5 Ways to Compare Excel Sheets in Python
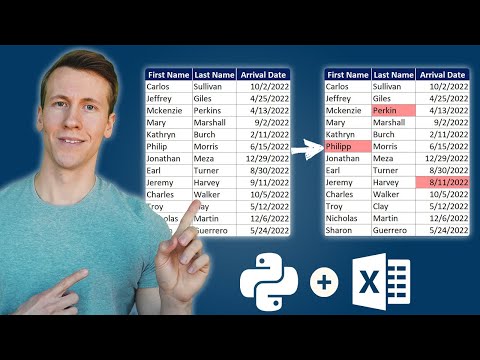
Microsoft Excel is an incredibly powerful tool for data analysis, but when you need to compare large datasets or numerous Excel files, manual comparison can be time-consuming and prone to errors. Fortunately, Python, with its rich ecosystem of libraries like pandas, openpyxl, and xlwings, provides versatile solutions for automating this task. Here are five effective ways to compare Excel sheets using Python, each offering different benefits depending on your specific needs.
Method 1: Using Pandas to Compare Sheets

Pandas is the go-to library for data manipulation and analysis in Python. Here’s how you can use it to compare two Excel sheets:
- Read the Excel files into pandas DataFrames.
- Compare these DataFrames for differences in data, structure, or even formatting.
import pandas as pd
# Load the Excel sheets
df1 = pd.read_excel('file1.xlsx', sheet_name='Sheet1')
df2 = pd.read_excel('file2.xlsx', sheet_name='Sheet1')
# Compare DataFrames
df_diff = df1.compare(df2)
print(df_diff)
⚠️ Note: This method assumes the structure of the sheets is similar. If the columns or rows differ significantly, consider pre-processing the data.
Method 2: Openpyxl for Granular Comparison
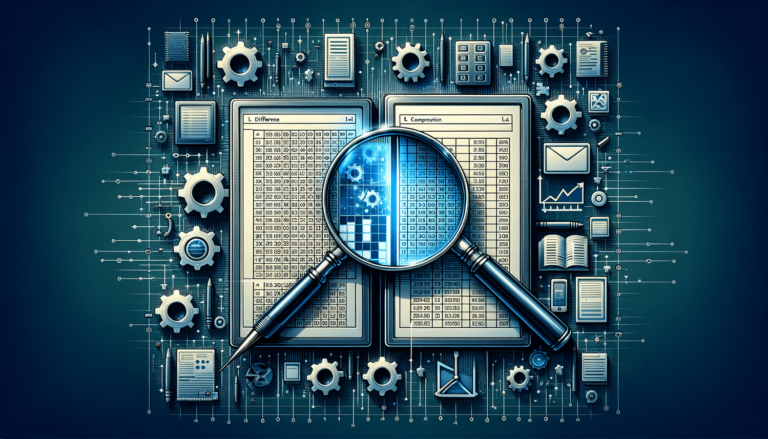
If you’re looking at not just the data but also styles, formats, or comments, openpyxl offers fine-grained control:
from openpyxl import load_workbook
# Load workbooks
wb1 = load_workbook(filename='file1.xlsx')
wb2 = load_workbook(filename='file2.xlsx')
# Access sheets by name
sheet1 = wb1['Sheet1']
sheet2 = wb2['Sheet1']
# Compare values and styles cell by cell
for row in range(1, max(sheet1.max_row, sheet2.max_row)+1):
for col in range(1, max(sheet1.max_column, sheet2.max_column)+1):
cell1 = sheet1.cell(row=row, column=col)
cell2 = sheet2.cell(row=row, column=col)
if cell1.value != cell2.value or cell1.style != cell2.style:
print(f"Diff at Row {row}, Column {col}: Sheet1: {cell1.value} vs Sheet2: {cell2.value}")
⚠️ Note: This method requires considerable memory for large files. For huge datasets, consider processing in chunks or using database tools.
Method 3: Xlwings for a VBA-like Approach
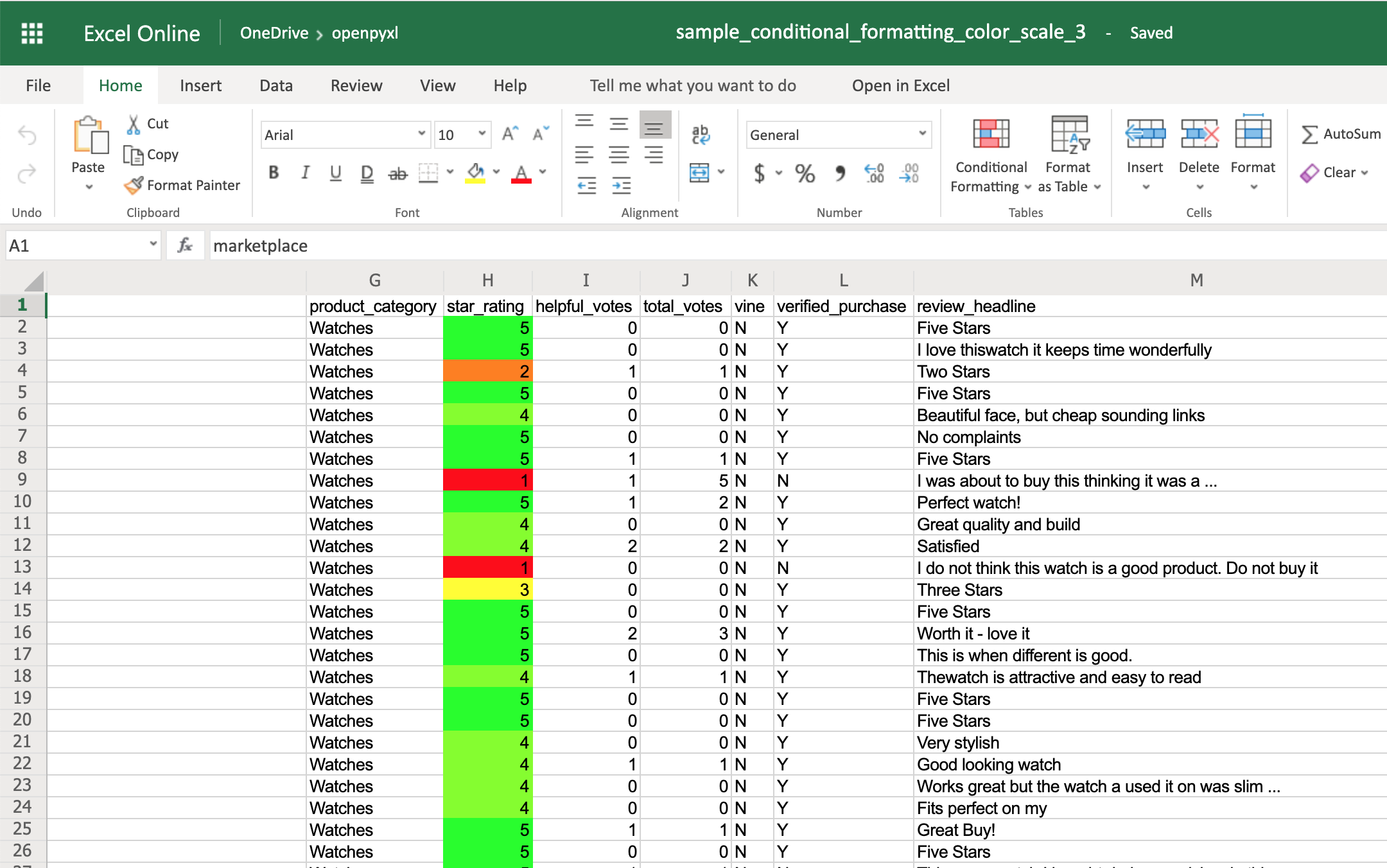
If you’re familiar with VBA and want to leverage Excel’s native features, xlwings can be your best bet:
import xlwings as xw
# Open Excel workbooks
wb1 = xw.Book('file1.xlsx')
wb2 = xw.Book('file2.xlsx')
# Use Excel's built-in functions to compare
sh1 = wb1.sheets['Sheet1']
sh2 = wb2.sheets['Sheet1']
# Example comparison, adjust as necessary
print(sh1.used_range.value == sh2.used_range.value)
wb1.close()
wb2.close()
💡 Note: Xlwings can also run in the background, making it suitable for automation and GUI-less environments.
Method 4: Using Hashlib for File Integrity

If you want to verify if the Excel files themselves are identical, hashing can be efficient:
import hashlib
def get_file_hash(file_path):
h = hashlib.sha256()
with open(file_path, 'rb') as file:
while chunk := file.read(8192):
h.update(chunk)
return h.hexdigest()
file1_hash = get_file_hash('file1.xlsx')
file2_hash = get_file_hash('file2.xlsx')
if file1_hash == file2_hash:
print("Files are identical")
else:
print("Files differ")
This approach can catch any change, including metadata or formatting, not just the visible data.
Method 5: Custom Logic with Third-Party Tools

For more complex comparisons or specific use cases, you might combine Python with third-party tools or custom scripts:
- Integrate Python with Git for version control to track changes in spreadsheets.
- Use difflib in Python for line-by-line comparison after converting spreadsheets to CSV or text.
import csv
from difflib import context_diff
def excel_to_csv(excel_file):
# Convert Excel to CSV, assuming the data starts from A1
df = pd.read_excel(excel_file)
df.to_csv(excel_file.replace('.xlsx', '.csv'), index=False)
return excel_file.replace('.xlsx', '.csv')
def compare_csvs(file1, file2):
with open(file1) as f1, open(file2) as f2:
for line in context_diff(f1.readlines(), f2.readlines()):
print(line, end='')
# Convert Excel files to CSV
file1_csv = excel_to_csv('file1.xlsx')
file2_csv = excel_to_csv('file2.xlsx')
# Compare CSVs
compare_csvs(file1_csv, file2_csv)
Each of these methods has its strengths:
- Pandas is excellent for simple data comparison.
- Openpyxl offers deep insights into Excel features.
- Xlwings is powerful for those familiar with VBA or needing to interact with Excel's native functions.
- File hashing is fast for file-level integrity checks.
- Custom logic allows for tailored comparison strategies.
By leveraging these Python-based approaches, you can streamline the process of comparing Excel sheets, making it quicker, more reliable, and scalable. Whether you're dealing with financial reports, scientific data, or inventory lists, Python provides tools to ensure data consistency and integrity across multiple sheets or files.
Can Python handle large Excel files efficiently?

+
Yes, Python with libraries like pandas or xlwings can handle large files by processing data in chunks or by optimizing memory usage. For very large datasets, consider using database solutions like SQL for comparisons.
What if the Excel sheets have different structures?

+
If the structures differ significantly, you’ll need to preprocess the data to align structures before comparison or use a method like difflib to compare row by row after converting to a text format.
How can I compare cell styles or comments?

+
Use libraries like openpyxl or Xlwings, which provide access to Excel’s cell properties, styles, and comments, allowing for a detailed comparison beyond just data.
Can I automate the process of comparing Excel files?

+
Yes, with Python’s automation capabilities, you can write scripts to automatically open, compare, and even send reports based on differences in Excel sheets.
Is it possible to compare Excel files without opening them?

+
Yes, using the hashing method, you can quickly compare the integrity of the entire file without needing to load or parse its contents.