5 Ways to Create Multiple Excel Sheets in Java
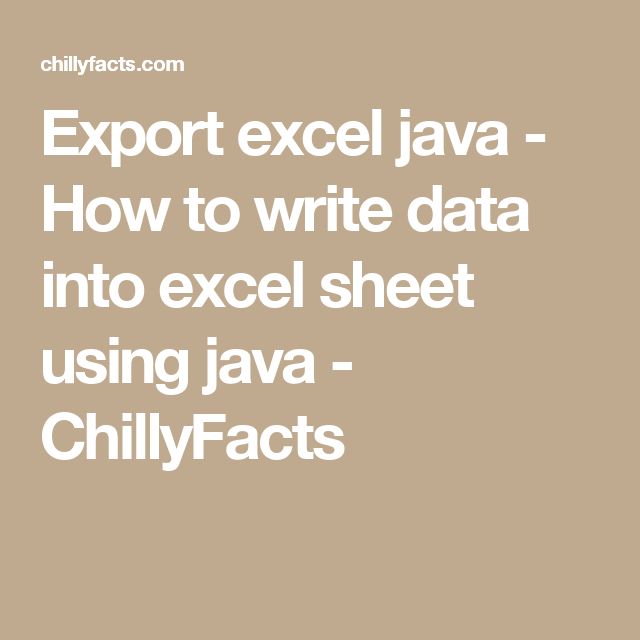
Managing data in Excel spreadsheets is an integral part of many businesses and organizations, providing a convenient way to organize, analyze, and store data. Java, with its robust libraries like Apache POI, makes it relatively straightforward to create and manipulate Excel files programmatically. In this blog, we will explore five different methods to create multiple Excel sheets in Java, each tailored to specific needs and functionalities.
Using Apache POI Library

Apache POI (Poor Obfuscation Implementation) is a powerful library that provides APIs for manipulating various file formats based upon Microsoft's OLE 2 Compound Document format. Here’s how you can use Apache POI to create multiple sheets:
- Download and include the Apache POI jars in your project.
- Use the following steps:
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
Workbook workbook = new XSSFWorkbook();
Sheet sheet1 = workbook.createSheet("FirstSheet");
Sheet sheet2 = workbook.createSheet("SecondSheet");
// Create more sheets as needed
// Write data to sheets
// ...
// Save the workbook
FileOutputStream fileOut = new FileOutputStream("workbook.xlsx");
workbook.write(fileOut);
fileOut.close();
workbook.close();
💡 Note: Apache POI supports both the newer .xlsx file format and the older .xls format, but for better performance and functionality, use .xlsx.
Using JXL (Java Excel API)

The JExcelApi (JXL) library is another option for Excel manipulation in Java, known for its simplicity. Here's how you can use it:
- Add JXL library to your classpath.
- Implement with following code:
import jxl.Workbook;
import jxl.write.Label;
import jxl.write.WritableSheet;
import jxl.write.WritableWorkbook;
import java.io.File;
WritableWorkbook workbook = Workbook.createWorkbook(new File("output.xls"));
WritableSheet sheet1 = workbook.createSheet("FirstSheet", 0);
WritableSheet sheet2 = workbook.createSheet("SecondSheet", 1);
// Add more sheets as necessary
// Write data to sheets
// ...
workbook.write();
workbook.close();
Using JAXB (Java API for XML Binding)

JAXB can be utilized for reading Excel files exported in XML format from Excel. Although this method involves an intermediate XML step, it can be very handy when dealing with XML-structured data:
- Use Excel to export your workbook to XML Spreadsheet 2003 format (.xml).
- Unmarshal XML data into Java objects using JAXB:
import javax.xml.bind.JAXBContext;
import javax.xml.bind.Unmarshaller;
JAXBContext jaxbContext = JAXBContext.newInstance(WorkbookDocument.class);
Unmarshaller unmarshaller = jaxbContext.createUnmarshaller();
WorkbookDocument workbookDoc = (WorkbookDocument) unmarshaller.unmarshal(new File("workbook.xml"));
// Manually create new sheets by modifying the XML structure or using XML manipulation
// ...
// Write back the modified XML structure to an Excel file
💡 Note: This method requires careful handling of XML data, especially for complex Excel functionalities.
Using XLSX Streamer

If dealing with large datasets, XLSX Streamer can be beneficial for its streaming capabilities:
- Add XLSX Streamer to your project dependencies.
- Here's how you might implement it:
import net.arnx.xlsx4j.XSSFWorkbook;
XSSFWorkbook workbook = new XSSFWorkbook();
XSSFSheet sheet1 = workbook.createSheet("Sheet1");
XSSFSheet sheet2 = workbook.createSheet("Sheet2");
// Add more sheets as needed
// Write data to sheets using a streaming method
// ...
// Save the workbook
try (FileOutputStream fileOut = new FileOutputStream("output.xlsx")) {
workbook.write(fileOut);
}
Custom Implementation with POI SXSSF

When performance is critical, especially with large files, using POI SXSSF for streaming can be optimal:
- Include POI and POI-Ooxml in your project.
- Implement like this:
import org.apache.poi.xssf.streaming.SXSSFWorkbook;
SXSSFWorkbook workbook = new SXSSFWorkbook(100); // buffer size of 100 rows per sheet
Sheet sheet1 = workbook.createSheet("Sheet1");
Sheet sheet2 = workbook.createSheet("Sheet2");
// Add more sheets as needed
// Stream data to sheets
// ...
try (FileOutputStream fileOut = new FileOutputStream("output.xlsx")) {
workbook.write(fileOut);
workbook.dispose();
}
In this summary, we’ve covered a wide array of techniques for creating multiple sheets in Excel using Java. Whether you’re looking for simplicity, performance, or specialized XML handling, Java libraries provide solutions to fit various needs. From Apache POI to more niche tools like JAXB or XLSX Streamer, each method offers unique advantages. Choosing the right approach depends on the complexity of your data, the size of your dataset, and how you intend to interact with the spreadsheets.
Understanding these methods can significantly enhance your ability to automate and streamline data management tasks, making your Java applications more versatile and efficient in handling Excel data.
What is the easiest way to create multiple Excel sheets in Java?

+
The easiest way is often using Apache POI or JXL libraries, as they provide straightforward methods to create and manipulate sheets directly from Java code.
Can I manipulate Excel files without creating a new file?

+
Yes, libraries like Apache POI allow you to read an existing Excel file, modify its contents or structure, and then save it back without creating a new file.
How does JAXB work with Excel?

+
JAXB works indirectly by manipulating XML files that have been exported from Excel. It provides a way to work with XML data, which can then be transformed back into Excel format.
What are the benefits of using SXSSF in Apache POI?

+
SXSSF allows for streaming of data, reducing memory usage when dealing with large datasets, as it writes data directly to disk rather than holding all data in memory.