5 Ways to Create Excel Sheets in Java Selenium
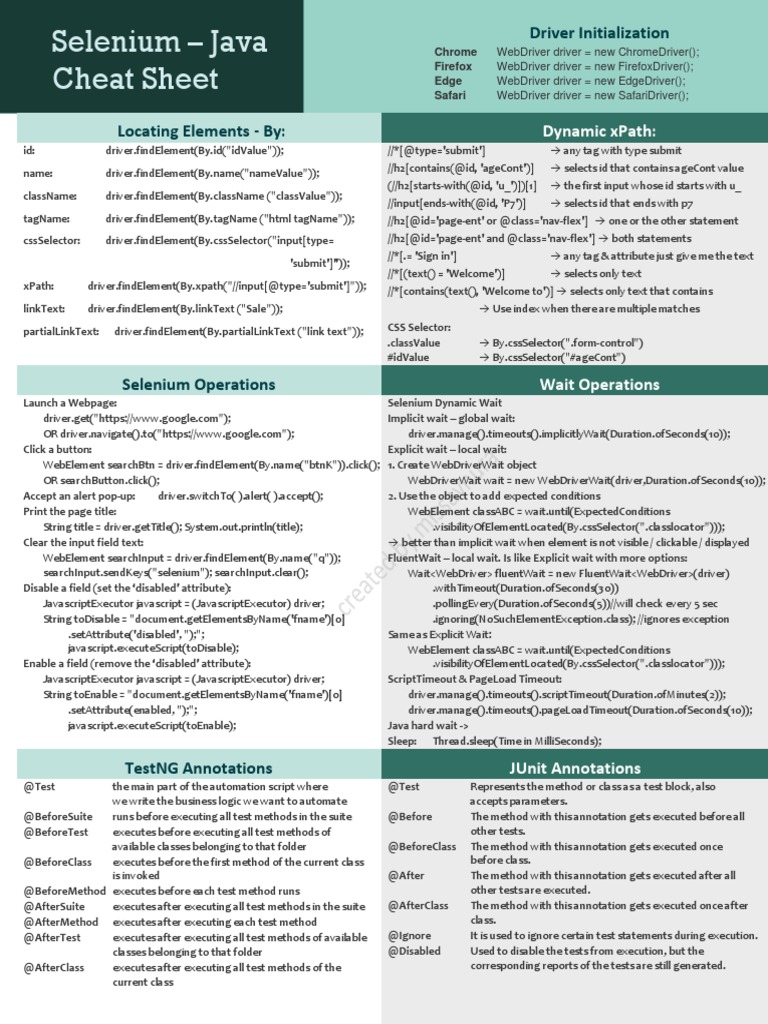
Automating Excel spreadsheets is a powerful feature for web developers who need to manage large datasets or generate reports. Java Selenium, known for its robust web browser automation capabilities, can be extended to manipulate Excel files. This guide will explore five distinct methods to create and manipulate Excel sheets with Java Selenium, making your web scraping or data management tasks more efficient.
Method 1: Using Apache POI

Apache POI is one of the most common libraries used for Excel file manipulation in Java.
- Add Apache POI dependencies to your project.
- Write code to create a workbook, add sheets, and write data.
To start, youโll need to add the following dependencies:
org.apache.poi
poi
5.2.0
org.apache.poi
poi-ooxml
5.2.0
Here's an example of how to create an Excel file:
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public void createExcelUsingPOI() throws IOException {
Workbook workbook = new XSSFWorkbook();
Sheet sheet = workbook.createSheet("Sheet1");
Row headerRow = sheet.createRow(0);
headerRow.createCell(0).setCellValue("Header 1");
headerRow.createCell(1).setCellValue("Header 2");
Row dataRow = sheet.createRow(1);
dataRow.createCell(0).setCellValue("Data 1");
dataRow.createCell(1).setCellValue("Data 2");
// Write the output to a file
FileOutputStream fileOut = new FileOutputStream("workbook.xlsx");
workbook.write(fileOut);
fileOut.close();
workbook.close();
}
Key Features of Apache POI

- Extensive API for cell styling, merging cells, adding formulas, etc.
- Supports both .xls and .xlsx formats.
๐ Note: Be sure to close streams to avoid file corruption.
Method 2: Using JExcelAPI

JExcelAPI, another powerful library, can be used for handling Excel files in Java, particularly for the .xls format.
- Set up JExcelAPI by adding dependencies.
- Create and populate a workbook.
net.sourceforge.jexcelapi
jxl
2.6.12
Here's a simple example:
import jxl.*;
import jxl.write.*;
public void createExcelUsingJExcelAPI() throws Exception {
WritableWorkbook workbook = Workbook.createWorkbook(new File("workbook.xls"));
WritableSheet sheet = workbook.createSheet("Sheet1", 0);
Label label = new Label(0, 0, "Header 1");
sheet.addCell(label);
label = new Label(1, 0, "Header 2");
sheet.addCell(label);
Number number = new Number(0, 1, 123.45);
sheet.addCell(number);
workbook.write();
workbook.close();
}
๐ Note: JExcelAPI does not support the .xlsx format directly; use Apache POI for .xlsx support.
Method 3: Integrating Selenium WebDriver and Apache POI

You can combine Selenium WebDriver to scrape web data and then use Apache POI to store that data in Excel sheets.
- Scrape data using Selenium.
- Save scraped data into Excel.
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public void seleniumDataToExcel() {
System.setProperty("webdriver.chrome.driver", "/path/to/chromedriver");
WebDriver driver = new ChromeDriver();
driver.get("http://example.com");
// Assume we're scraping some data
String data = driver.findElement(By.xpath("//some/xpath")).getText();
// Close browser
driver.quit();
// Now use Apache POI to write this data into an Excel file
XSSFWorkbook workbook = new XSSFWorkbook();
Sheet sheet = workbook.createSheet("Web Scraped Data");
Row row = sheet.createRow(0);
row.createCell(0).setCellValue(data);
// Write to file
FileOutputStream fileOut = new FileOutputStream("scraped_data.xlsx");
workbook.write(fileOut);
fileOut.close();
workbook.close();
}
๐ Note: Ensure your system has appropriate permissions to launch a WebDriver instance and write files.
Method 4: Leveraging Excel Add-ins or COM Automation

For Windows environments, you can automate Excel through COM objects with libraries like Jacob.
- Install Jacob.
- Write code to interact with Excel via COM.
import com.jacob.activeX.ActiveXComponent;
import com.jacob.com.Dispatch;
public void automateExcelWithCOM() {
ActiveXComponent excel = new ActiveXComponent("Excel.Application");
try {
Dispatch excelObject = excel.getObject();
Dispatch.put(excelObject, "Visible", true);
Dispatch workbooks = Dispatch.get(excelObject, "Workbooks").toDispatch();
Dispatch workbook = Dispatch.call(workbooks, "Add").toDispatch();
// Add some data
Dispatch sheet = Dispatch.get(workbook, "Sheets").toDispatch();
Dispatch sheet1 = Dispatch.call(sheet, "Item", new Object[]{1}).toDispatch();
Dispatch cells = Dispatch.get(sheet1, "Cells").toDispatch();
Dispatch.put(Dispatch.invoke(cells, "Item", 1, "SetText", 1, "Header 1").toDispatch(), "Value", "Data 1");
Dispatch.put(Dispatch.invoke(cells, "Item", 1, "SetText", 1, "Header 2").toDispatch(), "Value", "Data 2");
// Save workbook
Dispatch.call(workbook, "SaveAs", "COMAutomatedWorkbook.xlsx");
} finally {
excel.invoke("Quit", new Variant[0]);
}
}
This approach requires Microsoft Excel to be installed and runs only on Windows.
Method 5: Using JDBC-ODBC Bridge

This method leverages database connectivity to interact with Excel files as if they were databases:
- Install and configure the Microsoft Access Database Engine.
- Connect using JDBC-ODBC bridge.
import java.sql.*;
public void createExcelUsingJDBCODBC() throws Exception {
Class.forName("sun.jdbc.odbc.JdbcOdbcDriver");
Connection conn = DriverManager.getConnection("jdbc:odbc:Driver={Microsoft Excel Driver (*.xls, *.xlsx, *.xlsm, *.xlsb)};DBQ=C:\\path\\to\\excel.xlsx;");
Statement stmt = conn.createStatement();
// Create a new sheet by writing SQL commands
stmt.executeUpdate("CREATE TABLE [Sheet1] (Header1 VARCHAR, Header2 VARCHAR)");
stmt.executeUpdate("INSERT INTO [Sheet1] (Header1, Header2) VALUES ('Data 1', 'Data 2')");
stmt.close();
conn.close();
}
๐ Note: Ensure the 32-bit or 64-bit versions of the drivers match your Java runtime environment.
In automating Excel interactions, Java Selenium can be a powerful tool. Here are the key takeaways from our exploration:
- Apache POI is versatile for both .xls and .xlsx formats.
- JExcelAPI is good for older Excel files.
- Selenium can be combined with POI for web scraping and data storage.
- COM Automation is useful but limited to Windows.
- JDBC-ODBC bridge provides database-like manipulation of Excel files.
Each method has its use cases and limitations, so choosing the right one depends on your project's environment, requirements, and the version of Excel files you're working with. Happy automating!
What is the best method for creating Excel files in Java for use with Selenium?

+
Apache POI is widely regarded as the best method due to its robust support for both .xls and .xlsx files and its extensive documentation and community support. Itโs ideal for web scraping projects where data needs to be stored in Excel.
Can I automate Excel without having it installed on the machine?

+
Yes, you can use libraries like Apache POI or JExcelAPI, which do not require Excel to be installed since they work directly with the file format specifications.
How can I add formulas to cells in an Excel sheet using Java?

+
With Apache POI, you can set formulas by using the createFormulaCell
method or directly setting the cellโs formula:
Cell cell = row.createCell(3);
cell.setCellFormula(โA1+B1โ);
What limitations should I be aware of when using JDBC-ODBC for Excel manipulation?

+
The JDBC-ODBC method has several limitations:
- Itโs not as flexible or feature-rich as POI for complex formatting or operations.
- It requires the Microsoft Access Database Engine to be installed.
- There can be issues with data type mismatches, especially with dates and numbers.
Are there any performance considerations when dealing with large Excel files?

+
Large Excel files can significantly slow down operations due to memory usage and the time it takes to read and write data. Streaming methods in Apache POI or batch operations can help manage large datasets more efficiently.
Related Terms:
- Apache POI read Excel
- OOPS concepts in Selenium
- C Selenium interview questions
- Advanced Selenium interview questions