Convert Excel to XML in C: Easy Guide

Many developers and data analysts need to convert Excel spreadsheets into XML format for integration with various software systems, API interactions, or for further processing. In this guide, we'll explore how to achieve this conversion using C, providing an easy-to-follow, step-by-step approach that doesn't require extensive programming experience. Whether you're working on a project that demands seamless data transfer or just want to enhance your skills, this tutorial on converting Excel to XML in C is just for you.
Preparation

Before diving into coding, there are some preparations you need to make:
- Ensure you have Visual Studio installed for compiling your C programs.
- Install libxlsxwriter for handling Excel files, which you can get through your package manager or directly from its GitHub repository.
- Acquire expat for XML parsing and writing capabilities.
- Download a sample Excel file for testing purposes.
⚠️ Note: Ensure all libraries are compatible with your C compiler version.
Setting Up Your Development Environment

Here are the steps to set up your development environment for this tutorial:
- Install Visual Studio: If you’re using Windows, Visual Studio is ideal. For Linux, consider using a similar IDE or setting up a build environment.
- Library Setup:
- Download or clone the libxlsxwriter from its GitHub repository.
- Compile and install libxlsxwriter. On Linux, you might do:
sudo make install
- Similarly, for expat, use your package manager to install it:
- On Ubuntu/Debian:
sudo apt-get install libexpat1-dev
- On Fedora/CentOS:
sudo yum install expat-devel
Writing the Conversion Code

Now, let’s jump into the actual conversion process with C:
Reading Excel File

#include
#include #include #include #include int main() { lxw_workbook *workbook = workbook_new(“example.xlsx”); lxw_worksheet *worksheet = workbook_add_worksheet(workbook, NULL); worksheet_write_string(worksheet, 0, 0, “Hello”, NULL); workbook_close(workbook);
return 0;
}
The above code creates an Excel file. Here’s how you read from one:
int main() { lxw_workbook *workbook = workbook_new(NULL); workbook_xlsx_read(wb, “example.xlsx”, options); lxw_worksheet *worksheet = workbook_get_worksheet_by_name(workbook, “Sheet1”);
// Process the worksheet here... return 0;
}
Converting Data to XML

To convert your Excel data to XML, you’ll need to:
- Iterate through each cell in your Excel file.
- Extract the data.
- Write this data into an XML structure using libxml2 or expat.
XML_Parser parser = XML_ParserCreate(NULL); XML_SetElementHandler(parser, startElement, endElement); XML_SetCharacterDataHandler(parser, charData);
// For every cell in your worksheet, call these XML functions…
Error Handling and Optimization

While converting files, consider:
- Checking for file existence and permissions.
- Handling errors from both Excel and XML libraries.
- Optimizing the process to minimize memory usage and speed up execution.
📍 Note: Remember to free all allocated memory to prevent leaks.
Final Remarks

In this tutorial, we’ve covered the process of converting an Excel file to XML using C. This can be an invaluable skill when dealing with data-intensive projects or when integrating with systems that require XML input. Keep in mind the importance of proper library setup, error handling, and optimization for your particular use case. This guide should serve as a starting point, and with practice, you’ll be able to customize and expand on these methods to suit more complex scenarios.
Can I convert any Excel file to XML using C?
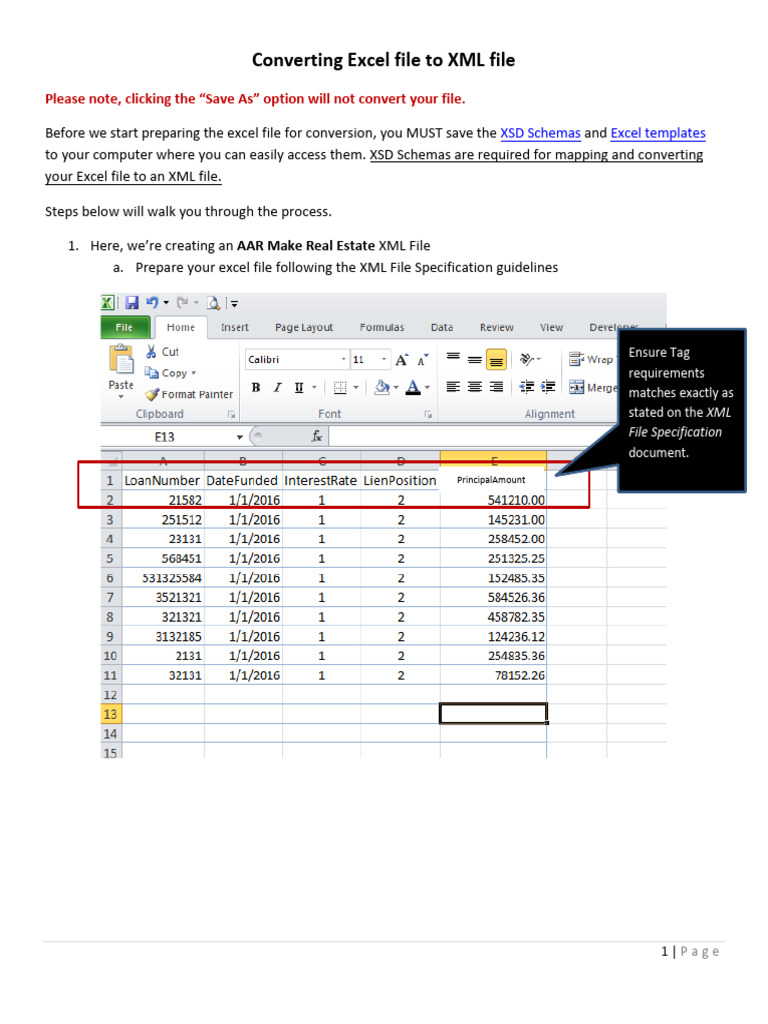
+
Yes, as long as the Excel file is not password-protected or using features not supported by the library you’re using.
What are the limitations of this approach?

+
The process can be complex due to the intricacies of file formats and library limitations. Also, complex Excel features might not convert properly to XML.
Are there any other libraries I can use for Excel to XML conversion in C?

+
Yes, alternatives include libxl, xlsxio, or even open-source projects like phpspreadsheet with C bindings. Each has its strengths and weaknesses.