Compare Excel Sheets with Selenium: A Simple Guide

Why Compare Excel Sheets with Selenium?

Comparative analysis plays a crucial role in data management and decision-making processes. Whether it's for tracking changes in inventory, comparing financial reports over time, or analyzing data from multiple sources, comparing Excel spreadsheets is often necessary. Traditionally, this task is performed manually, which can be time-consuming and error-prone. Here's where Selenium, a powerful tool in web automation, comes into play.
Selenium is typically known for web application testing, but its capabilities extend to automating Excel tasks, especially when paired with other tools like Python, Apache POI, or JXL. In this guide, we'll walk you through the steps to automate Excel sheet comparison using Selenium.
Prerequisites for Using Selenium to Compare Excel Files

- Python: A basic understanding of Python, as it's one of the easiest languages to interface with Excel when using Selenium.
- Selenium: Installed in your Python environment.
- Excel Libraries: Libraries like openpyxl or xlrd to handle Excel files.
- Web Browser Driver: For example, chromedriver for Chrome, geckodriver for Firefox, etc.
- Basic Automation Knowledge: Familiarity with automation concepts.
Setting Up Your Environment

To begin:
- Install Python if you haven't already, and ensure you're using Python 3.6 or later.
- Install the necessary packages:
- Download the appropriate web driver for your browser from their respective websites.
pip install selenium openpyxl
Automating Excel Sheet Comparison with Selenium
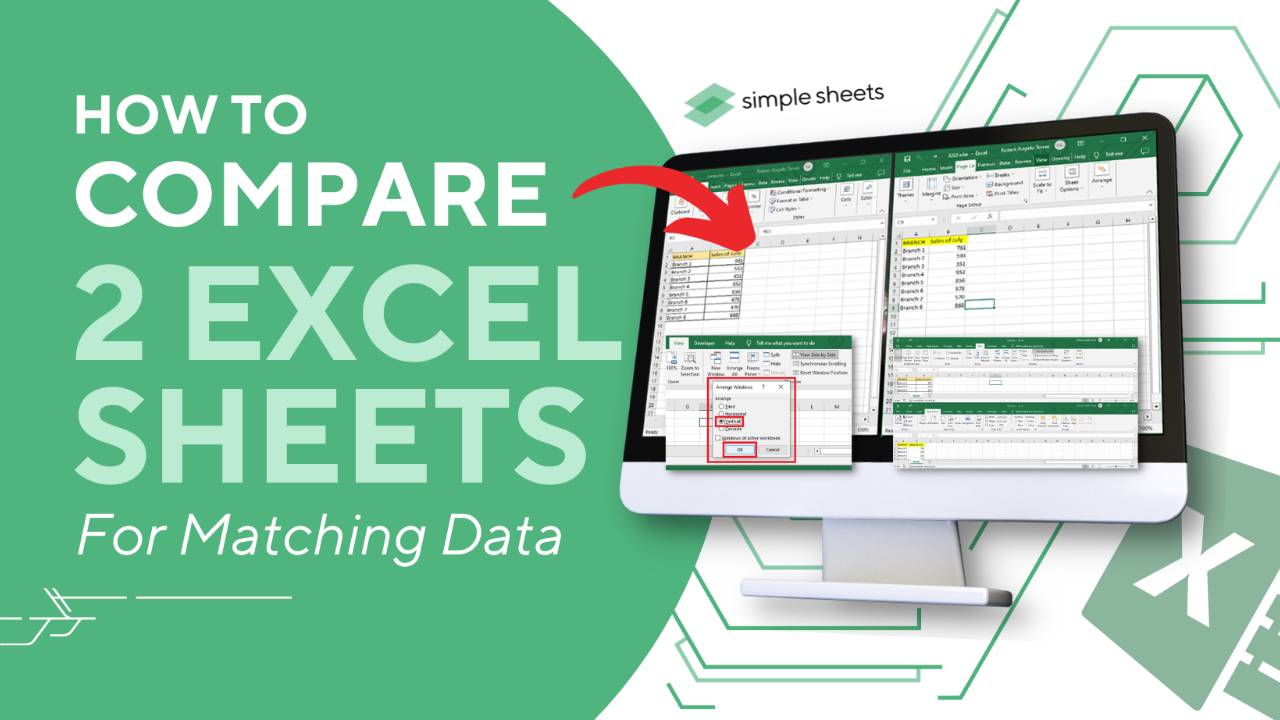
1. Prepare Your Excel Files

Ensure that the Excel files you want to compare are accessible to your Python script. Here are some guidelines:
- Make sure all sheets you wish to compare have similar structures and headers.
- Name your sheets in a way that can be easily tracked or automatically identified if there are many.
- Files should be in a directory accessible to your Python script.
2. Code Structure

Here’s a basic structure for your Python script:
from selenium import webdriver from selenium.webdriver.common.by import By from selenium.webdriver.support.ui import WebDriverWait from selenium.webdriver.support import expected_conditions as EC import openpyxl
driver = webdriver.Chrome(‘path_to_chromedriver’) wait = WebDriverWait(driver, 10)
def compare_excel_files(file1, file2): # Your comparison logic here wb1 = openpyxl.load_workbook(file1) wb2 = openpyxl.load_workbook(file2) sheet_names = wb1.sheetnames
# Assuming you only need to compare the first sheet sheet1 = wb1[sheet_names[0]] sheet2 = wb2[sheet_names[0]] differences = [] for row in range(1, max(sheet1.max_row, sheet2.max_row) + 1): for col in range(1, max(sheet1.max_column, sheet2.max_column) + 1): cell1 = sheet1.cell(row=row, column=col) cell2 = sheet2.cell(row=row, column=col) if cell1.value != cell2.value: differences.append(f'Difference at Cell ({row}, {col}): {cell1.value} vs {cell2.value}') return differences
driver.get(‘your_url’)
file_path1 = ‘path_to_file1.xlsx’ file_path2 = ‘path_to_file2.xlsx’ diffs = compare_excel_files(file_path1, file_path2)
driver.quit()
print(diffs)
🔍 Note: The above script assumes a straightforward comparison where the sheets match in structure. Real-world scenarios might require more complex logic, especially for mismatched sheet names or differing data structures.
3. Extracting Differences

Once you have identified the differences:
- Optionally, write the differences back into a new Excel sheet or log file.
- Or display them in the console or on a web page via Selenium.
Web Interaction for File Upload

Your automation might involve:
- Opening a web page where file upload is possible.
- Selecting the file to upload.
- Confirming the upload and triggering comparison if applicable.
Here's a small example:
# Assuming there's an upload button on the page
file_input = wait.until(EC.presence_of_element_located((By.ID, 'file_upload')))
file_input.send_keys(file_path1)
file_input.send_keys(file_path2)
# Click upload or compare button
driver.find_element_by_id('compare_button').click()
Reporting Differences

Once differences are identified:
- Console Output: For quick verification.
- Web Interface: Show differences on a web page or in a web app.
- Excel Report: Create a new Excel file with comparison results.
Advanced Features

For more advanced scenarios:
- Handling Complex Comparisons: Implement logic for different data structures.
- Dynamic Sheets: Automate selection of sheets to compare if the Excel files have many.
- Web-based Dashboard: Use Selenium to simulate user interactions and upload files to a comparison tool on the web.
Summing up, the integration of Selenium with Excel for data comparison offers a powerful solution for automating repetitive tasks. By setting up your environment correctly, understanding the basic principles of Selenium, and extending its capabilities with Python libraries, you can streamline the comparison of Excel sheets. Whether it's for tracking changes, analyzing data, or ensuring consistency across documents, this guide provides you with the foundational knowledge to start automating your Excel comparisons, thus saving time and reducing human error.
Can Selenium be used with other programming languages for Excel comparison?

+
Yes, while this guide focused on Python, Selenium supports languages like Java, C#, Ruby, JavaScript, and more, allowing you to automate Excel comparisons in various programming environments.
What if my Excel files have different structures?

+
You would need to adjust your comparison logic. Consider implementing logic to handle missing columns, different sheet names, or varying data structures by dynamically aligning data or focusing on specific data points.
Can I automate the entire process?

+
Yes, Selenium can automate not just the comparison but also file upload, report generation, and even email notifications or data visualization on a web interface.