5 Simple Steps to Add Sheets in Excel with C

Working with Excel spreadsheets programmatically can streamline repetitive tasks and enhance your ability to analyze and manipulate data efficiently. Microsoft Excel is a powerful tool widely used in various professional and personal scenarios to organize, analyze, and present data. Integrating Excel with a programming language like C# allows you to automate these processes, making data management more dynamic and less error-prone. Here, we'll explore how to add sheets to an Excel workbook using C#, providing you with five straightforward steps to get started.
Step 1: Set Up Your Development Environment

Before you can start manipulating Excel files with C#, you need to set up your development environment:
- Install Visual Studio: Microsoft’s Visual Studio is a comprehensive IDE for .NET development, which is perfect for C# coding.
- Install Excel Interop Assembly: You’ll need Microsoft.Office.Interop.Excel. This can be added to your project through NuGet Package Manager or by manually referencing the DLL.
Step 2: Create a New Excel Application Instance

Once your environment is set up, you can create an instance of the Excel application within your C# code:
var excelApp = new Microsoft.Office.Interop.Excel.Application();
Step 3: Open or Create an Excel Workbook

Next, you’ll either open an existing workbook or create a new one:
- To open an existing workbook: Use the
Workbook.Open
method. - To create a new workbook: Use the
Workbooks.Add()
method.
Workbook workbook;
if (File.Exists("your_workbook_path.xlsx"))
{
workbook = excelApp.Workbooks.Open("your_workbook_path.xlsx");
}
else
{
workbook = excelApp.Workbooks.Add();
}
Step 4: Add New Sheets to the Workbook

With the workbook open, you can now add new sheets:
- Adding a sheet: You can add a sheet by specifying its position or by simply adding it to the end.
Worksheet sheet = workbook.Worksheets.Add(After: workbook.Worksheets[workbook.Worksheets.Count]);
sheet.Name = "New Sheet";
💡 Note: The After parameter allows you to specify where the new sheet will be placed in relation to other sheets.
Step 5: Save and Close the Workbook
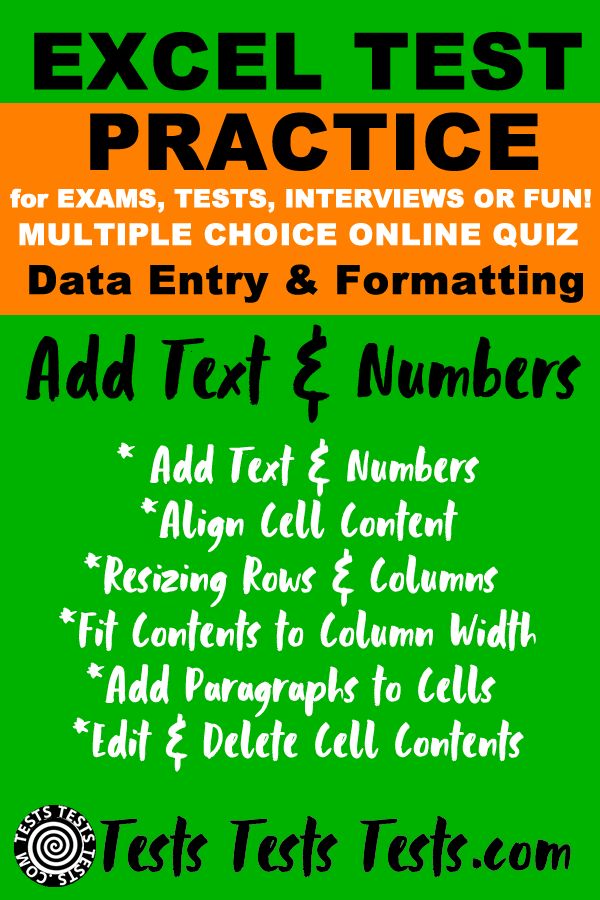
After performing your modifications, ensure to save the workbook:
workbook.SaveAs("your_workbook_path.xlsx");
workbook.Close();
excelApp.Quit();
Make sure to release all COM objects you instantiated to avoid memory leaks:
Marshal.ReleaseComObject(workbook);
Marshal.ReleaseComObject(excelApp);
Conclusion

Integrating Excel manipulation with C# can significantly boost productivity, particularly when dealing with large datasets or repetitive tasks. By following these five simple steps, you can automate the process of adding sheets to an Excel workbook, allowing for more dynamic data handling and reducing manual errors. This approach not only saves time but also allows for advanced manipulation through coding, providing you with greater control and flexibility over your data.
Why should I use C# to automate Excel?

+
Using C# to automate Excel can significantly reduce the time spent on repetitive tasks, improve accuracy by minimizing manual errors, and allow for complex data manipulations that would be time-consuming or impossible with manual Excel usage.
Can I automate other Excel functionalities besides adding sheets?

+
Yes, you can automate many Excel features including formatting cells, creating charts, handling data validations, sorting, and filtering, among others, using C#.
Is it possible to automate Excel without using Visual Studio?

+
While Visual Studio is the preferred IDE for C# development, you can also use other text editors like Visual Studio Code or write scripts with a text editor and compile them with command line tools or other IDEs.