5 Ways to Create an Excel Sheet with VBA
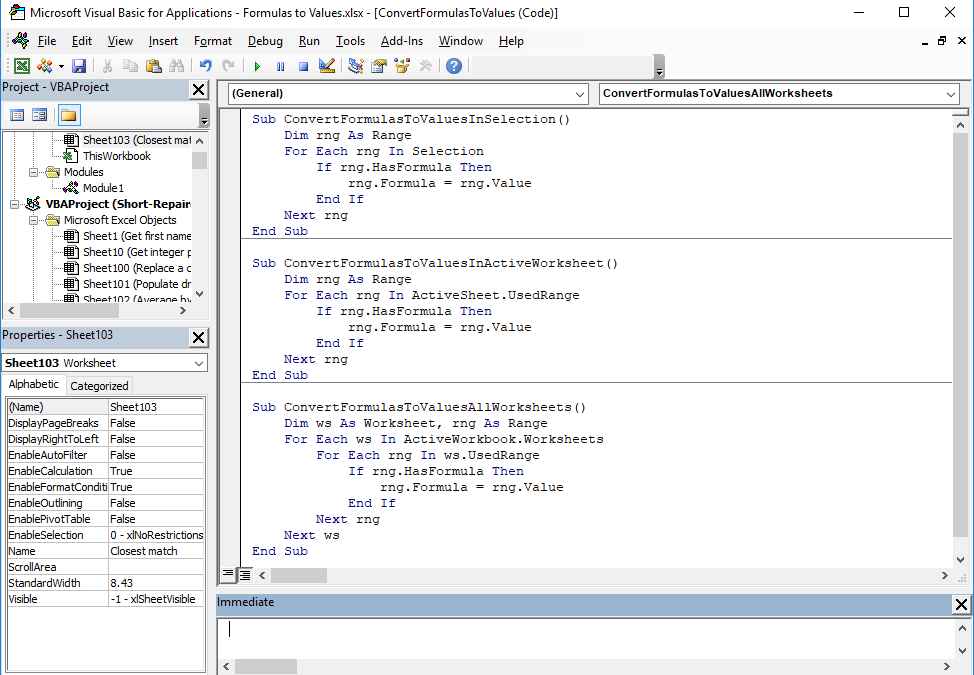
Visual Basic for Applications (VBA) is a powerful tool integrated into Microsoft Office applications like Excel, allowing users to automate tasks, perform complex calculations, and manage large datasets with ease. Whether you're automating repetitive tasks or looking to build sophisticated data analysis tools, here are five distinct methods to create Excel sheets using VBA. Each method provides a different approach suited to various scenarios and levels of VBA expertise.
1. Using the Worksheet.Add Method

The simplest way to add a new worksheet in VBA is through the Worksheet.Add
method. This method is straightforward and ideal for beginners or for quick additions without complex setup:
- Open your Excel file where you want to add a sheet.
- Press Alt + F11 to open the VBA editor.
- Insert a new module by right-clicking on any of the objects in the Project Explorer, choosing 'Insert' then 'Module'.
- Use the following code snippet to add a sheet:
Sub AddNewSheet()
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets.Add(After:=ThisWorkbook.Worksheets(ThisWorkbook.Worksheets.Count))
ws.Name = "SheetName"
End Sub
This VBA code creates a new sheet after the last existing sheet and names it "SheetName".
🛠️ Note: If you want to insert the sheet before the first one or in another position, adjust the After
or use Before
parameter accordingly.
2. Creating Sheets Based on Data
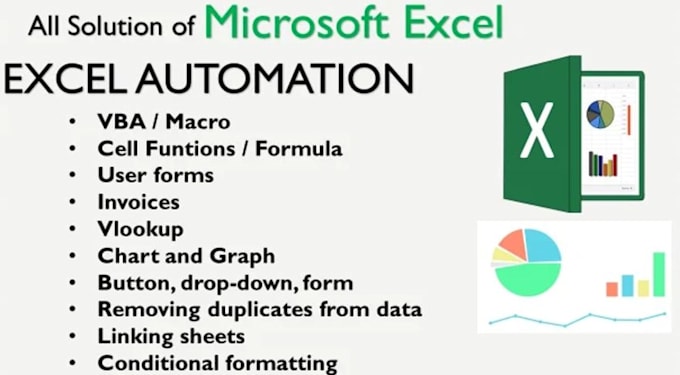
If your task involves splitting data into multiple sheets based on certain criteria, this approach will be handy:
- Suppose you have a dataset in "Sheet1" where you want to split rows into separate sheets based on a column value (e.g., department).
- Here's an example VBA code to achieve this:
Sub SplitDataIntoSheets()
Dim ws As Worksheet
Dim uniqueValues, val
Dim lastRow As Long, i As Long, j As Long
Set ws = ThisWorkbook.Sheets("Sheet1")
lastRow = ws.Cells(ws.Rows.Count, "A").End(xlUp).Row
uniqueValues = WorksheetFunction.Unique(ws.Range("A2:A" & lastRow))
For Each val In uniqueValues
With ThisWorkbook
Set newSheet = .Worksheets.Add(After:=.Sheets(.Sheets.Count))
newSheet.Name = val
End With
j = 2 ' Start from row 2 in the new sheet to copy headers from original sheet
For i = 2 To lastRow
If ws.Cells(i, "A").Value = val Then
ws.Rows(i).Copy Destination:=newSheet.Rows(j)
j = j + 1
End If
Next i
Next val
End Sub
3. Automating with a Template Sheet

If you need to create multiple sheets with a predefined structure or layout, you can utilize a template sheet:
- First, create a template sheet with the necessary layout.
- Then use VBA to copy this sheet multiple times:
Sub CreateMultipleSheetsFromTemplate()
Dim templateSheet As Worksheet
Dim newSheet As Worksheet
Dim i As Integer
Set templateSheet = ThisWorkbook.Sheets("Template")
For i = 1 To 5 'Creating 5 new sheets from the template
templateSheet.Copy After:=ThisWorkbook.Sheets(ThisWorkbook.Sheets.Count)
Set newSheet = ThisWorkbook.Sheets(ThisWorkbook.Sheets.Count)
newSheet.Name = "Sheet" & i
Next i
End Sub
📝 Note: Ensure the template sheet name is correct and is not being used elsewhere in your workbook before copying.
4. Conditional Sheet Creation

Sometimes, you might need to create sheets conditionally based on data analysis:
- Assume your data includes various status codes, and you want to generate summary sheets only for "Active" projects:
Sub ConditionalSheetCreation()
Dim ws As Worksheet
Dim statusRange As Range, cell As Range
Set ws = ThisWorkbook.Sheets("Data")
Set statusRange = ws.Range("B2:B" & ws.Cells(ws.Rows.Count, "B").End(xlUp).Row)
For Each cell In statusRange
If cell.Value = "Active" Then
ThisWorkbook.Worksheets.Add(After:=ThisWorkbook.Sheets(ThisWorkbook.Sheets.Count)).Name = "Project_" & cell.Offset(0, -1).Value
End If
Next cell
End Sub
5. Creating Sheets with User Interaction

For a more interactive approach, allow users to input data or decide on sheet creation:
- This method can use input boxes or forms for user interaction:
Sub UserDrivenSheetCreation()
Dim newSheetName As String
Dim response As VbMsgBoxResult
response = MsgBox("Do you want to create a new sheet?", vbYesNo + vbQuestion, "New Sheet")
If response = vbYes Then
newSheetName = InputBox("Please enter the name for the new sheet:", "Sheet Name")
If newSheetName <> "" Then
ThisWorkbook.Worksheets.Add(After:=ThisWorkbook.Sheets(ThisWorkbook.Sheets.Count)).Name = newSheetName
Else
MsgBox "Sheet creation cancelled as no name was provided.", vbInformation, "Info"
End If
Else
MsgBox "Sheet creation process aborted.", vbInformation, "Info"
End If
End Sub
When you create sheets in Excel using VBA, you're not only automating tasks but also enhancing the functionality of your spreadsheets. Each method above serves different needs:
- The
Worksheet.Add
method for basic operations. - Splitting data for organizing large datasets.
- Template sheets for uniformity and efficiency.
- Conditional sheets for targeted data management.
- User interaction for dynamic workbook development.
Remember, VBA's capabilities in Excel are vast. As you get more comfortable with these methods, consider integrating them with other Excel features or VBA functions to create more complex systems. Whether for personal use or within a business context, mastering these techniques can significantly boost productivity and data management efficiency.
What are some common errors when creating sheets with VBA?

+
Common errors include naming conflicts, where a sheet name already exists, memory limit issues when creating too many sheets, and runtime errors if the code tries to manipulate sheets that do not exist or when using incorrect references.
Can I use VBA to delete or move sheets?

+
Yes, VBA can be used to delete, move, rename, or hide sheets. For example, ThisWorkbook.Sheets(“Sheet1”).Delete
or ThisWorkbook.Sheets(“Sheet1”).Move Before:=ThisWorkbook.Sheets(“Sheet2”)
.
How can I protect the sheets I create with VBA?
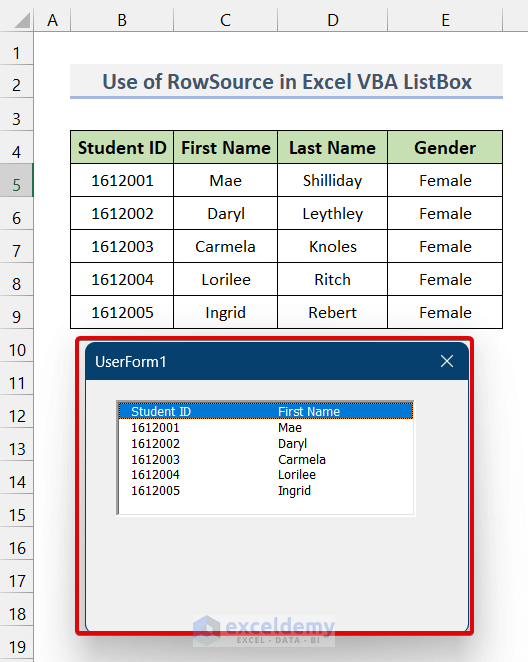
+
You can apply protection using code like newSheet.Protect Password:=“YourPassword”, DrawingObjects:=True, Contents:=True, Scenarios:=True
to lock down the sheet you just created.