5 Essential Tips for Cleaning Excel Sheets in RStudio

Working with Excel data in RStudio can be both a boon and a bane for data analysts. Excel's ubiquity in the business world means we often have to deal with large datasets full of clutter and inconsistencies. However, with a few smart techniques, cleaning your Excel sheets in RStudio can become an efficient part of your data wrangling process. Here are five essential tips to streamline this task.
1. Reading Excel Files with the rightreadxl Package


The first step in any data cleaning process is getting your data into R. The readxl package is perfect for this:
- Install the package if you haven’t already using
install.packages(“readxl”)
. - Use
library(readxl)
to load it. - Import your Excel data with
readxl::read_excel(“path/to/your/file.xlsx”)
.
Here’s an example of how to read an Excel file:
library(readxl) my_data <- read_excel("DataFile.xlsx", sheet = "Sheet1")
💡 Note: If your Excel file contains multiple sheets, specify the sheet name or number to ensure you’re working with the correct data.
2. Cleaning Column Names
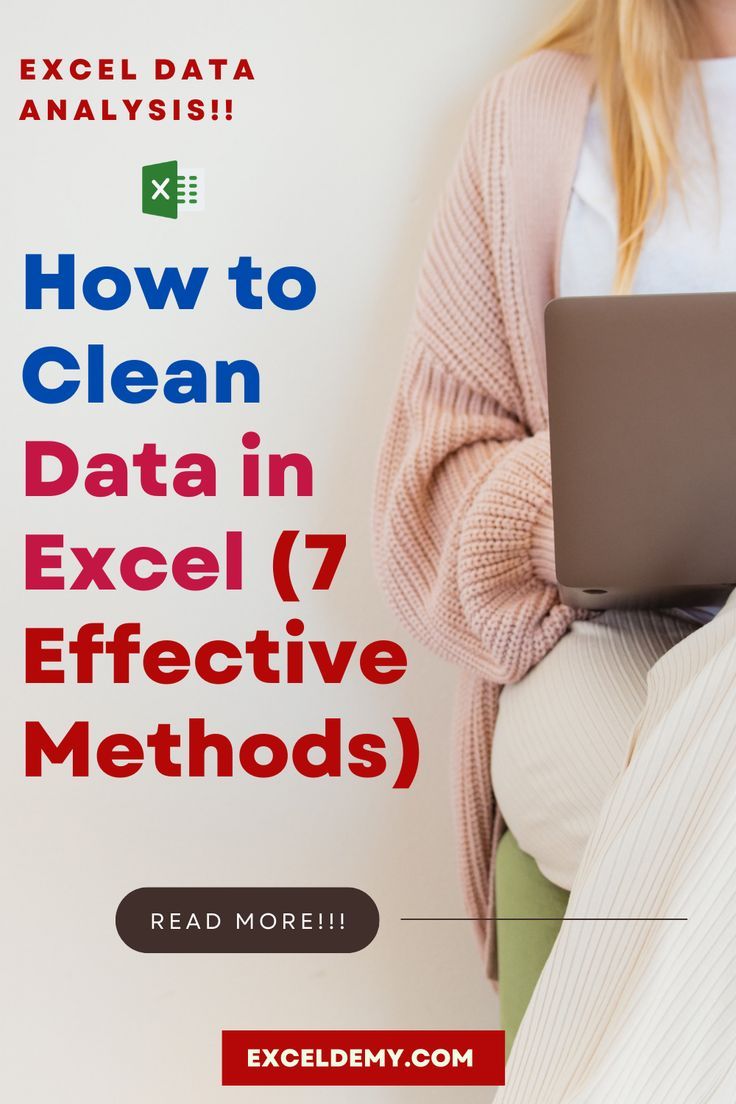

Excel sheets often come with column names that are not ideal for R programming:
- Use
janitor::clean_names()
from the janitor package to convert names to a consistent, snake_case format.
library(janitor) my_data <- clean_names(my_data)
🛠 Note: This function will also deal with special characters, white spaces, and make column names more R-friendly.
3. Handling Missing Values

Missing data is a common issue in Excel files:
- Use
dplyr::na_if
to replace specific values with NA. - You can also use
complete.cases()
orfilter( !is.na())
to remove rows with missing data.
library(dplyr) my_data <- my_data %>% na_if("") %>% na_if("N/A")
4. Data Transformation with Tidyverse


The tidyverse is a collection of R packages designed for data science. Here are some useful functions:
- Filter rows:
filter()
- Select columns:
select()
- Mutate (transform) columns:
mutate()
- Group by and summarize:
group_by()
withsummarize()
library(tidyverse) my_data <- my_data %>% filter(age > 18) %>% select(age, name, department) %>% mutate(new_col = log10(value))
5. Validate and Fix Data Types

Excel often interprets date and time data in formats that might not align with R’s expectations:
- Use
lubridate
package for parsing dates and times. - For numerical data, use
as.numeric()
to ensure numbers are treated as such.
library(lubridate) my_data <- my_data %>% mutate(date = dmy(date)) %>% mutate(numeric_value = as.numeric(value))
The key to efficient data cleaning in RStudio lies in leveraging the right tools and functions. By importing data correctly, cleaning column names, handling missing values, utilizing tidyverse for transformations, and ensuring proper data types, you can transform raw Excel sheets into structured data ready for analysis. Remember, practice makes perfect, and the more you work with these tools, the more proficient you'll become at cleaning your datasets in RStudio.
What is the advantage of using RStudio for Excel data cleaning?

+
RStudio provides powerful data manipulation tools and packages like dplyr, tidyr, and janitor, which can automate many cleaning tasks, making the process faster and more reproducible than manually cleaning in Excel.
Can I handle multiple sheets within one Excel file?

+
Yes, the readxl package allows you to specify sheets by name or number, enabling you to read and manipulate data from multiple sheets within the same file seamlessly.
How do I deal with Excel formatting issues like merged cells?

+
Excel’s formatting like merged cells can be a challenge. Tools like openxlsx or xlsx can sometimes handle this by importing data in a way that accounts for Excel’s formatting, though sometimes manual cleaning might be necessary.