5 Ways to Add New Sheets in Excel with Python

Understanding how to add new sheets to an Excel workbook using Python can greatly enhance your data management and analysis workflows. Python, with libraries like openpyxl and pandas, offers versatile solutions for manipulating Excel files. In this post, we will explore five different methods to add new sheets to Excel workbooks, making your scripting and automation efforts more efficient.
Method 1: Using openpyxl

One of the most straightforward ways to add sheets to an Excel file is by using the openpyxl library. Here’s how you can do it:
- Install openpyxl: Run
pip install openpyxl
if you haven't already. - Open an Existing Workbook or Create a New One:
from openpyxl import Workbook # Load workbook or create a new one workbook = Workbook() # or workbook = openpyxl.load_workbook('path/to/your/file.xlsx')
- Add a New Sheet:
new_sheet = workbook.create_sheet(title="New Sheet")
- Save the Workbook:
workbook.save('path/to/your/file.xlsx')
📝 Note: openpyxl supports various Excel features, but it does not handle charts or images well.
Method 2: Using pandas

pandas is known for its data manipulation capabilities, but it also allows for managing Excel workbooks:
- Install pandas: If not installed, use
pip install pandas
. - Create DataFrames: Assume you have a DataFrame
df
with data to insert.import pandas as pd # Create or load an Excel file df = pd.DataFrame({"A": [1, 2, 3], "B": [4, 5, 6]}) with pd.ExcelWriter('output.xlsx', engine='openpyxl') as writer: writer.book = openpyxl.load_workbook('path/to/your/file.xlsx') # Add a new sheet or write to the active sheet df.to_excel(writer, sheet_name="Sheet Name")
This method is great when you are already working with DataFrames, allowing you to write data directly into Excel sheets.
Method 3: Using xlsxwriter

xlsxwriter is another popular library for creating Excel files:
- Install xlsxwriter:
pip install xlsxwriter
. - Create a New Workbook:
import xlsxwriter workbook = xlsxwriter.Workbook('example.xlsx') worksheet = workbook.add_worksheet(name='Sheet1') workbook.add_worksheet(name='Sheet2') workbook.close()
This approach is excellent for creating new Excel files from scratch.
Method 4: Using win32com in Windows

If you’re working on Windows, you can use the COM interface to interact with Excel:
- Import the necessary libraries:
import win32com.client excel = win32com.client.Dispatch("Excel.Application") excel.Visible = True # Open an existing workbook or create a new one wb = excel.Workbooks.Open('path/to/your/file.xlsx') # or wb = excel.Workbooks.Add() # Add a new sheet ws = wb.Sheets.Add() ws.Name = 'New Sheet' # Save and close wb.SaveAs('path/to/your/newfile.xlsx') wb.Close() excel.Quit()
📝 Note: This method requires Excel to be installed on your machine and is Windows-specific.
Method 5: Combining openpyxl with pandas

By combining the capabilities of pandas for data manipulation with openpyxl for Excel operations, you can achieve a versatile solution:
- Example:
import pandas as pd from openpyxl import load_workbook # Load workbook and data df = pd.DataFrame({"A": [1, 2, 3], "B": [4, 5, 6]}) book = load_workbook('path/to/your/file.xlsx') # Add new sheet and write data writer = pd.ExcelWriter('path/to/your/file.xlsx', engine='openpyxl') writer.book = book df.to_excel(writer, sheet_name='New Sheet') writer.save()
This method is ideal when you need to manipulate both data and Excel sheets dynamically.
Each of these methods provides a unique way to interact with Excel files, catering to different use cases and workflow requirements. Whether you're adding new sheets programmatically, managing data, or working with specific file formats, Python's libraries offer robust solutions.
Wrapping up our exploration, we've seen how Python can be leveraged to automate the task of adding sheets to Excel workbooks. From basic operations with openpyxl, efficient data integration with pandas, creating new files with xlsxwriter, or using native Windows COM for complex Excel interactions, Python's ecosystem supports various scenarios. By mastering these methods, you not only streamline your workflow but also unlock the potential for complex data analysis and reporting, enhancing your productivity and data management skills.
Which Python library is best for working with Excel?

+
It depends on your needs. openpyxl is versatile for general Excel manipulation, pandas is excellent for data-centric tasks, and xlsxwriter is best for creating Excel files from scratch.
Can I add a sheet to an existing Excel file without opening it?

+
Yes, libraries like openpyxl allow you to add sheets to existing files without needing to open them manually.
Is there a way to automate Excel tasks on Mac?

+
Absolutely, libraries like openpyxl and pandas work on both Windows and macOS. However, the win32com method mentioned is Windows-specific.
How can I rename sheets in Excel using Python?
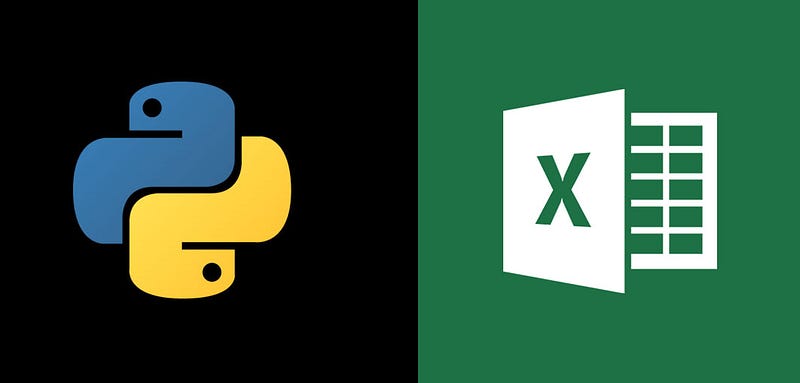
+
With openpyxl, you can use sheet.title = "New Name"
to rename an existing sheet.
What should I do if my Excel file becomes corrupt when I manipulate it with Python?

+
Ensure you’re using the latest version of the library you’re working with. Also, always work with a backup copy of your file to avoid losing data. Sometimes opening and resaving the file manually in Excel can fix minor issues.