Convert DataTable to Excel in VB.NET Instantly

Working with data in applications often necessitates exporting data from a DataTable to various formats for analysis, reporting, or archival purposes. Among the popular formats, Microsoft Excel is widely used due to its versatility and powerful data manipulation features. If you are developing an application using Visual Basic .NET (VB.NET), here's a comprehensive guide on how to instantly convert a DataTable to an Excel file without requiring manual entry or complex coding:
Prerequisites for Converting DataTable to Excel
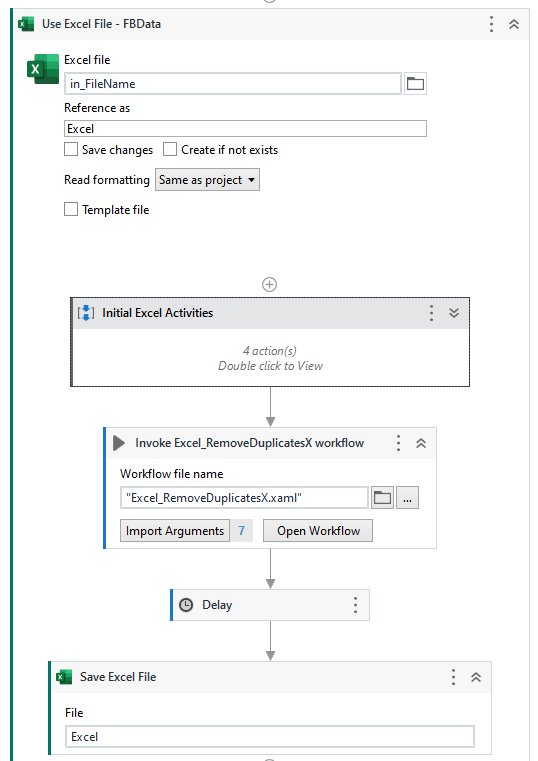
Before we dive into the steps, ensure your development environment is set up with:
- Visual Studio IDE with VB.NET setup
- .NET Framework 4.0 or later installed
- Reference to the necessary libraries (EPPlus, OpenXML SDK, or other third-party tools)
Using a Third-Party Library: EPPlus

EPPlus is a powerful tool for manipulating Office Open XML spreadsheets. Here’s how you can integrate and use EPPlus in your VB.NET application:
Step 1: Install EPPlus

You can add EPPlus to your project through NuGet Package Manager:
- Right-click on your project in Visual Studio.
- Select ‘Manage NuGet Packages…’
- Search for ‘EPPlus’, select it, and install the package.
Step 2: Importing the Necessary Namespaces
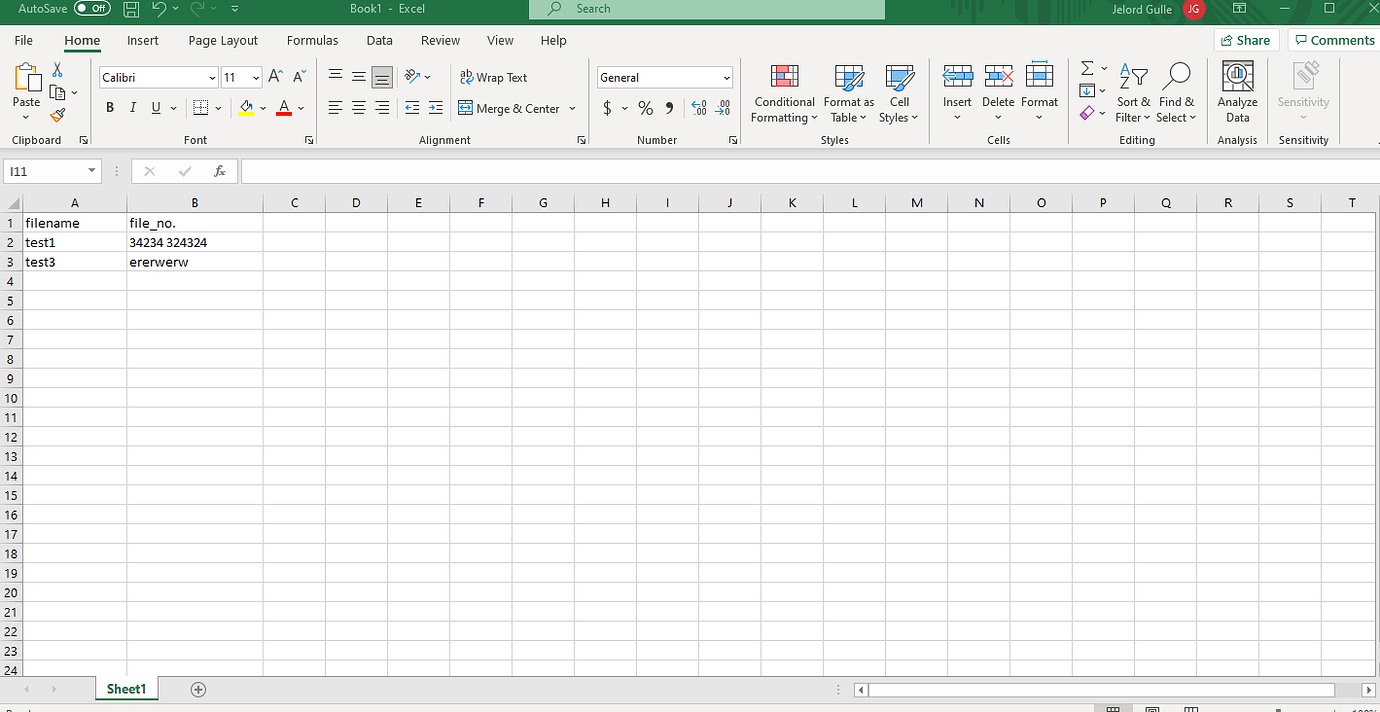
Add these namespaces at the top of your VB.NET file:
Imports OfficeOpenXml
Imports System.IO
Step 3: Creating the Excel File

Now, let’s write the code to convert a DataTable to an Excel file:
Public Sub ExportDataTableToExcel(ByRef dt As DataTable, ByVal fileName As String) Using pck = New ExcelPackage() Dim ws = pck.Workbook.Worksheets.Add(“DataTable Export”)
' Setting header row For i = 0 To dt.Columns.Count - 1 ws.Cells(1, i + 1).Value = dt.Columns(i).ColumnName ws.Cells(1, i + 1).Style.Font.Bold = True Next ' Populating data from DataTable ws.Cells("A2").LoadFromDataTable(dt, False) ' Auto-fit columns ws.Cells.AutoFitColumns() ' Save the Excel file Dim file = New FileInfo(fileName) If file.Exists Then file.Delete() End If pck.SaveAs(file) End Using
End Sub
Call this method by passing your DataTable and the desired file path:
Dim dt As New DataTable() ‘ Populate your DataTable here
ExportDataTableToExcel(dt, “C:\Export\data.xlsx”)
📌 Note: The above method will overwrite any existing file with the same name without prompting the user.
Using OpenXML SDK
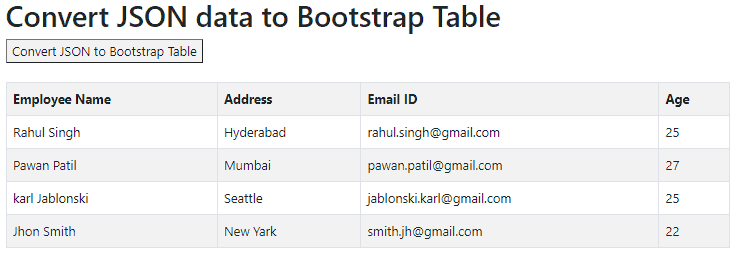
Alternatively, you can use the Microsoft Open XML SDK directly, which gives you finer control over Excel files:
Step 1: Install Open XML SDK

- Download the Open XML SDK from Microsoft’s website.
- Install and add a reference to your project.
Step 2: Importing Namespaces

Imports DocumentFormat.OpenXml.Packaging
Imports DocumentFormat.OpenXml.Spreadsheet
Imports DocumentFormat.OpenXml
Imports System.Data
Step 3: Writing the Conversion Code

Public Sub ExportDataTableToExcel(ByRef dt As DataTable, ByVal filePath As String) Dim document As SpreadsheetDocument = SpreadsheetDocument.Create(filePath, SpreadsheetDocumentType.Workbook) Dim workbookPart = document.AddWorkbookPart() workbookPart.Workbook = New Workbook()
Dim worksheetPart = workbookPart.AddNewPart(Of WorksheetPart)() worksheetPart.Worksheet = New Worksheet() Dim sheets = workbookPart.Workbook.AppendChild(Of Sheets)(New Sheets()) ' Create a row for headers Dim headerRow As New Row() For Each column As DataColumn In dt.Columns Dim cell As New Cell() cell.CellValue = New CellValue(column.ColumnName) cell.DataType = CellValues.String headerRow.Append(cell) Next worksheetPart.Worksheet.Append(headerRow) ' Add data rows For Each row As DataRow In dt.Rows Dim newRow As New Row() For Each column As DataColumn In dt.Columns Dim cell As New Cell() If row(column.ColumnName) IsNot DBNull.Value Then cell.CellValue = New CellValue(row(column.ColumnName).ToString()) cell.DataType = CellValues.String End If newRow.Append(cell) Next worksheetPart.Worksheet.Append(newRow) Next workbookPart.Workbook.Save() document.Close()
End Sub
This method creates a new Excel document, adds a worksheet, and populates it with the DataTable's data. It's more complex than using EPPlus but offers full control over the spreadsheet structure.
Choosing Between EPPlus and OpenXML SDK

Both methods have their merits:
- EPPlus: Simpler to implement, provides a higher level of abstraction, making Excel manipulation straightforward.
- OpenXML SDK: Gives you more control but requires a deeper understanding of the XML structure of Excel files.
Summary of Converting DataTable to Excel
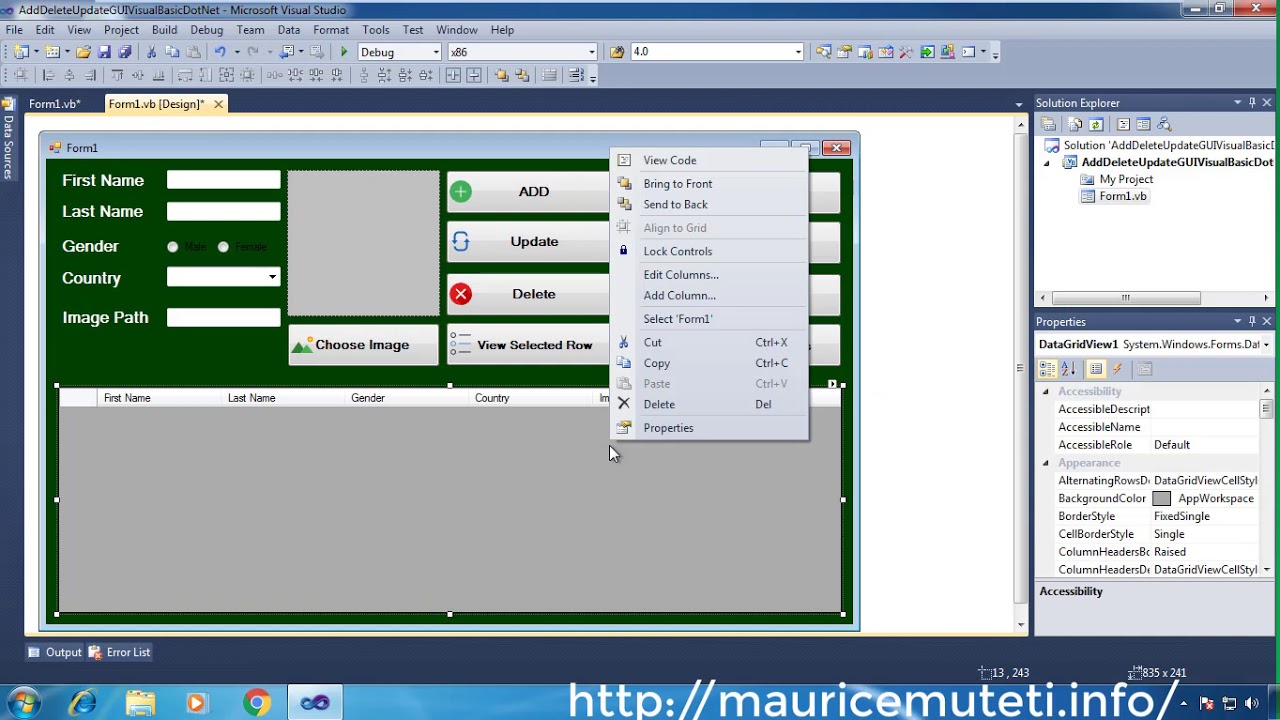
Converting a DataTable to Excel in VB.NET can streamline the process of data handling within your application. Whether you choose EPPlus for its ease or OpenXML SDK for its control, the key steps involve:
- Setting up your development environment with the required tools and references.
- Writing code to export the DataTable to Excel using either library.
- Handling file creation or overwriting carefully to avoid data loss.
By implementing this functionality, you're providing an invaluable feature to your users, allowing them to analyze or report data directly from your application with minimal effort. This not only enhances user experience but also increases the efficiency of data manipulation workflows.
Can I use OpenXML SDK without having Excel installed on the system?
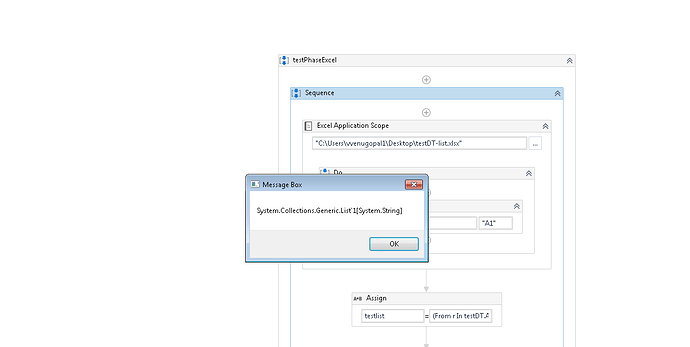
+
Yes, OpenXML SDK is designed to work without needing Excel installed as it directly manipulates the XML format of Excel files.
What are the limitations of using EPPlus to convert DataTable to Excel?
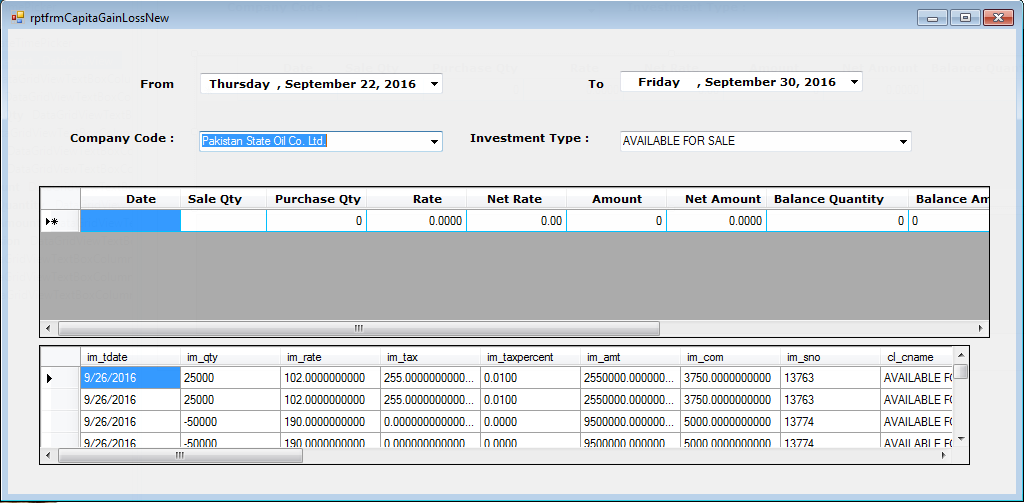
+
EPPlus does not support all Excel functionalities, especially when dealing with complex formulas, macro-enabled workbooks, or some chart types.
How can I format cells when using EPPlus?

+
EPPlus provides methods like .Style.Font.Bold for fonts, .Style.Numberformat for number formatting, and various style properties to customize cells.