5 Ways to Compare Excel Sheets with Python

The task of comparing Excel sheets can often be tedious and error-prone when done manually, especially when you are dealing with multiple spreadsheets or large datasets. Here, we explore five powerful methods to compare Excel files using Python, a versatile programming language with extensive libraries for data manipulation.
Method 1: Using Openpyxl

Openpyxl is an excellent library for dealing with Excel files in Python. Here’s how you can compare two Excel sheets:
- Install Openpyxl: Ensure you have Openpyxl installed (
pip install openpyxl
). - Load Workbooks: Use Openpyxl to load both Excel workbooks you want to compare.
- Iterate Through Sheets: Go through each sheet in both workbooks, comparing cell values.
- Highlight Differences: Log or highlight differences for easy identification.
📌 Note: This method works best for smaller datasets or when you need fine-grained control over the comparison.
Method 2: Pandas

Pandas is a powerful data manipulation tool in Python which also provides robust functionality to compare Excel files:
- Install Pandas: If not installed, use
pip install pandas
. - Read Excel Files: Use Pandas to read Excel files into DataFrames.
- Compare DataFrames: Utilize Pandas built-in functions like
.compare()
to spot differences. - Display or Export Results: Show differences or save them to another Excel sheet or CSV.
Step | Action |
---|---|
1 | Install Pandas |
2 | Load Excel Files into DataFrames |
3 | Compare DataFrames |
4 | Output Differences |

Method 3: xlrd and xlwt

For those using older Python versions or dealing with .xls files:
- Install xlrd and xlwt: Use
pip install xlrd xlwt
. - Open Files: Open the Excel files using
xlrd
. - Compare: Loop through the rows and columns, comparing cell by cell.
- Save Results: Optionally, write differences to a new file using
xlwt
.
Method 4: Python-diff-match-patch
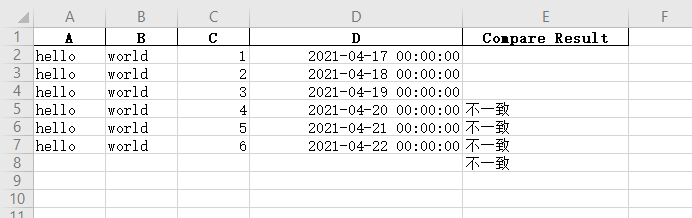
This method is particularly useful for comparing text within cells:
- Install diff-match-patch: Use
pip install diff-match-patch
. - Prepare Data: Convert Excel cell values to text for comparison.
- Compare Text: Use the library to find differences in cell content.
- Report Differences: Output differences in a meaningful format.
Method 5: Custom Script with Multiple Libraries

This approach combines several libraries to achieve a more customized comparison:
- Combine Openpyxl, Pandas, and diff-match-patch: Leverage the strengths of each library.
- Create Comprehensive Comparison: Iterate through sheets, compare data structures, and text within cells.
- Visualize or Export Differences: Present differences in charts, heatmaps, or export to a detailed report.
Each method has its strengths, and the choice depends on your specific needs:
Which method is best for comparing spreadsheets with numerous formulas?

+
Method 2 with Pandas, as it can handle cell values including formulas well.
Can I use Python to compare sheets from different Excel files?

+
Absolutely, all methods listed above support comparing sheets from different workbooks.
What about large Excel files? Will these methods handle them?

+
Pandas and Openpyxl can manage large datasets; however, memory constraints might be an issue with extremely large files.
Is there a method to detect changes made to an Excel sheet over time?

+
Yes, Method 5 or a custom script can help track changes over time by comparing sheets sequentially.
The methods described provide a robust framework for automating the comparison of Excel spreadsheets, saving time, and increasing accuracy. Whether dealing with data validation, financial auditing, or simply tracking changes, Python’s tools and libraries offer flexible solutions to meet various needs. By choosing the appropriate method and tweaking it as required, you can efficiently compare Excel sheets, making data analysis and management much more efficient and reliable.
Finishing Up
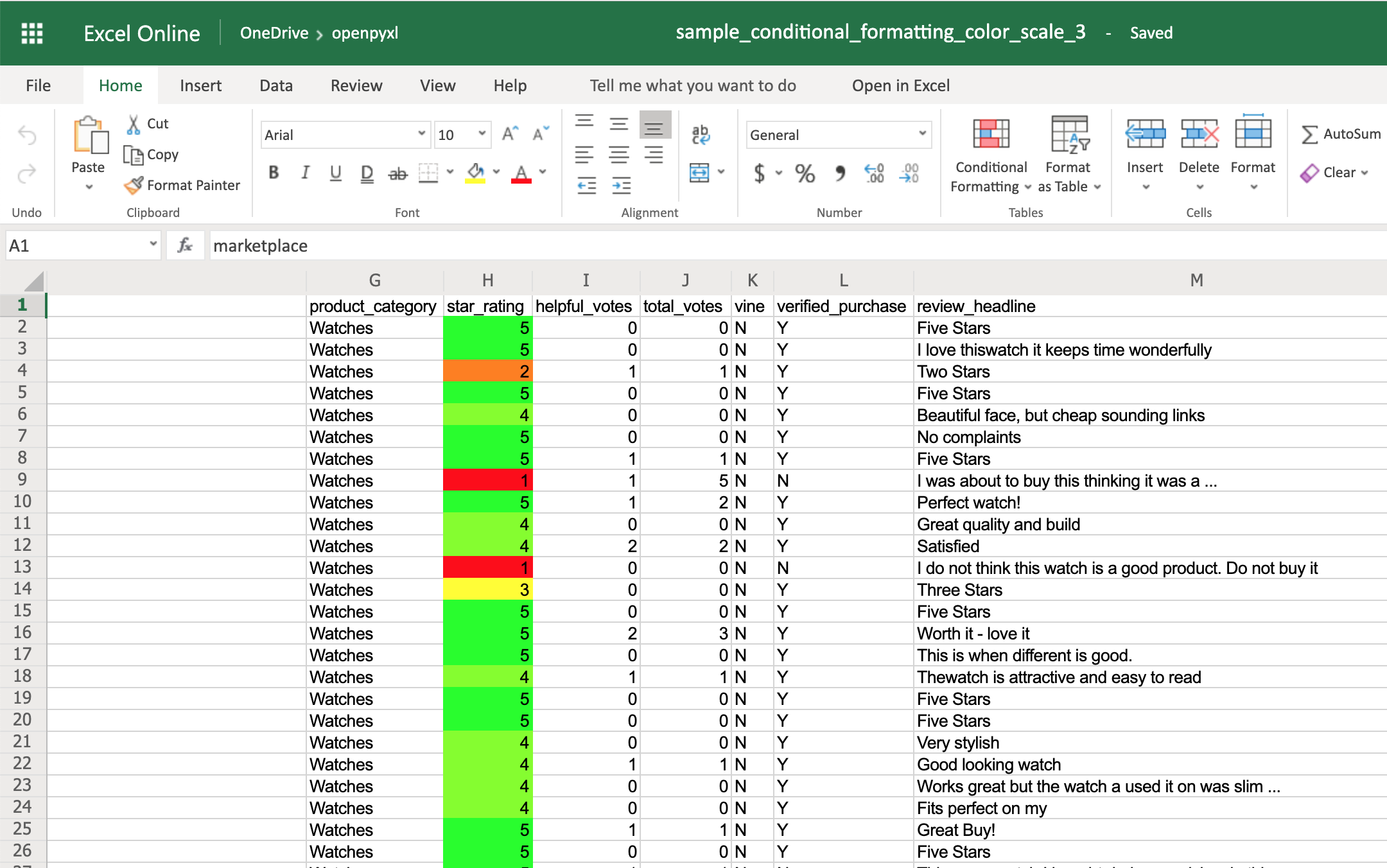
In conclusion, comparing Excel sheets with Python offers a versatile, efficient, and automated approach to data analysis. Here are the key takeaways:
- Each method has its unique strengths, suitable for different types of data and comparison needs.
- Python’s libraries like Openpyxl, Pandas, xlrd/xlwt, and diff-match-patch empower users to handle comparisons with varying complexity.
- Automation with Python not only reduces errors but also provides scalability and customizability in data comparison tasks.
Whether you’re an analyst, auditor, or simply someone who works extensively with spreadsheets, mastering these Python methods can revolutionize your workflow, making data comparison an effortless task. Remember, the right choice depends on your specific requirements, the size of your data, and how you wish to handle and present differences. With these tools at your disposal, you’re well-equipped to compare, track, and analyze Excel data with precision and ease.