5 Ways to Generate Excel Sheets in C# Web Apps

Generating Excel sheets in C# web applications can significantly enhance the functionality of your software by allowing users to export data in a universally recognized format. This capability is especially valuable for data analysis, reporting, and user-friendly data interaction. In this comprehensive guide, we'll explore five distinct methods to generate Excel sheets in C# web applications, each with its own set of features, pros, and cons.
Using Microsoft.Office.Interop.Excel

The Microsoft.Office.Interop.Excel library allows you to interact with Microsoft Excel directly from your C# code. Here's how you can utilize it to generate Excel sheets:
- Installation: No additional installation is required if you already have Office installed on the server.
- Pros:
- Direct manipulation of Excel files.
- Full support for Excel functionalities like formatting, charts, and macros.
- Cons:
- Requires Office to be installed on the server, which can be resource-intensive.
- Performance issues for high-volume operations due to Excel's COM automation.
Code Example

using Microsoft.Office.Interop.Excel;
using System.Runtime.InteropServices;
// Create Excel application instance
Application excel = new Application();
excel.Visible = false;
Workbook workbook = excel.Workbooks.Add();
Worksheet worksheet = (Worksheet)workbook.Worksheets[1];
// Add data
worksheet.Cells[1, 1] = "Header 1";
worksheet.Cells[1, 2] = "Header 2";
//...
// Save and close
string fileName = Path.GetRandomFileName() + ".xlsx";
workbook.SaveAs(fileName);
workbook.Close();
excel.Quit();
Marshal.ReleaseComObject(excel);
⚠️ Note: Remember to properly dispose of COM objects to prevent memory leaks.
Using EPPlus

EPPlus is an open-source library that allows you to read and write Excel files using .NET Framework or .NET Core, without needing Excel installed on the server.
- Installation: NuGet package 'EPPlus' can be installed easily.
- Pros:
- No dependency on Office installation.
- Great performance for large datasets.
- Cons:
- Some advanced Excel features might be missing.
Code Example

using OfficeOpenXml;
using System.IO;
// Create a workbook and worksheet
var package = new ExcelPackage();
var worksheet = package.Workbook.Worksheets.Add("Sheet1");
// Add data
worksheet.Cells["A1"].Value = "Header 1";
worksheet.Cells["B1"].Value = "Header 2";
//...
// Save the workbook
using (var memoryStream = new MemoryStream())
{
package.SaveAs(memoryStream);
var fileBytes = memoryStream.ToArray();
// Use fileBytes to send Excel file to user
}
Using ClosedXML

ClosedXML provides another approach to generate Excel files, known for its simplicity and ease of use.
- Installation: Also available via NuGet.
- Pros:
- Straightforward API.
- Good performance.
- Cons:
- Limited advanced features compared to EPPlus or Excel itself.
Code Example

using ClosedXML.Excel;
using System.IO;
// Create workbook
var workbook = new XLWorkbook();
var worksheet = workbook.Worksheets.Add("Sheet1");
// Add data
worksheet.Cell(1, 1).Value = "Header 1";
worksheet.Cell(1, 2).Value = "Header 2";
//...
// Save the workbook
using (var memoryStream = new MemoryStream())
{
workbook.SaveAs(memoryStream);
var fileBytes = memoryStream.ToArray();
// Use fileBytes to send Excel file to user
}
Using NPOI

NPOI, known as the .NET version of POI Java project, supports reading and writing of Office documents including Excel files.
- Installation: Can be installed via NuGet.
- Pros:
- Very versatile and widely used.
- Good for both reading and writing Excel files.
- Cons:
- Complex for beginners due to its API structure.
- Performance can be an issue with very large files.
Code Example

using NPOI.SS.UserModel;
using NPOI.XSSF.UserModel;
using System.IO;
// Create workbook and worksheet
IWorkbook workbook = new XSSFWorkbook();
ISheet sheet = workbook.CreateSheet("Sheet1");
// Add data
IRow headerRow = sheet.CreateRow(0);
headerRow.CreateCell(0).SetCellValue("Header 1");
headerRow.CreateCell(1).SetCellValue("Header 2");
//...
// Save to memory stream
using (var memoryStream = new MemoryStream())
{
workbook.Write(memoryStream);
var fileBytes = memoryStream.ToArray();
// Use fileBytes to send Excel file to user
}
Using GemBox.Spreadsheet

GemBox.Spreadsheet is a paid library, but it provides a full-featured Excel solution with a simple API.
- Installation: Available via NuGet or directly from their website.
- Pros:
- Complete Excel support.
- Fast and straightforward implementation.
- Cons:
- Licensing costs for commercial applications.
Code Example

using GemBox.Spreadsheet;
using System.IO;
// Initialize
SpreadsheetInfo.SetLicense("FREE-LIMITED-KEY");
// Create workbook
var workbook = new ExcelFile();
var worksheet = workbook.Worksheets.Add("Sheet1");
// Add data
worksheet.Cells[0, 0].Value = "Header 1";
worksheet.Cells[0, 1].Value = "Header 2";
//...
// Save
using (var memoryStream = new MemoryStream())
{
workbook.SaveXlsx(memoryStream);
var fileBytes = memoryStream.ToArray();
// Use fileBytes to send Excel file to user
}
In wrapping up this journey through the different methods of generating Excel sheets in C#, we've explored five unique approaches. Each has its strengths tailored to different needs:
- Microsoft.Office.Interop.Excel is powerful but resource-heavy, ideal for environments with full Excel capabilities.
- EPPlus offers a high-performance solution for file generation without Excel.
- ClosedXML simplifies the process with an easy-to-use API.
- NPOI provides versatility and compatibility with Java applications.
- GemBox.Spreadsheet gives a complete feature set for those willing to pay for commercial licensing.
Choosing the right tool depends on your application's requirements, server environment, budget, and the level of Excel functionality needed. By implementing one of these methods, your C# web app can provide users with a seamless way to export data into Excel spreadsheets, enhancing both usability and data management capabilities.
Which method is best for generating Excel files on a shared hosting server?

+
EPPlus or ClosedXML would be more suitable as they don’t require Excel to be installed on the server.
Can I use these libraries for both .NET Framework and .NET Core?

+
Yes, EPPlus, ClosedXML, NPOI, and GemBox.Spreadsheet have versions that support both .NET Framework and .NET Core.
What are some common issues when using Microsoft.Office.Interop.Excel?
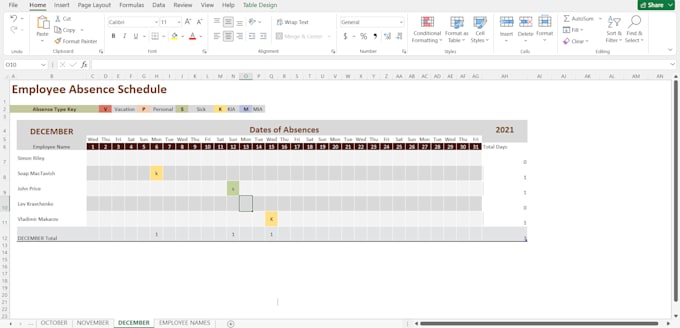
+
Common issues include memory leaks, performance bottlenecks due to COM interop, and the need to handle processes properly to avoid leaving Excel instances running.
How can I handle large datasets with these libraries?

+
For large datasets, use streaming options if available or batch the data into smaller chunks when writing to Excel to manage memory usage effectively.