JExcelAPI is ideal for fast read/write operations. Aspose.Cells offers advanced features for more complex Excel interactions. JXL, while outdated in some respects, remains an option for specific legacy needs. ExcelBuilder, although less known, provides a simple API for basic Excel operations. When choosing a library, consider:
- Performance Requirements: Does your project demand speed or extensive Excel features?
- License and Cost: Some libraries are free, while others require a commercial license.
- Community Support: Popular libraries tend to have better community support and documentation.
- Complexity of Operations: How advanced are the Excel manipulations you need?
Finally, consider the scalability and maintainability of your solution over time. Choosing the right tool not only simplifies your development process but also ensures your application’s long-term viability.
What is the difference between XLS and XLSX formats?

+
XLS is the older binary file format used by Excel 97-2003, while XLSX is the newer XML-based file format introduced in Excel 2007. XLSX supports larger files and more features than XLS.
Why would I choose JXL over Apache POI?

+
JXL might be chosen over Apache POI for projects requiring faster read/write operations, especially with older Excel formats (XLS). JXL is also simpler for basic operations but lacks support for some advanced features.
Can these libraries handle Excel formulas?

+
Yes, most libraries like Apache POI, Aspose.Cells, and JXL support reading and writing Excel formulas, though the level of support for complex formulas can vary.